25
AprDifference between Lazy Loading and Eager Loading
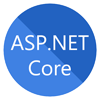
ASP.NET Core Course
In LINQ and Entity Framework, you have Lazy Loading and Eager Loading for loading the related entities of an entity. In this article you will learn the differences between these two loading.
Lazy/Deferred Loading
In case of lazy loading, related objects (child objects) are not loaded automatically with its parent object until they are requested. By default LINQ supports lazy loading.
For Example:
var query = context.Categories.Take(3); // Lazy loading foreach (var Category in query) { Console.WriteLine(Category.Name); foreach (var Product in Category.Products) { Console.WriteLine(Product.ProductID); } }
Generated SQL Query will be:
SELECT TOP (3) [c].[CatID] AS [CatID], [c].[Name] AS [Name], [c].[CreatedDate] AS [CreatedDate] FROM [dbo].[Category] AS [c] GO -- Region Parameters DECLARE @EntityKeyValue1 Int = 1 -- EndRegion SELECT [Extent1].[ProductID] AS [ProductID], [Extent1].[Name] AS [Name], [Extent1].[UnitPrice] AS [UnitPrice], [Extent1].[CatID] AS [CatID], [Extent1].[EntryDate] AS [EntryDate], [Extent1].[ExpiryDate] AS [ExpiryDate] FROM [dbo].[Product] AS [Extent1] WHERE [Extent1].[CatID] = @EntityKeyValue1 GO -- Region Parameters DECLARE @EntityKeyValue1 Int = 2 -- EndRegion SELECT [Extent1].[ProductID] AS [ProductID], [Extent1].[Name] AS [Name], [Extent1].[UnitPrice] AS [UnitPrice], [Extent1].[CatID] AS [CatID], [Extent1].[EntryDate] AS [EntryDate], [Extent1].[ExpiryDate] AS [ExpiryDate] FROM [dbo].[Product] AS [Extent1] WHERE [Extent1].[CatID] = @EntityKeyValue1 GO -- Region Parameters DECLARE @EntityKeyValue1 Int = 3 -- EndRegion SELECT [Extent1].[ProductID] AS [ProductID], [Extent1].[Name] AS [Name], [Extent1].[UnitPrice] AS [UnitPrice], [Extent1].[CatID] AS [CatID], [Extent1].[EntryDate] AS [EntryDate], [Extent1].[ExpiryDate] AS [ExpiryDate] FROM [dbo].[Product] AS [Extent1] WHERE [Extent1].[CatID] = @EntityKeyValue1
In above example, you have 4 SQL queries which means calling the database 4 times, one for the Categories and three times for the Products associated to the Categories. In this way, child entity is populated when it is requested.
You can turn off the lazy loading feature by setting LazyLoadingEnabled property of the ContextOptions on context to false. Now you can fetch the related objects with the parent object in one query itself.
context.ContextOptions.LazyLoadingEnabled = false;
Eager loading
In case of eager loading, related objects (child objects) are loaded automatically with its parent object. To use Eager loading you need to use Include() method.
For Example
var query = context.Categories.Include("Products").Take(3); // Eager loading foreach (var Category in query) { Console.WriteLine(Category.Name); foreach (var Product in Category.Products) { Console.WriteLine(Product.ProductID); } }
Generated SQL Query will be
SELECT [Project1].[CatID] AS [CatID], [Project1].[Name] AS [Name], [Project1].[CreatedDate] AS [CreatedDate], [Project1].[C1] AS [C1], [Project1].[ProductID] AS [ProductID], [Project1].[Name1] AS [Name1], [Project1].[UnitPrice] AS [UnitPrice], [Project1].[CatID1] AS [CatID1], [Project1].[EntryDate] AS [EntryDate], [Project1].[ExpiryDate] AS [ExpiryDate] FROM (SELECT [Limit1].[CatID] AS [CatID], [Limit1].[Name] AS [Name], [Limit1].[CreatedDate] AS [CreatedDate], [Extent2].[ProductID] AS [ProductID], [Extent2].[Name] AS [Name1], [Extent2].[UnitPrice] AS [UnitPrice], [Extent2].[CatID] AS [CatID1], [Extent2].[EntryDate] AS [EntryDate], [Extent2].[ExpiryDate] AS [ExpiryDate], CASE WHEN ([Extent2].[ProductID] IS NULL) THEN CAST(NULL AS int) ELSE 1 END AS [C1] FROM (SELECT TOP (3) [c].[CatID] AS [CatID], [c].[Name] AS [Name], [c].[CreatedDate] AS [CreatedDate] FROM [dbo].[Category] AS [c] ) AS [Limit1] LEFT OUTER JOIN [dbo].[Product] AS [Extent2] ON [Limit1].[CatID] = [Extent2].[CatID]) AS [Project1] ORDER BY [Project1].[CatID] ASC, [Project1].[C1] ASC
In above example, you have only one SQL queries which means calling the database only one time, for the Categories and the Products associated to the Categories. In this way, child entity is populated with parent entity.
What do you think?
I hope you will enjoy the tips while programming with LINQ and Entity Framework. I would like to have feedback from my blog readers. Your valuable feedback, question, or comments about this article are always welcome.