04
JunExploring Node.js Code Execution Process
By default, In Node.js all I/O operations like file I/O, database operations, network operations etc. are non-blocking. When these operations are completed, they are notified to the main thread using callback functions. You can also write blocking code for these operations using blocking version of Node.js functions which have Sync
word as suffix.
Before going to explore Node.js code execution process, let's understand the blocking code and Non-blocking code with the help of example.
Blocking Code Example
The given code is a blocking code, since it will not continue the execution of the next lines of code until the read operation is completed.
var fs = require('fs'); //blocking code without callback function var data = fs.readFileSync('text.txt','utf8'); //wait for reading console.log(data); console.log("Done!"); /* Output * Hello Node.js * Done! */
Read More - Node JS Interview Questions for Freshers
Un-blocking Code Example
The above blocking code can be converted into non-blocking code with the help of callback function. Hence, the given code will continue the execution of the next lines of code without waiting for read operation to be complete.
var fs = require('fs'); //un-blocking code with the help of callback function fs.readFile('text.txt', 'utf8', function (err, data) {//doesn't wait and will read file asynchronously console.log(data); }); console.log("Done!"); /* Output * Done! * Hello Node.js */
The Event Loop
Node.js execution model is based on JavaScript Event loop which is single threaded and based on JavaScript callback mechanism. The event loop facilitates the Node.js to handle concurrent requests. Let’s understand the Node.js execution model with the help of following diagram.
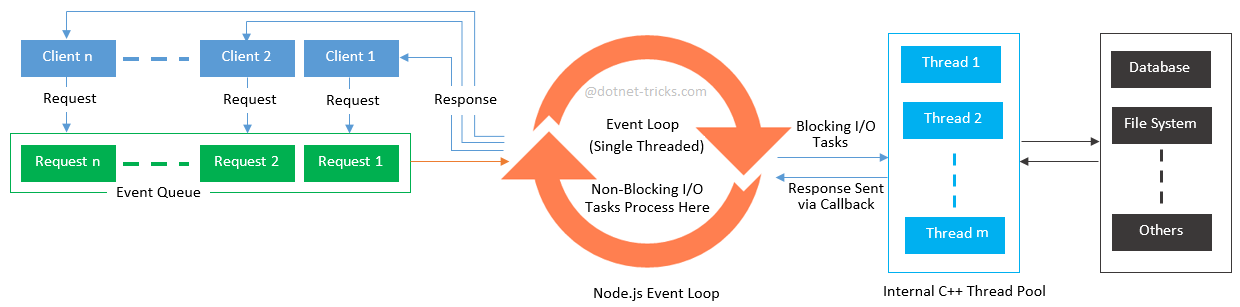
The steps of code execution are given below:
Clients send theirs request to Node.js Server.
Node.js Server receives those requests and places them into a processing Queue that is known as “Event Queue”.
Node.js uses JavaScript Event Loop to process each client request. This Event loop is an indefinite loop to receive requests and process them. Event Loop continuously checks for client’s requests placed in Event Queue. If requests are available, then it process them one by one.
If the client request does not require any blocking I/O operations, then it process everything, prepare the response and send it back to the client.
If the client request requires some blocking I/O operations like interacting with database, file system, external services then it uses C++ thread pool to process these operations.
Thread pool process steps are as:
Event loop checks for thread availability from internal thread pool. If thread is available, then picks up one thread and assign the client request to that thread.
Now, this thread is responsible for handling that request, process it, perform blocking I/O operations, prepare the response and send it back to the Event Loop.
Finally, the Event Loop sends the response to the respective client.
What do you think?
I hope you get the idea how Node.js code execution process work. I would like to have feedback from my blog readers. Your valuable feedback, question, or comments about this article are always welcome.
Take our Nodejs skill challenge to evaluate yourself!

In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.