21
meiUnderstanding Grid View in Asp.Net
GridView control is used to show all table data on the web page. In GridView control each column defines a file or title, and each row depicts a record or data. In short, The GridView control shows data with rows and columns, and you can display the whole table in GridView as well as you can also display only the required columns from a table in GridView control in asp.net.
In this ASP.Net tutorial, We will see a brief introduction to GridView Control which will include what is GridView in Asp.Net, what are the features of GridView control, and Creating a GridView in Asp.Net.
Feature of GridView Control
Creating a GridView in Asp.Net
<asp:GridView ID="gridService" runat="server">
</asp:GridView>
While creating a GridView we will see, how to use a GridView control in ASP.Net using C#.
In this, Let's perform the following different operations on GridView.
- Binding data to the GridView column
- Editing data in GridView
- Updating the GridView
Binding data to the GridView control column
1. Binding data using SQL Connection and SQL DataAdapter
2. Binding Data using DataSet and Table Adapter
3. Binding Data using the LINQ method.
Example:
using System.Data.SqlClient;
using System.Data;
1. Binding data using SQL Connection and SQL DataAdapter
- In this stage write the given code on the ‘ShowData’ (Display Data)button.
- In this method, we used SQLConnection and SQLDataAdapter objects and wrote SQL queries in code behind the page.
- This SQL connection method shows a unique session to an SQL Server data source.
protected void btnview_Click(object sender, EventArgs e)
{
SqlConnection SQLConn = new SqlConnection("Data Source=.\\SQLEXPRESS;Initial Catalog='Scholarhat';Integrated Security=True");
SqlDataAdapter SQLAdapter = new SqlDataAdapter("Select * from UserTable", SQLConn);
DataTable DT = new DataTable();
SQLAdapter.Fill(DT);
GridView1.DataSource = DT;
GridView1.DataBind();
}
2. Binding Data using DataSet and Table Adapter
- Write the given code on the ‘Show Data using SP’ Button(Display Data using SP).
- In this method, we used DataSet and SQL Stored Procedure for SQL connection.
- Also, we used a Table Adapter instead of DataAdapter.
protected void btnviewdataSP_Click(object sender, EventArgs e)
{
DS_USER.USERMST_SELECTDataTable UDT = new DS_USER.USERMST_SELECTDataTable();
DS_USERTableAdapters.USERMST_SELECTTableAdapter UAdapter = new DS_USERTableAdapters.USERMST_SELECTTableAdapter();
UDT = UAdapter.SelectData();
GridView1.DataSource = UDT;
GridView1.DataBind();
}
Binding Data using LINQ method.
- Here, Write the given code on the ‘Show Data using LINQ’ button(Display Data using LINQ).
- We used the LINQ method to fetch data from the SQL server and Display data in GridView control.
protected void btnviewLINQ_Click(object sender, EventArgs e)
{
DataClassesDataContext Ctx = new DataClassesDataContext();
GridView1.DataSource = Ctx.USERMST_SELECT();
GridView1.DataBind();
}
The result of the above GridView example.
Full code Behind this in C# Compiler
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
using System.Data;
public partial class GridviewExample : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnview_Click(object sender, EventArgs e)
{
SqlConnection SQLConn = new SqlConnection("Data Source=.\\SQLEXPRESS;Initial Catalog='Scholarhat';Integrated Security=True");
SqlDataAdapter SQLAdapter = new SqlDataAdapter("Select * from UserMST", SQLConn);
DataTable DT = new DataTable();
SQLAdapter.Fill(DT);
GridView1.DataSource = DT;
GridView1.DataBind();
}
protected void btnviewdataSP_Click(object sender, EventArgs e)
{
DS_USER.USERMST_SELECTDataTable UDT = new DS_USER.USERMST_SELECTDataTable();
DS_USERTableAdapters.USERMST_SELECTTableAdapter UAdapter = new DS_USERTableAdapters.USERMST_SELECTTableAdapter();
UDT = UAdapter.SelectData();
GridView1.DataSource = UDT;
GridView1.DataBind();
}
protected void btnviewLINQ_Click(object sender, EventArgs e)
{
DataClassesDataContext Ctx = new DataClassesDataContext();
GridView1.DataSource = Ctx.USERMST_SELECT();
GridView1.DataBind();
}
}
Editing the GridView
protected void gridService_RowEditing(object sender, GridViewEditEventArgs e)
{
try
{
gridService.EditIndex = e.NewEditIndex;
_BindService();
}
catch (Exception)
{
throw;
}
}
Updating the GridView
protected void gridService_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
try
{
string servicename = ((TextBox)gridService.Rows[e.RowIndex].FindControl("txtService")).Text;
string filePath =((FileUpload)gridService.Rows[e.RowIndex].FindControl("fuService")).FileName;
bOService.Service_name = servicename;
bOService.Service_image = "../images/service/" + filePath;
if (File.Exists(Server.MapPath("~/images/service/" + filePath)))
{
}
else
{
((FileUpload)gridService.FooterRow.FindControl("fuService")).SaveAs(Server.MapPath("~/images/service/" + filePath));
}
dALService.UpdateService(bOService);
_BindService();
}
catch (Exception)
{
throw;
}
}
If you want to perform a Delete operation on GridView Please Check the Below article.
New Article: How to Delete Particular Row From Gridview in ASP.Net
Conclusion
FAQs
Q1. What is GridView control in detail?
Q2. What is the data grid view control?
Q3. Which property is data grid control?
Take our free skill tests to evaluate your skill!
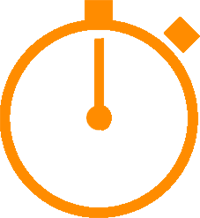
In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.