10
meiInheritance in C++ with Modifiers
Inheritance in C++: An Overview
Inheritance in C++ is taking the attributes and functionality of the desired class without writing the same code in our class. Understanding inheritance
is essential if want to improve your programming abilities in C++. We'll be taking an in-depth look at this particular topic today in this C++ tutorial - so settle down with us as we discuss this important concept. For more check our C++ Certification Training program.
What is Inheritance in C++?
Inheritance is a basic object-oriented programming (OOP) concept in C++ that allows one class to inherit the attributes and functions of another. This means that the derived class can use all of the base class's members as well as add its own. Because it reduces the need to duplicate code for comparable classes, inheritance promotes code reusability and maintainability.
Example of Inheritance in C++
#include <iostream>
class Animal {
public:
void eat() {
std::cout << "Animal is eating." << std::endl;
}
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Dog is barking." << std::endl;
}
};
int main() {
Dog dog;
dog.eat(); // Animal is eating.
dog.bark(); // Dog is barking.
return 0;
}
The Dog class in this example in C++ Compiler derives from the Animal class. This means that the Dog class includes all of the members of the Animal class, as well as any extra members defined by it. The Dog class defines an additional member method called bark() in this situation. The main() function creates a Dog class object and calls its eat() and bark() member functions.
Output
Animal is eating.
Dog is barking.
Read More - C++ Interview Questions and Answers
Why use Inheritance in C++?
- By allowing you to inherit common members from a base class into derived classes, inheritance increases code reusability.
- This eliminates the need for duplicating code and reduces the total coding effort.
- Inheritance aids in the hierarchical organization of code by reflecting the links between various classes.
- This improves the readability and maintainability of the code.
- Inheritance is the foundation for polymorphism, allowing objects of different classes to respond to the same method calls in different ways.
- This increases C++ object-oriented program flexibility and extension.
When to use Inheritance in C++
- When you have a class hierarchy: If you have a group of related classes, and there is a considerable amount of code that is shared by all of them, then inheritance is an acceptable option.
- When you want to construct polymorphic classes: Inheritance is an excellent choice if you want to create classes that can respond to the same message in multiple ways.
- When you need to organize your code: If you wish to organize your code more logically and understandably, inheritance might be a useful concept.
Types of Inheritance Classes
There are two types of Inheritance Classes in C++:
- Base Class
- Derived Class
1. Derived Class
A derived class inherits the base class's properties and methods. The derived class can inherit all of the base class's attributes and methods or only a subset of them. The derived class may additionally include its own set of properties and methods.
2. Base Class
A base class is one that other classes inherit from. The base class defines the attributes and methods that its derived classes inherit. In addition, the base class can specify its properties and methods.
Example of Inheritance Classes
#include <iostream>
class Shape {
public:
virtual void draw() = 0;
};
class Circle : public Shape {
public:
Circle(int radius) : radius(radius) {}
void draw() override {
std::cout << "Drawing a circle with radius " << radius << std::endl;
}
private:
int radius;
};
class Rectangle : public Shape {
public:
Rectangle(int width, int height) : width(width), height(height) {}
void draw() override {
std::cout << "Drawing a rectangle with width " << width << " and height " << height << std::endl;
}
private:
int width;
int height;
};
int main() {
Circle circle(5);
Rectangle rectangle(10, 20);
circle.draw();
rectangle.draw();
return 0;
}
Output
Drawing a circle with radius 5
Drawing a rectangle with width 10 and height 20
Types of Inheritance in C++ with Example
C++ supports the following five types of inheritance:
Single inheritance
Multiple
inheritance
Multilevel inheritance
Hierarchical inheritance
Hybrid inheritance
1. Single Inheritance in C++
When a child/derived class inherits only one base/parent class, it is called a single inheritance
.
Example to demonstrate single inheritance in C++ Editor
#include <iostream>
using namespace std;
class Vehicle
{
public:
int speed;
void StartEngine()
{
cout << "Engine started." << endl;
}
};
class Car : public Vehicle
{
public:
void Accelerate()
{
cout << "Car is accelerating." << endl;
}
};
int main()
{
Car myCar; // object of the Car class
myCar.speed = 60; // member of the base class
myCar.StartEngine(); // member function of the base class
myCar.Accelerate(); // member function of the derived class
return 0;
}
Here, the derived class Car
inherits a single base class i.e. Vehicle
.
Output
Engine started.
Car is accelerating.
Read More - C++ Interview Questions Interview Questions for Freshers
2. Multiple Inheritance in C++
When a child/derived class inherits from more than one base/parent class, it is called multiple inheritance
.
Example to demonstrate multiple inheritance
#include <iostream>
using namespace std;
class Birds {
public:
Birds() {
cout << "Birds are of two types" << endl;
}
};
class Oviparous {
public:
Oviparous() {
cout << "Oviparous birds lay eggs." << endl;
}
};
class Sparrow: public Birds, public Oviparous {
public:
void display() {
cout << "I am a sparrow." << endl;
}
};
int main() {
Sparrow h1;
h1.display();
return 0;
}
Here the class Sparrow
is derived from base classes Birds
and Oviparous
. It makes sense because Sparrow
is a bird as well as oviparous
.
Output
Birds are of two types
Oviparous birds lay eggs.
- Ambiguity in Multiple Inheritance:
Suppose, two base classes have the same function. When you call that function using the object of the derived class, the compiler shows an error. It's because the compiler doesn't know which function to call.
Example
#include <iostream> using namespace std; class base1 { public: void display() { cout << "Class base1" << endl; } }; class base2 { public: void display() { cout << "Class base2" << endl; } }; class derived : public base1, public base2 { void view() { display(); } }; int main() { derived d; d.display(); return 0; }
Here the method
display()
is defined in both base classes,base1
andbase2
. The derived class methodview()
contains the function call todisplay()
method. When the objectd
of the derived class calls thedisplay()
method, the compiler gets confused about whichdisplay()
to call, thebase1
class or thebase2
class. Thus, it throws an error.Output
error: reference to 'display' is ambiguous display();
This ambiguity could be resolved in two ways
- using the scope resolution operator
::
. In the above example, it can be resolved in two waysclass derived : public base1, public base2 { void view() { base1::display(); // Calling the display() function of class base1 base2::display(); // Calling the display() function of class base2 } };
int main() { d.base1::display(); // Function of base1 class is called d.base2::display(); // Function of base2 class is called. }
- The above error would not have occurred if the
display()
method had been defined in the derived class as well. In other words, if thedisplay()
method had been overridden in the derived class. We will understand this in the Polymorphism in C++ and C++ Function Overriding tutorial.
- using the scope resolution operator
3. Multilevel Inheritance in C++
When one base class is inherited by a derived class which is further inherited by any other derived class, it is known as multilevel inheritance. Here, a derived class becomes the base class of another derived class. In the end, the last derived class acquires all the members of all its base classes.
Example to demonstrate multilevel inheritance
#include <iostream>
using namespace std;
class base
{
public:
void display()
{
cout << "Class base1" << endl;
}
};
class derived1 : public base
{
public:
void print()
{
cout << "This is the 1st derived class" << endl;
}
};
class derived2: public derived1
{
void view()
{
cout << "This is the 2nd derived class" << endl;
}
};
int main()
{
derived2 d;
d.display();
d.print();
d.view();
return 0;
}
Here, class derived1
is inheriting the class, base
which is further inherited by the derived2
class. The object d
of the derived2
class accesses all the methods of the base classes, base
and derived1
.
Output
Class base1
This is the 1st derived class
This is the 2nd derived class
4. Hierarchical Inheritance in C++
If more than one derived class inherits a single base class, a hierarchy of base classes is formed and so it is known as hierarchical inheritance.
Example to demonstrate hierarchical inheritance
#include <iostream>
using namespace std;
class Birds {
public:
void type() {
cout << "I'm an oviparous bird" << endl;
}
};
class pigeon: public Birds{
public:
void name1() {
cout << "I am a pigeon." << endl;
}
};
class Sparrow: public Birds {
public:
void name2() {
cout << "I am a sparrow." << endl;
}
};
int main() {
pigeon p; // object of pigeon class
cout << "\npigeon Class:" << endl;
p.type(); // base class method
p.name1();
Sparrow h; // object of Sparrow class
cout << "\nSparrow Class:" << endl;
h.type(); // base class method
h.name2();
return 0;
}
Here, both the pigeon
and Sparrow
classes are derived from the Birds
class. As such, both the derived classes can access the type()
function belonging to the Birds
class.
Output
pigeon Class:
I'm an oviparous bird
I am a pigeon.
Sparrow Class:
I'm an oviparous bird
I am a sparrow.
5. Hybrid Inheritance in C++
It is the combination of more than one type of inheritance i.e hybrid variety of inheritance.
Example to demonstrate hybrid inheritance
#include <iostream>
using namespace std;
class Birds {
public:
Birds() {
cout << "Birds are of two types" << endl;
}
};
class Oviparous : public Birds {
public:
Oviparous() {
cout << "Oviparous birds lay eggs." << endl;
}
};
class Sparrow {
public:
void name2() {
cout << "I am a sparrow." << endl;
}
};
class HybridSparrow : public Oviparous, public Sparrow {
public:
void display() {
name2();
cout << "HybridSparrow class is a combination of Oviparous and Sparrow." << endl;
}
};
int main() {
HybridSparrow h1;
h1.display();
return 0;
}
- In the above code in C++ Online Compiler, class
Oviparous
inherits from classBirds
. This is a single inheritance. - The class
HybridSparrow
inherits from both classOviparous
and classSparrow
. This is multiple inheritance. - The
Oviparous
class inheritsBirds
class which is further inherited by theHybridSparrow
class. This is a multilevel inheritance.
Output
Birds are of two types
Oviparous birds lay eggs.
I am a sparrow.
HybridSparrow class is a combination of Oviparous and Sparrow.
Access Modes of Inheritance
There are three Access Modes of Inheritance in C++:1. Public Mode
When we derive a subclass from a public base class. Then the base class's public members become public in the derived class, and the base class's protected members become protected in the derived class.
2. Protected Mode
When a subclass is derived from a Protected base class. Then, in the derived class, both public and protected members of the base class will be protected.
3. Private Mode
When a subclass is derived from a Private base class. The base class's public and protected members will then become Private in the derived class.
C++ protected Members
- Protected members are a sort of access specifier in C++ that regulates the accessibility of class members.
- Protected members can be accessed from within the class, as well as from any derived classes and friend functions in C++.
- They are, however, inaccessible from outside the class or from any other classes that are not inherited from it.
Here is a table summarising protected members' accessibility:
Access Level | Access |
Inside the class | Yes |
Derived classes | Yes |
Friend functions | Yes |
Outside the class | No |
Other classes | No |
Example of C++ Protected Members
#include <iostream>
class Base {
protected:
int data;
public:
Base(int data) {
this->data = data;
}
int getData() {
return data;
}
};
class Derived : public Base {
public:
Derived(int data) : Base(data) {}
int getDerivedData() {
return data * 2;
}
};
int main() {
Base base(10);
Derived derived(20);
// Output: 10
std::cout << base.getData() << std::endl;
// Output: 20
std::cout << derived.getData() << std::endl;
// Output: 40
std::cout << derived.getDerivedData() << std::endl;
return 0;
}
In this example, the Base class's data member is protected, limiting access to the Base class, its derived classes, and friend methods of the Base class. The data member is unreachable outside of these bounds. The Derived class receives access to the data member by inheriting from the Base class, allowing the getDerivedData() method to access and alter it. This demonstrates how protected members can be accessed in a controlled manner within class hierarchies.
Output
10
20
40
Advantages of Inheritance in C++
- Code Reusability: Inheritance in C++ allows you to create new classes that are based on existing classes, so you can reuse existing code and avoid rewriting the same functionality. This saves a lot of time and effort when you’re creating new programs.
- Organization: Inheritance in C++ helps you organize your code in a logical and structured way. You can create a hierarchy of related classes that makes it easy to understand how your code works and how different parts of your program are related.
- Polymorphism: Inheritance facilitates polymorphism, which allows you to treat objects of derived classes as objects of their base class, enabling you to write code that operates on base-class pointers or references but can work with objects of derived classes at runtime.
- Overriding: Inheritance in C++ allows you to override methods in a derived class, which means you can change the way a method works without changing the original code.
- Abstraction: Inheritance in C++ allows you to create abstract classes that define the basic behavior of a group of related classes while leaving the implementation details to the derived classes.
Summary
Learning about inheritance
C++ programming can help you better understand how objects
are related to one another. This can be a helpful tool when working with large programs. If you want to learn more about inheritance in C++ programming, you can go for C++Certification and you will get a vast idea about inheritance.
Take our free skill tests to evaluate your skill!
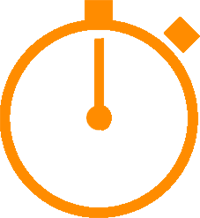
In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.