10
meijQuery Animation
jQuery provides several methods to add some special effect and animation to our web application. In this article we are going to see those jQuery methods which is used to add some special effect to our web page
Read More - jQuery Interview Questions for Experienced
hide ()
It is basically used to hide the matched element in the web page.
HTML<h2> jQuery Animation</h2> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" /> <button id="btnTxtVal">Hide Text Box</button>JavaScript
$('#btnTxtVal').on('click', function () { $("#txtInput").hide(); $("#btnTxtVal").text("Show Text Box") })Browser
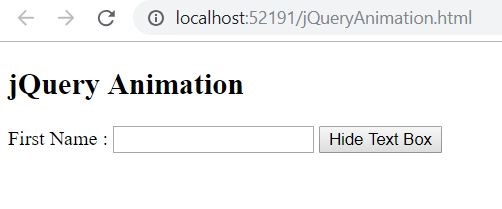
After hitting the Hide Text Box button, the textbox will get hidden and the button caption will be change to Show Text Box
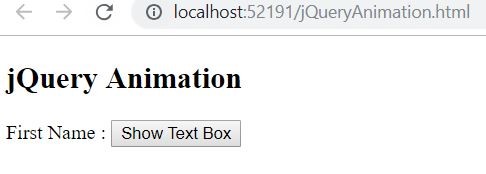
hide () come with different overloads, like
hide (duration, call back)
hide(option)
hide (duration, call back)
Duration is a number which is used to determine how long the animation need to run, default duration is 400ms. call back function which is executed once the animation execution is completed
$('#btnTxtVal').on('click', function () { $("#txtInput").hide(5000, function () { console.log("hide() completed") }); $("#btnTxtVal").text("Show Text Box") })Browser
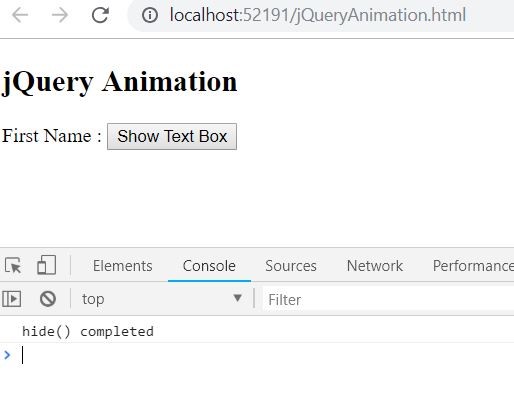
So, the text box hiding is animated for 5sec, Once the animation is completed the call back function is executed.
hide(option)There are many options which can be overloaded with these animation functions in jQueryMost used options are duration, start, done, fail.We already discussed about the duration. Start is a kind of event which is fired when the animation going to start, done event will be executed when the animation gets completed and the fail event will be executed when the animation gets failed in executionNote : All the jQuery animation effects can be off using the statement jQuery.fx.off = true .
show ()
It is used to show the matched element in the web page.
HTML<h2> jQuery Animation</h2> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" style="display:none" /> <button id="btnTxtVal">Show Text Box</button>JavaScript
$('#btnTxtVal').on('click', function () { $("#txtInput").show(); $("#btnTxtVal").text("Hide Text Box") })Browser
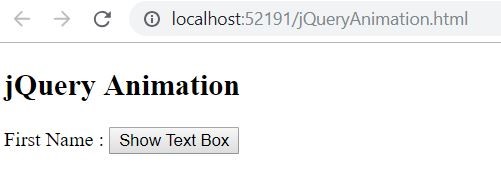
While user hitting the Show Text box button the first name text box will be displayed, behind the scene the style display:none will be removed. As like hide (), show() also come with different overloads, like
show(duration, call back)
show(option)
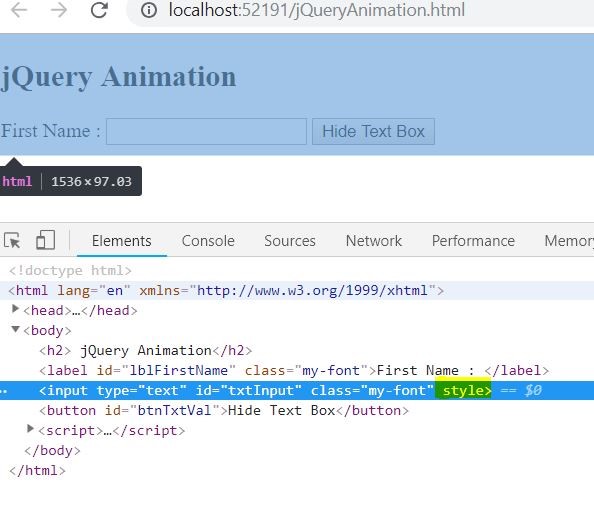
From the above figure you can notice the style “display:none” of the text box will get remove when the show function is execute.
toggle()
It is used to show or hide the matched element
HTML<h2> jQuery Animation</h2> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" style="display:none" /> <button id="btnTxtVal">Toggle Text Box</button>JavaScript
$('#btnTxtVal').on('click', function () { $("#txtInput").toggle(); });Browser
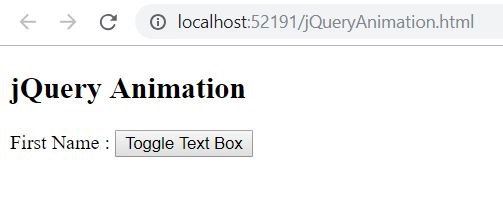
While clicking on toggle text box the text box will get displayed behind the scene the display:none style got removed from the element.
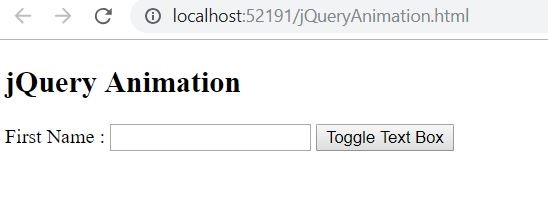
Hitting the toggle text box button again will hide the text box behind the scene it will add CSS property display: none to the style attribute for the element. As like other jQuery effect functions toggle() also comes with different overloads, like
toggle( duration, call back)
toggle(option)
toggle(display)
we already covered first two usage of overload with hide(), now let's see the new display overload to toggle.
toggle(display)display is a Boolean, we can pass true or false, true to display false to hide the element.
HTML<h2> jQuery Animation</h2> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" style="display:none" /> <button id="btnTxtVal">Show Text Box</button>JavaScript
var txtInput = $("#txtInput"); var btnTxtVal = $("#btnTxtVal"); if (txtInput.is(":visible")) { txtInput.toggle(false); btnTxtVal.text("Show Text Box ") } else { txtInput.toggle(true); btnTxtVal.text("Hide Text Box ") }
The above code is used to check whether the textbox is visible or not, if it is visible hide it using toggle(false) or show it using toggle(true)
Browser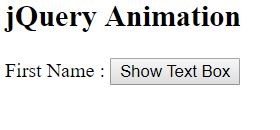
Once the button is clicked the text box will be displayed by executing toggle(true)
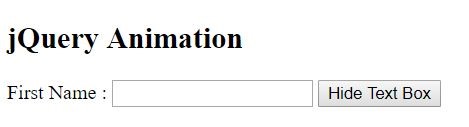
The toggle(false) will be executed once the hide text box is clicked.
slideToggle ()
It is used to toggle the element with some animated effect like sliding motion
HTML<h2> jQuery Animation</h2> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" style="display:none" /> <button id="btnTxtVal">Toggle Text Box</button>JavaScript
$("#txtInput").slideToggle();
As like other jQuery effect functions slideToggle() also comes with different overloads, like
slideToggle (duration, call back)
slideToggle (option)
fadeToggle()
It is used to display or hide the matched element with a fading effect, which means customizing the opacity of the element
JavaScript$("#txtInput").fadeToggle();
As like other jQuery effect functions fadeToggle() also comes with different overloads, like
fadeToggle (duration, call back)
fadeToggle (option)
fadeToggle(duration, callback)
$("#txtInput").fadeToggle(2000,function(){ console.log(“fadeToggle execution completed”); });
The textbox fading will last for 2sec and at the end of execute the call back function will get fired
slideDown()
It is used to display the matched element with sliding motion effect
JavaScript$("#txtInput").slideDown();
As like other jQuery effect functions slideDown() also comes with different overloads, like
slideDown (duration, call back)
slideDown (option)
slideDown(duration, callback)
$("#txtInput").fadeToggle(2000,function(){ console.log(“slideDown execution completed”); });
The first name textbox will slide and displayed to the user within a duration of 2sec and final the callback method will be executed
slideUp()
It is used to hide the matched element with sliding motion effect.
HTML<h2> jQuery Animation</h2> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" /> <button id="btnTxtVal">Toggle Text Box</button>JavaScript
$("#txtInput").slideUp();
As like other jQuery effect functions slideUp() also comes with different overloads, like
slideUp(duration, call back)
slideUp(option)
slideUp(duration, callback)
$("#txtInput").slideUp(2000,function(){ console.log(“slideUp execution completed”); });
The first name textbox will slide and hidden from the user within a duration of 2sec and final the callback method will be executed.
fadeIn()
It is used to display the matched element with fading effect.
HTML<h2> jQuery Animation</h2> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" style="display:none" /> <button id="btnTxtVal">Toggle Text Box</button>JavaScript
$("#txtInput").fadeIn();
when fadeIn() executes, the text box will display with fading effect As like other jQuery effect functions fadeIn() also comes with different overloads, like
fadeIn (duration, call back)
fadeIn (option)
$("#txtInput"). fadeIn(2000,function(){ console.log(“fadeIn execution completed”); });
The above code is used to display the text box with fading effect and duration of 2sec, the call back function will fire once the animation is completed.
fadeOut()
It is used to hide the matched element with fading effect.
HTML<h2> jQuery Animation</h2> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" /> <button id="btnTxtVal">Toggle Text Box</button>JavaScript
$("#txtInput").fadeOut();
when fadeOut() executes, the text box will hide with fading effectAs like other jQuery effect functions fadeOut() also comes with different overloads, like
fadeOut(duration, call back)
fadeOut(option)
$("#txtInput"). fadeOut(2000,function(){ console.log(“fadeOut execution completed”); });
The above code is used to hide the text box with fading effect and duration of 2sec, the call back function will fire once the animation is completed.
fadeTo()
This method gives the flexibility to adjust the opacity of the matched element.
$(selector).fadeTo(duration , opacity , callback)HTML
<h2> jQuery Animation</h2> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" /> <button id="btnTxtVal">Click Me</button>JavaScript
$("#lblFirstName").fadeTo("slow", Math.random(), function () { console.log("fadeIn execution completed") })
From the above code the “slow” is used to set the duration the value range will be 200ms-600ms.
Browser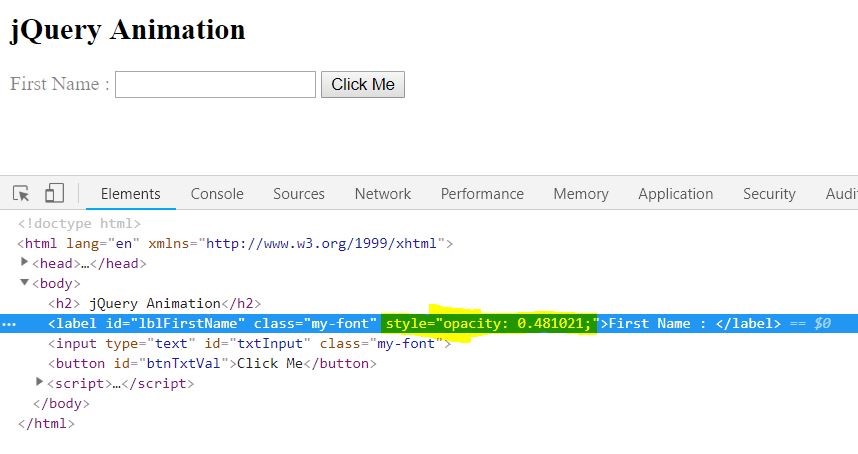
From the above figure you can notice the random opacity is added to the first name label when the button is clicked.
delay()
It is used to create a delay to execute the subsequent item in queue, basically it will set a timer to delay the execution.
$(selector).delay(duration,queueName)HTML
<h2> jQuery Animation</h2> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" /> <button id="btnTxtVal">Click Me</button>JavaScript
$("#txtInput").fadeOut(400).delay(1000).fadeIn(400);
First the fadeout will be executed then the execution of fadeIn will be delayed for 1sec
animate()
It is used to animate the matched element based on the CSS properties
$(selector). animate(properties,duration, callback)
properties - > it is an object of CSS properties.
HTML<h2> jQuery Animation</h2> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" /> <button id="btnTxtVal">Click Me</button>JavaScript
$('#btnTxtVal').on('click', function () { $("#txtInput").animate({ opacity: Math.random(), width: "140px", }, 400, function () { console.log("animate execution completed") }); });Browser
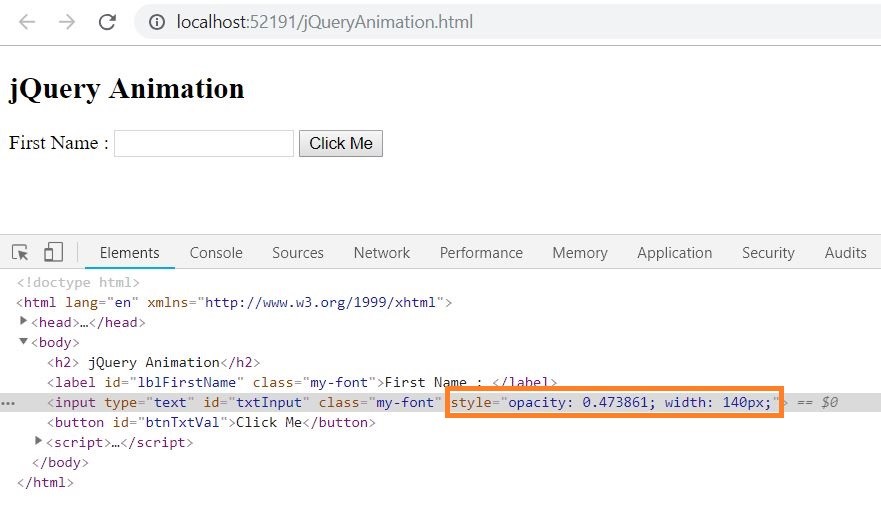
From the above figure you can observe the CSS style is defined for textbox using animate() while clicking on button.
Summary
We have seen how to animate the HTML element using the jQuery animation functions and the flexibility provided by these animate functions. The functions which we discussed in this article is frequently used jQuery methods to animate the web page.
Download the source code https://github.com/gowthamece/jQueryBasics/blob/master/jQueryAnimation.html
Take our free skill tests to evaluate your skill!
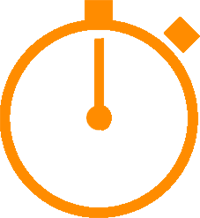
In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.