10
meiTop 50 OOPs Interview Questions and Answers
OOPs Interview Questions and Answers
Object-oriented programming (OOP) is a programming approach that organizes software design around data, rather than functions and logic. It is a fundamental concept of programming.
Major companies seek developers proficient in object-oriented approaches as well as patterns. So, if you are planning to appear for such an interview process, you must have an in-depth understanding of the OOP concepts. No matter whether you give the interview for a C++, Java, or Python developer, OOP questions will come to the surface. Hence, it's compulsory to get yourself prepared for the toughest of the OOP questions.
To help you in your journey, we've collected from various sources some of the commonly asked OOP questions and segregated them under basic, intermediate, and advanced category questions. If you've faced any other questions in the past or come across in the future, do comment and let us know.
OOPs Interview Questions & Answers for Beginners
1. What do you understand by the term OOP?
OOPs is an acronym for Object Oriented Programming. It is a programming paradigm in which everything is represented as an object. OOPs, implement real-world entities in the form of objects. The main aim of OOP is to organize together the data and the functions that operate on them so that no other part of the program can access this data except that function.
2. What is a class in OOP?
Class is a collection of objects. It is like a blueprint for an object. It is the foundational element of object-oriented programming. An instance of a class is created for accessing its data types and member functions.
3. What is an object in OOP?
An object is a real-world entity having a particular behavior and a state. It can be physical or logical. An object is an instance of a class and memory is allocated only when an object of the class is created.
Example illustrating Classes and Objects in Different Programming Languages
class MyClass: # The class
def __init__(self): # Constructor
self.num = 0 # Attribute (int variable)
self.string = "" # Attribute (string variable)
if __name__ == "__main__":
obj = MyClass() # Create an object of MyClass
# Access attributes and set values
obj.num = 25
obj.string = "Welcome to ScholarHat"
# Print attribute values
print(obj.num)
print(obj.string)
public class MyClass { // The class
public int num; // Attribute (int variable)
public String string; // Attribute (string variable)
public static void main(String[] args) {
MyClass obj = new MyClass(); // Create an object of MyClass
// Access attributes and set values
obj.num = 25;
obj.string = "Welcome to ScholarHat";
// Print attribute values
System.out.println(obj.num);
System.out.println(obj.string);
}
}
#include
#include
class MyClass { // The class
public: // Access specifier
int num; // Attribute (int variable)
std::string string; // Attribute (string variable)
};
int main() {
MyClass obj; // Create an object of MyClass
// Access attributes and set values
obj.num = 25;
obj.string = "Welcome to ScholarHat";
// Print attribute values
std::cout << obj.num << "\n";
std::cout << obj.string;
return 0;
}
Output
25
Welcome to ScholarHat
Read more: Object Oriented Programming (OOPs) Concepts in C++
4. What are the differences between Structured Programming and Object Oriented Programming?
Object-Oriented Programming | Structured Programming |
Object-oriented programming is built on objects having a state and behavior. | A program’s logical structure is provided by structural programming, which divides programs into their corresponding functions. |
It follows a bottom-to-top approach. | It follows a Top-to-Down approach. |
Restricts the open flow of data to authorized parts only providing better data security. | No restriction to the flow of data. Anyone can access the data. |
Enhanced code reusability due to the concepts of polymorphism and inheritance. | Code reusability is achieved by using functions and loops. |
In this, methods are written globally and code lines are processed one by one i.e., Run sequentially. | In this, the method works dynamically, making calls as per the need of code for a certain time. |
Modifying and updating the code is easier. | Modifying the code is difficult as compared to OOPs. |
Data is given more importance in OOPs. | Code is given more importance |
5. What are the main features of OOPs?
Below are the four main features of OOPs:
- Inheritance
- Encapsulation
- Polymorphism
- Data Abstraction
6. How is a class different from a structure?
Class | Structure |
Classes are of reference types | Structures are of value types |
It is allocated to heap memory | It is allocated on stack memory |
Allocation is cheaper in the large reference type | Allocation is cheaper in value type than a reference type |
It has limitless features | It has limited features |
A class is used in large programs | A structure is used in small programs |
It contains a constructor and a destructor | It contains a parameterized or static constructor |
It uses a new() keyword every time it creates instances | It can easily create instances with or without any keywords. |
A class can inherit from another class. | A structure is not allowed to inherit |
The data members of a class can be protected | The data members of a structure can’t be protected |
Function members can be abstract or virtual | Function members can’t be abstract or virtual |
Two different variables of class can include the reference of a similar object | Each variable contains its copy |
7. List out a few advantages of using OOPs.
- Modularity: OOP promotes modularity by encapsulating data and methods into objects, facilitating easy maintenance and updates.
- Reusability: Objects can be reused in different parts of the program or other projects.
- Readability: OOP enhances code readability with a clear structure, making it easier for developers to understand and collaborate.
- Scalability: OOP accommodates the growth of software projects, allowing the creation of large, complex systems with manageable components.
- Flexibility: Polymorphism and inheritance provide flexibility, allowing the creation of versatile and adaptable code.
- Data abstraction: OOP hides complex implementation details through data abstraction, exposing only essential features for user interaction.
- Code maintenance: OOP simplifies code maintenance by isolating changes to specific objects or classes, reducing the risk of unintended consequences in other parts of the code.
8. What is encapsulation?
Encapsulation helps to wrap up the functions and data together in a single unit. By privatizing the scope of the data members it can be achieved. This particular feature makes the program inaccessible to the outside world.
Example illustrating Encapsulation
class MyClass { // The class
public: // Access specifier
int num; // Attribute (int variable)
std::string string; // Attribute (string variable)
// class function
void show_data() {
// code
};
In the above example, we bundled together the variables num, string, and the function show_data() into a class named MyClass. Encapsulation ensures that only member functions of a class can access its data, which results in data hiding.
9. What do you understand about Access Specifiers/Access Modifiers? What are their types?
Access specifiers define how the members (data members and member functions) of a class can be accessed. They allow us to determine which class members are accessible to other classes and functions, and which are not.
There are three access specifiers in object-oriented programming:
- public
- private
- protected
These modifiers are crucial in achieving Encapsulation as they control the visibility of internal details and contribute to the overall security and organization of the code.
10. Explain the types of Access Modifiers in detail with examples.
- Public Access Modifier: This employs a public keyword to create public members (data and functions). The public members are accessible from any part of the program i.e. from inside or outside the class.
Example of public Access Specifier
#include <iostream> using namespace std; class ScholarHat { public: // Public access specifier int x; // Public attribute void display() { //// Public method cout << "x = " << x << endl; } }; int main() { ScholarHat myObj; myObj.x = 70; myObj.display(); return 0; }
- Here, we have created a class named
ScholarHat
, which contains apublic
variablex
and a public functiondisplay()
. - In
main()
, we have created an object of theScholarHat
class namedmyObj
. - We then access the
public
elements directly by using the codesmyObj.x
andmyObj.display()
.
Output
x = 70
- Here, we have created a class named
- Private Access Modifier: This employs a private keyword to create private members (data and functions). The private members can only be accessed from within the class.
Example of private Access Specifier
#include <iostream> using namespace std; class ScholarHat { private: // Private access specifier int y; //private attribute public: // Public access specifier int x; // Public attribute void display() { // Public method cout << "y = " << y << ", x = " << x << endl; } }; int main() { ScholarHat myObj; myObj.x = 70; myObj.y = 80 myObj.display(); return 0; }
Here,
y
is aprivate
member. It cannot be accessed outside the class. Therefore the program shows an error on compilation.Output
prog.cpp: In function ‘int main()’: prog.cpp:18:9: error: ‘int ScholarHat::y’ is private within this context 18 | myObj.y = 70; | ^ prog.cpp:5:9: note: declared private here 5 | int y; //private attribute | ^
- Protected Access Modifier: This employs the protected keyword to create protected members (data and function). The protected members can be accessed within the class and from the derived class.
Example of protected Access Specifier
class User { protected int year = 2024; protected void printYear() { System.out.println("Its " + year + " !!"); } public static void main(String[] args) { User user = new User(); System.out.println(user.year); user.printYear(); } }
- The User class has a protected member variable year and a protected method printYear().
- In the main method, we create an instance of the User class.
- We can access the protected member variable year directly within the same package.
- We call the printYear() method, which prints the value of the year variable. This method is accessible within the same package.
Read More: Access Modifiers in C++
11. Compare classes and objects in OOP.
Class | Object |
Class is a logical entity | An object is a physical entity |
Class is a template from which an object can be created | An object is an instance of the class |
Class is a prototype that has the state and behavior of similar objects | Objects are entities that exist in real life such as a mobile, mouse, or a bank account |
Class is declared with a class keyword | An object is created via a new keyword |
During class creation, there is no allocation of memory | During object creation, memory is allocated to the object |
There is only a one-way class defined using the class keyword | Object creation can be done in many ways such as using new keyword, newInstance() method, clone(), and factory method |
Cannot be used directly for storing data or performing actions | Used to store data and perform actions as defined by its class |
Every class is defined once and sets a template for what objects created from it will be like. | Objects can be numerous, each created from the class template but with potentially unique characteristics. |
12. What is meant by constructor?
A constructor is a special member function invoked automatically when an object of a class is created. It is generally used to initialize the data members of the new object. They are also used to run a default code when an object is created. The constructor has the same name as the class and does not return any value.
Example
MyClass obj = new MyClass();
In the above code, the method called after the “new” keyword - MyClass(), is the constructor of this class. This will help to instantiate the data members and methods and assign them to the object obj.
13. Explain the types of constructors in C++.
- Default Constructor: It is a constructor in C++ with no parameters.
Example of C++ Default Constructor
#include <iostream> using namespace std; class Student { public: Student() { cout << "Default Constructor Invoked" << endl; } }; int main() { Student s1; // Creating an object of Student class Student s2; return 0; }
The above C++ code in C++ Online Compiler has a default constructor named
Student()
. When the objectss1
ands2
of theStudent
class is created, the constructorStudent()
gets invoked and prints the statement written inside itOutput
Default Constructor Invoked Default Constructor Invoked
- Parameterized Constructor: It is a constructor in C++ with parameters.
Example of C++ Parametrized Constructor
#include <iostream> using namespace std; class Student { public: int id; // data member (also instance variable) string name; // data member (also instance variable) float marks; Student(int i, string n, float m) { id = i; name = n; marks = m; } void display() { cout << id << " " << name << " " << marks << " " << endl; } }; int main() { Student s1 = Student(101, "Pradnya", 78); // creating an object of Student Student s2 = Student(102, "Pragati", 89); s1.display(); s2.display(); return 0; }
Here the class
Student
has a parametrized constructorStudent(int i, string n, float m)
. When the objects1
ands2
of theStudent
class is created, the constructor is called with arguments to initialize the data members of the class.Output
101 Pradnya 78 102 Pragati 89
- Copy Constructor: A member function initializes an object's data members using another object from the same class. In other words, the copy constructor in C++ is used to copy data of one object into another.
Example of C++ Copy Constructor
#include <iostream> using namespace std; class Student { public: int id; // data member (also instance variable) string name; // data member (also instance variable) float marks; Student(int i, string n, float m) { // parametrized constructor id = i; name = n; marks = m; } // Copy constructor with a Student object as a parameter // Copies data of the obj parameter Student(const Student& obj) { id = obj.id; name = obj.name; marks = obj.marks; } void display() { cout << id << " " << name << " " << marks << " " << endl; } }; int main() { Student s1 = Student(101, "Pradnya", 78); // Creating an object of the Student class Student s2 = s1; // Copy contents of s1 to s2 s1.display(); s2.display(); return 0; }
In this program, we have used a copy constructor,
Student(const Student& obj)
to copy the contents of one object of theStudent
class to another.- The parameter of this constructor has the address of an object of the
Student
class. - We then assign the values of the variables of the
obj
object to the corresponding variables of the object by calling the copy constructor. This is how the contents of the object are copied. - The
const
in the copy constructor's parameterconst Student& obj
is used to ensure that the object being passed as an argument to the copy constructor is not modified within the copy constructor itself. - In
main()
, we then create two objectss1
ands2
and then copy the contents ofs1
tos2
. - The
s2
object calls its copy constructor by passing the address of thes1
object as its argument i.e.&obj = &s1
.
Output
101 Pradnya 78 101 Pradnya 78
- The parameter of this constructor has the address of an object of the
Read More: Constructors and Destructors in C ++
14. How is a constructor different from a method?
Constructors | Methods |
The constructor's name should match that of the Class | Methods should not have the same name as the Class name |
They are used to create, initialize, and allocate memory to the object. | Methods are used to execute certain statements written inside them. |
Constructors are implicitly invoked by the system whenever objects are created. | Methods are invoked when it is called. |
They are invoked using a new keyword while creating an instance of the class (object) | Methods are invoked during program execution |
A constructor does not have a return type | The method has a return type |
A constructor cannot be inherited by the subclass | Methods can be inherited by a sub-class |
15. What is inheritance?
Inheritance in object-oriented programming (OOP) allows one class to inherit the attributes and functions of another. The class that inherits the properties is known as the child class/derived class and the main class is called the parent class/base class. This means that the derived class can use all of the base class's members as well as add its own. Because it reduces the need to duplicate code for comparable classes, inheritance promotes code reusability and maintainability.
Inheritance is an is-a relationship. We can use inheritance only if there is an is-a relationship between two classes.
In the above figure:
- A car is a Vehicle
- A bus is a Vehicle
- A truck is a Vehicle
- A bike is a Vehicle
Here, the Car class can inherit from the Vehicle class, the bus class can inherit from the Vehicle class, and so on.
Read More: What is Inheritance in Java
16. Explain the concept of a base class, subclass, and superclass.
- Base Class: It is the class that other classes inherit from. The base class defines the attributes and methods that its derived classes inherit. In addition, the base class can specify its properties and methods. It is also known as the parent class.
In the above-given figure, the Vehicle is the base class. It would have common properties like speed, color, and methods like start() and stop(). These properties and methods are typical for various types of vehicles.
- Subclass: A subclass inherits the base class's properties and methods. The derived class can inherit all of the base class's attributes and methods or only a subset of them. The derived class may additionally include its own set of properties and methods. It is also known as the child class.
In the above-given figure, the Car inherits from the Vehicle base class. Hence, the Car class will automatically have the properties and methods of the Vehicle class.
- Superclass: A class from which other classes, called subclasses, inherit properties and behaviors. It is used as the base class for one or more derived classes.
In the above-given figure, the Vehicle class is a superclass containing common vehicle properties and methods. Cars and Buses are subclasses, inheriting the properties and methods from the Vehicle class.
17. State the advantages of inheritance.
- Code Reusability: Inheritance allows you to create new classes that are based on existing classes, so you can reuse existing code and avoid rewriting the same functionality. This saves a lot of time and effort when you’re creating new programs.
- Organization: Inheritance helps you organize your code in a logical and structured way. You can create a hierarchy of related classes that makes it easy to understand how your code works and how different parts of your program are related.
- Polymorphism: Inheritance facilitates polymorphism, which allows you to treat objects of derived classes as objects of their base class, enabling you to write code that operates on base-class pointers or references but can work with objects of derived classes at runtime.
- Overriding: Inheritance allows you to override methods in a derived class, which means you can change the way a method works without changing the original code.
- Abstraction: Inheritance allows you to create abstract classes that define the basic behavior of a group of related classes while leaving the implementation details to the derived classes.
18. Describe Polymorphism.
Polymorphism is made up of two words, poly means more than one and morphs means forms. So, polymorphism means the ability to take more than one form. This property makes the same entities such as functions, and operators perform differently in different scenarios. You can perform the same task in different ways.
In the given figure, a person when a student pays his bills. After completing his studies, the same person becomes a millionaire and pays bills of various types. Here the person is the same and his functions are also the same. The only difference is in the type of bills.
19. List out the object-oriented programming languages.
- Java
- C++
- Python
- C#
- Ruby
- PHP
- TypeScript
20. How much memory does a class occupy?
Classes do not use memory. They merely serve as a template from which items are made. Now, objects initialize the class members and methods when they are created, using memory in the process.
Intermediate OOPs Interview Questions & Answers
21. Is it always necessary to create objects from class?
No, it is not always necessary to create objects from a class. A class can be used to define a blueprint for creating things, but it is not required to create an object from a class to use its methods or access its properties.
22. What are the different types of polymorphism?
There are two types of polymorphism. These are:
- Compile time Polymorphism: In this, the compiler at the compilation stage knows which functions to execute during the program execution. It matches the overloaded functions or operators with the number and type of parameters at the compile time.
The compiler performs compile-time polymorphism in two ways:
- Function Overloading: If two or more functions have the same name but different numbers and types of parameters, they are known as overloaded functions.
- Operator Overloading: It is function overloading, where different operator functions have the same symbol but different operands. And, depending on the operands, different operator functions are executed. The operands here are the user-defined data types like objects or structures and not the basic data types.
- Runtime Polymorphism: In this, the compiler at the compilation stage does not know the functions to execute during the program execution. The function call is not resolved during compilation, but it is resolved in the run time based on the object type. This is also known as dynamic binding or late binding. This is achieved through function overriding.
- When you redefine any base class method with the same signature i.e. same return type, number, and type of parameters in the derived class, it is known as function overriding.
Read More: Polymorphism in Java: Compile-Time and Runtime Polymorphism
23. Differentiate between static and dynamic binding.
Parameters | Static Binding | Dynamic Binding |
Time of binding | Happens at compile time | Happens at run time |
Actual object | The actual object is not used for binding | The actual object is used for binding |
Also known as | early binding because binding happens during compilation | late binding because binding happens at run time |
Example | Method overloading | Method overriding |
Methods of binding | Private, static, and final methods show static binding. Because they cannot be overridden. | Other than private, static and final methods show dynamic binding. Because they can be overridden. |
24. Explain the virtual function and pure virtual function.
A virtual function is a member function of a class, and its functionality can be overridden in its derived class. A virtual function can be declared using a virtual keyword in C++. In Java, every public, non-static, and non-final method is a virtual function. Python methods are always virtual.
Example of a virtual function
#include
class Base {
public:
// Virtual function
virtual void display() {
std::cout << "Display from Base class" << std::endl;
}
};
class Derived : public Base {
public:
// Override virtual function
void display() override {
std::cout << "Display from Derived class" << std::endl;
}
};
int main() {
// Base class pointer pointing to a Derived class object
Base* ptr = new Derived();
// Call the virtual function
ptr->display();
delete ptr; // Freeing allocated memory
return 0;
}
The derived class Derived overrides the display() function. In the main() function, we create a pointer of type Base that points to a Derived object. When we call ptr->display(), the overridden function in Derived is invoked due to dynamic polymorphism.
Output
Display from Derived class
Pure Virtual Function: It is a do-nothing virtual function declared in the base class with no definition relative to the base class. A base class containing the pure virtual function cannot be used to declare the objects of its own. Such classes are known as abstract base classes.
The main objective of the base class is to provide the traits to the derived classes and to create the base pointer used for achieving the runtime polymorphism.
Example of a pure virtual function
// Abstract class with abstract method
abstract class Shape {
// Abstract method (no implementation)
abstract void draw();
}
// Concrete subclass implementing the abstract method
class Circle extends Shape {
// Implementation of the abstract method
void draw() {
System.out.println("Drawing a circle");
}
}
// Subclass implementing the abstract method
class Rectangle extends Shape {
// Implementation of the abstract method
void draw() {
System.out.println("Drawing a rectangle");
}
}
public class Main {
public static void main(String[] args) {
// Creating objects of subclasses
Shape circle = new Circle();
Shape rectangle = new Rectangle();
// Calling the draw method on objects of concrete subclasses
circle.draw();
rectangle.draw();
}
}
There's an abstract class Shape with an abstract method draw(). We then have two subclasses Circle and Rectangle, each implementing the draw() method with their specific implementation. In the main method, we create objects of the subclasses, circle, and rectangle, and call the draw() method on them. The specific implementation of the draw() method for each subclass is invoked based on the object's runtime type.
Output
Drawing a circle
Drawing a rectangle
Read More: Virtual Functions in C++
25. What is Abstraction?
Abstraction means providing only the essential details to the outside world and hiding the internal details, i.e., hiding the background details or implementation. Abstraction is a programming technique that depends on the separation of the interface and implementation details of the program.
26. What is an interface?
An interface refers to a special type of class, which contains methods, but not their definition. Only the declaration of methods is allowed inside an interface. To use an interface, you cannot create objects. Instead, you need to implement that interface and define the methods for their implementation.
Example of interface implementation
// Define the interface
interface Animal {
public void makeSound();
}
// Define a class that implements the interface
class Cat implements Animal {
public void makeSound() {
System.out.println("Meow");
}
}
// Define another class that implements the interface
class Dog implements Animal {
public void makeSound() {
System.out.println("Woof");
}
}
// Test the interface and the classes that implement it
public class InterfaceExample {
public static void main(String[] args) {
Animal myCat = new Cat();
Animal myDog = new Dog();
myCat.makeSound();
myDog.makeSound();
}
}
This example in the Java Online Editor shows how to use the "Animal" interface and its two implementing classes, "Cat" and "Dog." It enables the "makeSound()" method, which prints out the corresponding noises "Meow" and "Woof" when called in the "main" method, to be called by objects of these types.
Output
Meow
Woof
Read More: Interfaces and Data Abstraction in C++
27. How is an Abstract class different from an Interface?
Abstract Class | Interface |
When an abstract class is inherited, however, the subclass is not required to supply the definition of the abstract method until and unless the subclass uses it. | When an interface is implemented, the subclass is required to specify all of the interface’s methods as well as their implementation. |
An abstract class can have both abstract and non-abstract methods. | An interface can only have abstract methods |
An abstract class can have final, non-final, static, and non-static variables | The interface has only static and final variables. |
Abstract class doesn’t support multiple inheritance | An interface supports multiple inheritance. |
Read More: Abstract Class in Java: Concepts, Examples, and Usage
28. Describe the use of the super keyword.
super keyword in Java is used to identify or refer to parent (base) class.
- We can use super to access the superclass constructors and call methods of the superclass.
- When method names are the same in the superclass and subclass, the super keyword refers to the superclass.
- To access the same name data members of the parent class when they are present in parent and child class.
- Super can be used to make an explicit call to no-arg and parameterized constructors of the parent class.
- Parent class method access can be done using super when the child class has a method overridden.
class DotNetTricks {
public void print() { // overridden function
System.out.println("Welcome to DotNetTricks");
}
}
class ScholarHat extends DotNetTricks {
@Override
public void print() { // overriding function
// call method of the superclass
super.print();
System.out.println("Welcome to ScholarHat");
}
}
public class Main {
public static void main(String[] args) {
ScholarHat obj1 = new ScholarHat();
// Call the print() function
obj1.print();
}
}
Here, the super keyword in the Java Online Editor is used to call the print() method present in the superclass.
Output
Welcome to DotNetTricks
Welcome to ScholarHat
Read More: What is Inheritance in Java: Types of Inheritance in Java
29. What is a friend function in C++?
A friend function is a friend of a class that is allowed to access public, private, or protected data in that same class without being a member of that class. They are the non-member functions that can access and manipulate the private and protected members of the class for they are declared as friends.
A friend function can be:
- A global function
- A member function of another class
#include
class MyClass;
// Declaration of the friend function
void display(const MyClass& obj);
class MyClass {
private:
int num;
public:
MyClass(int n) : num(n) {}
// Declare the friend function as a friend
friend void display(const MyClass& obj);
};
// Definition of the friend function
void display(const MyClass& obj) {
std::cout << "Friend function: The value of num is " << obj.num << std::endl;
}
int main() {
MyClass obj(42);
// Call the friend function
display(obj);
return 0;
}
- Here, the class MyClass has a private member variable num.
- The friend function display() takes a MyClass object as a parameter. This function is declared as a friend of the MyClass class, giving it access to the private members of MyClass.
- Inside the display function, we can access the private member num of the MyClass object.
- In the main function, we create an object of MyClass and call the display function, passing the object as an argument.
Output
Friend function: The value of num is 42
30. What are some other programming paradigms other than OOPs?
- Functional Programming: This emphasizes using functions and immutable data to solve problems.
- Procedural Programming: This emphasizes breaking a program into small procedures or functions to improve readability and maintainability.
- Logic Programming: This emphasizes using logic and mathematical notation to represent and manipulate data.
- Event-driven Programming: This emphasizes handling events, and the control flow is based on the events.
31. Distinguish between Method Overloading and Method Overriding.
Parameters | Method Overloading | Method Overriding |
Definition | Multiple methods in the same class | Method in a subclass with the same name as in the superclass |
Inheritance | Not necessary, can be in the same class | Must have the same method name and parameter list as the superclass |
Signature | Must have different parameter lists | Must have the same method name and parameter list as the superclass |
Return Type | Can be different | Must be the same as the method in the superclass |
Usage | Provides multiple methods with the same name but different parameters | Allows a subclass to provide a specific implementation of a method defined in the superclass |
32. Is Operator overloading supported in Java?
No, operator overloading is not supported in Java. Unlike languages like C++ where you can redefine the behavior of operators for user-defined types, Java does not allow you to overload operators.
In Java, operators such as +, -, *, /, etc., have predefined meanings for built-in types (like int, double, String, etc.), and you cannot change their behavior for your custom classes or types. Instead, Java provides methods (such as toString(), equals(), compareTo(), etc.) and operator-like methods (such as + for concatenating strings) to achieve similar functionality.
33. How will you differentiate a class from an interface?
Class | Interface |
A template for creating objects, defining their state (attributes) and behavior (methods) | A collection of abstract methods (and sometimes constants) that a class can implement |
Contains concrete implementations of methods and properties | Does not contain any method implementation. Only declares method signatures |
A class can extend another class, inheriting its properties and methods | A class implements an interface, agreeing to perform the specific behaviors outlined in the interface |
Typically, a class cannot extend more than one class (depends on the language, but it is true for languages like Java) | A class can implement multiple interfaces, allowing for a form of multiple inheritances |
To encapsulate data and provide functionality | To define a contract that implementing classes must follow, ensuring a certain level of functionality |
34. What are the operators that cannot be overloaded?
Following are the operators that cannot be overloaded:
- Scope Resolution (::)
- Member Selection (.)
- Member selection through a pointer to function (.*)
35. Is it possible for the static method to use nonstatic members?
In Java, a static method cannot directly access or use non-static (instance) members of a class. This is because static methods are associated with the class itself, rather than with instances of the class. Non-static members, on the other hand, belong to individual instances of the class and require an object reference to be accessed.
Advanced OOPs Interview Questions & Answers for Experienced
36. What is meant by Garbage Collection in OOPs?
Object-oriented programming revolves around entities like objects. Each object consumes memory and there can be multiple objects of a class. So if these objects and their memories are not handled properly, then it might lead to certain memory-related errors and the system might fail.
Garbage Collection (GC) is an automatic memory management process in programming languages. It involves identifying and reclaiming memory occupied by objects no longer in use by the program. Instead of requiring manual memory deallocation, which can lead to memory leaks and bugs, GC tracks and frees up memory occupied by objects that are no longer reachable or referenced in the program.
37. Why new keyword is used in Java?
When we create an instance of the class, i.e. objects, we use the Java keyword new. It allocates memory in the heap area where JVM reserves space for an object. Internally, it invokes the default constructor as well.
Syntax
Class_name obj = new Class_name();
38. Explain hybrid and hierarchical inheritance.
- Hybrid Inheritance: It is the combination of more than one type of inheritance i.e. hybrid variety of inheritance.
Example to demonstrate hybrid inheritance
#include <iostream> using namespace std; class Birds { public: Birds() { cout << "Birds are of two types" << endl; } }; class Oviparous : public Birds { public: Oviparous() { cout << "Oviparous birds lay eggs." << endl; } }; class Sparrow { public: void name2() { cout << "I am a sparrow." << endl; } }; class HybridSparrow : public Oviparous, public Sparrow { public: void display() { name2(); cout << "HybridSparrow class is a combination of Oviparous and Sparrow." << endl; } }; int main() { HybridSparrow h1; h1.display(); return 0; }
- In the above code in C++ Online Compiler, class
Oviparous
inherits from classBirds
. This is a single inheritance. - The class
HybridSparrow
inherits from both classOviparous
and classSparrow
. This is multiple inheritance. - The
Oviparous
class inheritsBirds
class which is further inherited by theHybridSparrow
class. This is a multilevel inheritance.
Output
Birds are of two types Oviparous birds lay eggs. I am a sparrow. HybridSparrow class is a combination of Oviparous and Sparrow.
- In the above code in C++ Online Compiler, class
- Hierarchical Inheritance: If more than one derived class inherits a single base class, a hierarchy of base classes is formed and so it is known as hierarchical inheritance.
Example to demonstrate hierarchical inheritance
#include <iostream> using namespace std; class Birds { public: void type() { cout << "I'm an oviparous bird" << endl; } }; class pigeon: public Birds{ public: void name1() { cout << "I am a pigeon." << endl; } }; class Sparrow: public Birds { public: void name2() { cout << "I am a sparrow." << endl; } }; int main() { pigeon p; // object of pigeon class cout << "\npigeon Class:" << endl; p.type(); // base class method p.name1(); Sparrow h; // object of Sparrow class cout << "\nSparrow Class:" << endl; h.type(); // base class method h.name2(); return 0; }
Here, both the
pigeon
andSparrow
classes are derived from theBirds
class. As such, both the derived classes can access thetype()
function belonging to theBirds
class.Output
pigeon Class: I'm an oviparous bird I am a pigeon. Sparrow Class: I'm an oviparous bird I am a sparrow.
Read More:
39. What is an inline function?
An inline function is a particular type of function in programming where the code from the function is inserted directly into the calling code instead of having a separate set of instructions in memory. It reduces overhead and allows for better optimization.
40. What are errors and what are exceptions? Differentiate between them.
Exceptions are runtime errors. Many a time, your program looks error-free during compilation. But, at the time of execution, some unexpected errors or events or objects come to the surface and the program prints an error message and stops executing.
Errors represent serious system or hardware failures, typically leading to program termination, and are usually beyond the program’s control.
Differences between Errors and Exceptions
Parameters | Errors | Exceptions |
Typical Use | Represents serious system failures | Used for manageable exceptional conditions in programs |
Examples | OutOfMemoryError, StackOverflowError | NullPointerException, ArrayIndexOutOfBoundsException |
Handling | Often cannot be recovered, leads to program termination | Can be caught and handled to prevent program termination |
Cause | Typically caused by the system or hardware | Caused by program conditions that can be handled |
41. How does Java implement multiple inheritance?
Multiple inheritance is not supported by Java using classes. To achieve multiple inheritance in Java, we must use the interface. Interfaces ensure that classes follow predetermined behaviors by defining method contracts without implementing them.
Example
// Define two interfacesinterface Animal {
void eat();
void sleep();
}interface Bird {
void fly();
}// Create a class that implements both interfaces
class Sparrow implements Animal, Bird {
@Override
public void eat() {
System.out.println("Sparrow is eating.");
} @Override
public void sleep() {
System.out.println("Sparrow is sleeping.");
} @Override
public void fly() {
System.out.println("Sparrow is flying.");
}
}public class Main {
public static void main(String[] args) {
Sparrow sparrow = new Sparrow();
sparrow.eat(); // Calls the eat() method from the Animal interface
sparrow.sleep(); // Calls the sleep() method from the Animal interface
sparrow.fly(); // Calls the fly() method from the Bird interface
}
}
The "Animal" and "Bird" interfaces are defined in this code, along with the class "Sparrow," which implements both interfaces. The 'eat()','sleep()', and 'fly()' methods are implemented by the 'Sparrow' class. It generates a "Sparrow" object and invokes its methods in the "main" method to show multiple inheritance through interfaces.
Output
Sparrow is eating.
Sparrow is sleeping.
Sparrow is flying.
Read More: Multiple Inheritance in Java
42. Explain the difference between abstract class and method.
Abstract Class | Abstract Method |
An object cannot be created from the abstract class | The abstract method has a signature but does not have a body |
Subclass created or inherit abstract class to access members of abstract class | It is compulsory to override abstract methods of the superclass in their sub-class |
Abstract classes can contain abstract methods or non-abstract methods | Class containing abstract method should be made the abstract class |
43. Distinguish encapsulation from abstraction.
Encapsulation | Abstraction |
Encapsulation is the process or method of containing the information in a single unit and providing this single unit to the user. | Abstraction is the process or method of gaining information |
Main feature: Data hiding. It is a common practice to add data hiding in any real-world product to protect it from the external world. In OOPs, this is done through specific access modifiers | Main feature: reduce complexity, promote maintainability, and also provide a clear separation between the interface and its concrete implementation |
problems are solved at the implementation level. | problems are solved at the design or interface level |
It is a method to hide the data in a single entity or unit along with a method to protect information from outside | It is the method of hiding the unwanted information |
encapsulation can be implemented using access modifiers i.e. private, protected, and public | We can implement abstraction using abstract classes and interfaces |
implementation complexities are hidden using abstract classes and interfaces | the data is hidden using methods of getters and setters |
the objects that result in encapsulation need not be abstracted | The objects that help to perform abstraction are encapsulated |
Encapsulation hides data and the user cannot access the same directly | Abstraction provides access to specific parts of data |
Encapsulation focus is on “How” it should be done | Abstraction focus is on “what” should be done |
44. What is the use of the finalize method?
The finalize method helps to perform cleanup operations on the resources that are not currently used. The finalize method is protected, and it is accessible only through this class or by a derived class.
45. Can a Java application be run without implementing the OOPs concept?
No. Java applications are based on Object-oriented programming models or OOPs concept, and hence they cannot be implemented without it.
46. What are the differentiating points between extends and implements?
Extends | Implements |
A class can extend another class (a child extending parent by inheriting his characteristics). An interface can also inherit (using the keyword extends) another interface | A class can implement an interface |
Sub-class extending superclass may not override all of the superclass methods | Class implementing interface has to implement all the methods of the interface |
A class can only extend a single superclass | A class can implement any number of interfaces |
Interface can extend more than one interface | An interface cannot implement any other interface |
Syntax: class Child extends class Parent | Syntax: class Hybrid implements Rose |
47. Is Java a pure Object Oriented language?
Java is not an entirely pure object-oriented programming language. The following are the reasons:
- Java supports and uses primitive data types such as int, float, double, char, etc.
- Primitive data types are stored as variables or on the stack instead of the heap.
- In Java, static methods can access static variables without using an object, contrary to object-oriented concepts.
48. Can you create an instance of an abstract class?
No, an instance of the Abstract class cannot be created.
However, you can create an instance of a concrete subclass that extends the abstract class. This concrete subclass must provide implementations for all the abstract methods defined in the abstract class. Then you can create an instance of this subclass.
abstract class AbstractClass {
abstract void abstractMethod(); // Abstract method
}
class ConcreteClass extends AbstractClass {
void abstractMethod() {
System.out.println("Implementation of abstractMethod in ConcreteClass");
}
}
public class Main {
public static void main(String[] args) {
// AbstractClass obj = new AbstractClass(); // Error: Cannot instantiate abstract class
ConcreteClass obj = new ConcreteClass(); // Creating an instance of ConcreteClass
obj.abstractMethod(); // Calling the implemented method
}
}
Here,
- AbstractClass is an abstract class with an abstract method abstractMethod().
- ConcreteClass is a concrete subclass of AbstractClass that provides an implementation for the abstractMethod().
- In the main method, we create an instance of ConcreteClass, which is allowed because it provides implementations for all abstract methods defined in AbstractClass.
49. What are manipulators?
Manipulators are the functions that can be used in conjunction with the insertion (<<) and extraction (>>) operators on an object. Examples are endl and setw.
50. Define the term 'composition' in OOP.
Composition in Object-Oriented Programming refers to a design principle where a class includes instances of other classes to add functionality or behavior. This "has-a" relationship allows for flexible and reusable code.
Summary
So here we saw in depth almost all the important OOP interview questions and answers from a basic to advanced level. Go through it before going for your interview.
Take our free skill tests to evaluate your skill!
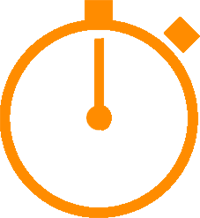
In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.