10
meiAn Introduction to JSX - React JSX
Introduction
React's JSX (JavaScript XML) syntax extension allows programmers to construct JavaScript code that resembles HTML. Offering a more declarative & intuitive approach, it makes the process of creating user interfaces simpler. Developers can define reusable React components with JSX by combining JavaScript and syntax like HTML. Before JSX code is presented to the DOM, it is converted into standard JavaScript using programs like Babel. This makes it possible to create dynamic material and update it effectively. React JS certification training in JSX speeds up UI development in React applications by fusing the strength of JavaScript with the well-known HTML structure.
What is JSX?
JSX, also known as JavaScript syntactic Extension, is a strong and user-friendly JavaScript syntactic extension that is frequently used in React. It makes it simpler to construct user interfaces by allowing developers to simply write HTML-like code in their JavaScript files.
Here is an example of JSX code in a React component:
import React from 'react';
const MyComponent = () => {
return (
<div>
<h1>Hello, JSX!</h1>
<p>This is an example of JSX in React.</p>
</div>
);
};
export default MyComponent;
In the given example, the parenthetically contained JSX code used for MyComponent, a React component, has syntax similar to HTML. The HTML components that will be shown in the DOM are represented by the JSX code.
Read More - Top 50 Mostly Asked React Interview Question & Answers
Understanding JSX Syntax
A syntax that is very similar to HTML can be used to write HTML components in JSX. For example, we can write <h1>Hello, World!</h1>instead of using React.createElement('h1', {}, 'Hello, World!').
JSX as Syntactic Sugar
JSX is frequently referred to as "syntactic sugar" since it makes the creation of React components simpler. JSX is internally converted into JavaScript code using programs like Babel. It is changed using React.createElement() method into a more complex form.
Why JSX?
Benefits of JSX in React
JSX is advantageous for React development in several ways:
- Combining Markup & Logic: JSX enables developers to incorporate JavaScript logic and HTML markup in a single file, simplifying the codebase's understanding and maintenance.
- Improved TypeScript Integration: JSX and TypeScript work together seamlessly to offer better type-checking and a better development environment.
- Enhanced Security: JSX automatically eludes dynamic material to protect users from common security flaws like cross-site scripting (XSS) assaults.
How Does JSX Work?
Here are the steps of how JSX functions in React:
- Write JSX: Create JSX to describe the UI structure by combining JavaScript and HTML-like syntax.
- Babel's transformations: Use Babel to translate JSX into standard JavaScript.
- Describe React Components: Use JSX to build reusable UI components.
- JavaScript expressions to embed: To add dynamic material and computations use curly brackets.
- Transpile JSX: JSX elements are compiled into React.createElement() functions.
- Render JSX: Render transpile JSX into the DOM, modifying the UI as required.
Read More - React developer salary in India
Getting Started with JSX
Setting up Babel and Webpack
You must configure Babel and Webpack to use JSX in your project. In contrast to Webpack, which assists with module bundling as well as build optimizations, Babel is in charge of transpiling JSX code. You can manually set up Babel and Webpack or use well-known programs like Create React App to create a React project that comes preconfigured with JSX compatibility.
Using Create React App
Popular tool Create React App creates a new React project with all essential configurations, including JSX support. Managing the setup and building process for you makes it easier to get started with React & JSX.
JSX Expressions
JavaScript Expressions Embedded: Developers can use curly brackets to enclose JavaScript expressions thanks to JSX. This allows JSX elements to contain dynamic content and logic. As an example, <h1>{name}</h1> will display the value of the name variable inside the <h1> element.
JSX Expression Execution: Any legitimate JavaScript expression can be a JSX expression. They could consist of arithmetic calculations, function calls, or even complex logic. The runtime evaluation of the expressions produces a result that is presented as a JSX element.
Here is an example of code that will help you better
import React from 'react';
function App() {
const name = 'Mohan Kumar';
const age = 25;
return (
<div>
<h1>Hello, {name}!</h1>
<p>You are {age} years old.</p>
<p>Next year, you will be {age + 1}.</p>
</div>
);
}
export default App;
The code shows how variables like name & age are represented by the JavaScript expressions "name" and "age," respectively, in JSX. The message "You will be 26 next year" is displayed when the phrase "age + 1" evaluates to 26.
JSX and React Components
Creating UI Components with JSX
React's reusable UI components can be easily made by using JSX. Components can be considered JavaScript classes or functions, & JSX can be used to display these components along with their corresponding children and properties.
Reusing Components in JSX
Reusing components is one of JSX's main benefits. With JSX, you may nest components inside of one another to produce a hierarchical structure that symbolizes your user interface. Complex UIs are now simpler to manage and keep up to date.
JSX Advantages & Disadvantages
Advantages of JSX
- JSX enhances the readability and maintainability of code by allowing developers to write HTML-like syntax within JavaScript.
- JSX provides the ability to create reusable components, leading to more modular and organized code.
- JSX offers better performance compared to traditional templating solutions by optimizing the rendering process.
- JSX allows for the integration of JavaScript expressions within the HTML-like syntax, enabling dynamic rendering of data.
Disadvantages of JSX
- JSX requires an additional build step to transform the HTML-like syntax into valid JavaScript code that browsers can understand.
- JSX can be overwhelming for developers who are not familiar with HTML and CSS concepts.
- JSX can make debugging more challenging due to the mixing of JavaScript and HTML-like syntax.
- JSX may not be suitable for all projects, especially those that require strict separation of concerns between HTML, CSS, and JavaScript.
JSX vs HTML
Differences between JSX and HTML
JSX syntax and HTML syntax are very similar, however, there are some important distinctions to be aware of. Some HTML attributes have distinct names in JSX, and camelCase is used for JSX style attributes rather than kebab-case. In JSX, self-closing tags that are used in HTML must be explicitly closed.
JSX Tags and Attributes
All HTML tags as well as unique React components are supported by JSX. These elements can have extra characteristics and values added to them using JSX style attributes, providing dynamic behavior and interactivity. When using React, you may add unique jsx data attributes to the JSX elements by using the data-* properties. These characteristics provide a means of storing extra jsx data attribute that is accessible and modifiable via JavaScript but is not immediately used by the browser. For a comprehensive understanding, consider enrolling in the Best React JS course.
Wrapping Elements or Children in JSX
When using React, there are two ways to wrap elements or children in JSX:
- Using a parent element: The most typical method of enclosing elements or children is by using a parent element. Simple parent elements like div or span are used to enclose the elements or children.
- Using a fragment: The fragment is a specific kind of JSX element that may be used to group elements collectively without adding a new DOM node. This can be helpful for wrapping elements or children who would otherwise be presented as independent DOM nodes.
Here is a simple example of wrapping children or elements in JSX:
import React from 'react';
const MyComponent = () => {
return (
<div>
<h1>Welcome!</h1>
<p>This is a simple example.</p>
<p>Here's another paragraph.</p>
</div>
);
};
export default MyComponent;
The <h1> and <p> elements in the example are combined by being enclosed in a <div> container. This enhances the readability and organization of JSX code. You can apply styles and manage the collection of items as a whole using the container.
Comments in JSX
React comments in JSX differ slightly from comments in standard JavaScript. Comments in JSX must be enclosed in curly brackets{}.
Here are some guidelines for using comments in JSX:
- Curly braces must be used to enclose comments.
- There is no way to nest comments.
- Within attributes, properties, and children, comments can be used anywhere in JSX.
Here is an easy example showing how to utilize comments in JSX in a React component:
import React from 'react';
const App = () => {
return (
<div>
{/* This is a comment */}
<h1>Hello, World!</h1>
{/* Commented out paragraph */}
{/* <p>Welcome to my website.</p> */}
</div>
);
};
export default App;
JSX conditional attribute
With React, JSX enables you to include JavaScript code that looks like HTML. When using JSX, JSX conditional attribute can be used to conditionally render items or apply particular behavior based on specified circumstances.
Using the if statement or the conditional (ternary) operator is one typical technique to achieve conditional rendering in JSX.
In JSX for React, JSX conditional attributes have the following characteristics:
- Depending on the result of a condition, they can be utilized to render various types of material.
- They can be used to display or conceal things according to the user's permission.
- By using an API, they can dynamically load content.
- Errors or unexpected conditions can be handled with them.
- They are simple to understand and use.
- By simply rendering the relevant content, they can be utilized to enhance the efficiency of your application.
Here is a basic example of a JSX conditional expression used inside a React component:
import React from 'react';
function Greeting(props) {
const isLoggedIn = props.isLoggedIn;
return (
<div>
{isLoggedIn ? (
<h1>Welcome back, User!</h1>
) : (
<h1>Please log in.</h1>
)}
</div>
);
}
export default Greeting;
The isLoggedIn prop is utilized in the Greeting functional component to conditionally render content. If "Welcome back, User!" appears in the <h1> element, then "isLoggedIn" is true. If isLoggedIn is false, the message "Please log in" is displayed in the <h1> element.
Loops in JSX
A collection of data can be iterated over using loops in JSX in React, and each item in the collection can then be rendered with a distinct component. This can be helpful for a number of purposes, including:
- Creating a list of things
- The creation of a data table
- Rendering an image gallery
You can use the following various loop types in React components by using JSX.
- Map Loop: A built-in JavaScript method for arrays called "map" iterates through each element in an array to create a new array of JSX elements.
Example:
import React from 'react';
function MyList(props) {
const items = props.items;
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
}
For...Of Loop:
The for...of the loop is a JavaScript loop that enables you to perform an action on each element while you cycle over the iterable object (like arrays) elements.
Example:
import React from 'react';
function MyList(props) {
const items = props.items;
return (
<ul>
{(() => {
const elements = [];
for (const item of items) {
elements.push(<li key={item.id}>{item.name}</li>);
}
return elements;
})()}
</ul>
);
}
forEach Loop:
A built-in JavaScript method called forEach is used to iterate through each item in an array and carry out a specific action for each one.
Example:
import React from 'react';
function MyList(props) {
const items = props.items;
const elements = [];
items.forEach((item, index) => {
elements.push(<li key={index}>{item}</li>);
});
return <ul>{elements}</ul>;
}
Best Practices of JSX
- For constructing components in React, use the JSX syntax.
- When using components without children, use self-closing tags.
- To incorporate JavaScript expressions into JSX, use curly brackets {}.
- For improved readability, use proper indentation.
- When naming attributes, use camel case.
- For string attribute values, use quotes.
- When applying CSS classes, use className rather than class.
- Wrap all JSX code in a single root element.
- Use JSX expressions in functions as arguments or return values.
- In JSX, avoid using inline styles.
- Keep your JSX code organized and brief.
- Create smaller, reusable components out of complex ones.
- For improved clarity, use meaningful variables and prop names.
Resources for further learning and practice
- Official Redux documentation: Read the documentation at https://legacy.reactjs.org/docs/getting-started.html The official Redux documentation is a comprehensive resource that covers all aspects of Redux, including React Redux.
- Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/react-certification-training. There are numerous online tutorials and courses available that specifically focus on React Redux.
Summary
We have covered the foundations of JSX and its use in developing React in this extensive guide. By fusing JavaScript logic with HTML-like syntax, JSX offers a strong and simple solution to develop user interfaces. We've talked about the advantages of utilizing JSX, transpilation, and the best ways to write JSX code. Developers may easily construct interactive and dynamic UIs with React with a thorough understanding of JSX. To fully realize the promise of developing rich user experiences, incorporate JSX into your React projects.
Take our free skill tests to evaluate your skill!
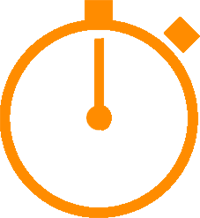
In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.