25
AprData Types in C# with Examples: Value and Reference Data Type
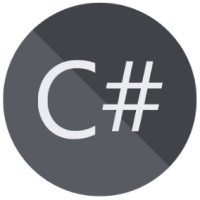
C# Programming For Beginners Free Course
Data Types in C#: An Overview
In the world of programming, data types play a crucial role in defining the kind of data that can be stored and manipulated by a program. As a C# developer, understanding data types is essential for writing efficient and error-free code. In this article, we will delve into the various types of data that can be used in C# programming. In this C# Tutorial, we will explore more about data Types in C# with Examples: Value and Reference Data Types.
What are Data Types in C#?
In C#, data types are used to specify the type of data that a variable can hold. Data types help define the characteristics and constraints of the data, such as whether it's a whole number, a decimal number, a character, a string, and so on. C# provides a rich set of built-in data types to handle various kinds of data.Importance of Data Types in C# programming
- Type Safety: C# is a statically typed language, which means that variables and expressions have predefined data types. Understanding data types ensures that you assign appropriate values to variables and avoid type errors during compilation and runtime.
- Memory Allocation: Data types determine the amount of memory required to store a particular value. By understanding data types, you can optimize memory allocation and avoid unnecessary memory consumption.
- Data Integrity: Different data types have specific ranges and constraints. Understanding data types allows you to choose the appropriate type for a particular data value, ensuring data integrity and preventing data corruption or loss.
- Arithmetic Operations: Data types define the set of valid arithmetic operations that can be performed on the data. By understanding the data types involved in an arithmetic operation, you can ensure accurate results and prevent unexpected behaviour due to implicit conversions or type mismatches.
- Function Signatures: Data types are an essential part of function signatures in C#. By understanding the data types of function parameters and return values, you can correctly call functions, pass arguments, and handle the results.
Read More - C Sharp Interview Questions
Types of Data Types in C#
Data types in C# are mainly divided into two categories:
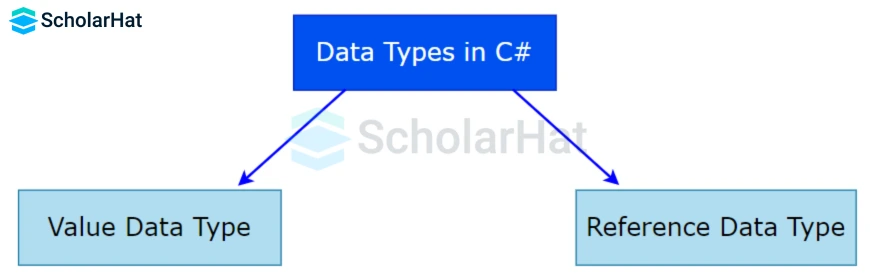
Types | Data Types |
Value Data Type | short, int, char, float, double etc. |
Reference Data Type | String, Class, Object, and Interface |
- Value Data Type in C#
The value data type in C# is not a specific data type itself. Instead, it refers to value types, which are data types that hold their values directly. The value data type stores its value directly in memory. There are 2 types of value data type in C# language:
- Predefined Data Types - such as Integer, Boolean, Float, etc.
- User-defined Data Types - such as Structure, Enumerations, etc.
- int: Signed 32-bit integer.
- float: Single-precision floating-point number.
- double: Double-precision floating-point number.
- decimal: Precise decimal representation with higher precision.
- bool: Boolean value (true or false).
- char: Single Unicode character.
- Reference Data Type in C#
- String: It displays an array of Unicode characters and the type name is “System.String”. So, both strings are equivalent.
Numeric types:
Alias | Type Name | Type | Size(bits) | Range | Default Value |
sbyte | System.Sbyte | signed integer | 8 | -128 to 127 | 0 |
short | System.Int16 | signed integer | 16 | -32768 to 32767 | 0 |
int | System.Int32 | signed integer | 32 | -231 to 231-1 | 0 |
long | System.Int64 | signed integer | 64 | -263 to 263-1 | 0L |
byte | System.byte | unsigned integer | 8 | 0 to 255 | 0 |
ushort | System.UInt16 | unsigned integer | 16 | 0 to 65535 | 0 |
uint | System.UInt32 | unsigned integer | 32 | 0 to 232 | 0 |
ulong | System.UInt64 | unsigned integer | 64 | 0 to 263 | 0 |
Character types:
Alias | Type name | Size In(Bits) | Range | Default value |
char | System.Char | 16 | U +0000 to U +ffff | ‘\0’ |
Structures (structs):
User-defined value types are created using the struct keywords
Example
using System;
namespace ValueTypeTest
{
class ScholarHat
{
static void Main()
{
char a = 'S';
int i = 89;
short s = 56;
long l = 4564;
uint ui = 95;
ushort us = 76;
ulong ul = 3624573;
double d = 8.358674532;
float f = 3.7330645f;
decimal dec = 389.5m;
Console.WriteLine("char: " + a);
Console.WriteLine("integer: " + i);
Console.WriteLine("short: " + s);
Console.WriteLine("long: " + l);
Console.WriteLine("float: " + f);
Console.WriteLine("double: " + d);
Console.WriteLine("decimal: " + dec);
Console.WriteLine("Unsinged integer: " + ui);
Console.WriteLine("Unsinged short: " + us);
Console.WriteLine("Unsinged long: " + ul);
}
}
}
Explanation
This C# code demonstrates the use of various value types. It declares and initializes variables of different value types, including char, int, short, long, uint, ushort, ulong, double, float, and decimal. Then, it prints their respective values using Console.WriteLine().
Output
char: S
integer: 89
short: 56
long: 4564
float: 3.733064
double: 8.358674532
decimal: 389.5
Unsinged integer: 95
Unsinged short: 76
Unsinged long: 3624573
Reference data types in C# are types that store references to objects rather than the actual values. They hold a memory address that points to the location of the object in memory. Reference types are allocated on the heap and are accessed through a reference (or a pointer) to that memory location. The built-in reference types are string and object.
Example:
string s1 = "hello"; // creating through string keyword
string s2 = "welcome"; // creating through String class
- Object: In C#, Object is the direct or indirect ancestor of all kinds—predefined and user-defined, reference types and value types. In essence, it serves as the root class for all C# data types. It requires type conversion before values may be assigned. Boxing describes the process of converting a value type variable into an object.
Example:
using System;
namespace ValueTypeTest
{
class Program
{
static void Main()
{
string a = "Scholar";
a += "for";
a = a + "Scholar";
Console.WriteLine(a);
object obj;
obj = 20;
Console.WriteLine(obj);
Console.WriteLine(obj.GetType());
}
}
}
Output:
ScholarforScholar
20
System.Int32
Conclusion
In this article, We discussed the importance of understanding data types, the common datatypes in C#, and best practices for using datatypes. Now that you have a deeper understanding of datatypes in C#, it's time to put your knowledge into practice and start building amazing applications. Happy coding! Also, Consider our C# Programming Course for a better understanding of all C# concepts.Resources for Further Learning and Practice
Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/csharp-certification-training
There are numerous online tutorials and courses available that specifically focus on C# language.
FAQs
Q1. What is data types C#?
Q2. How many types are there in C#?
Q3. Why do we use datatype in C#?
Take our free csharp skill challenge to evaluate your skill

In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.