25
AprDifference Between C# Const and ReadOnly and Static
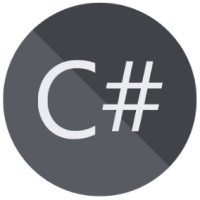
C# Programming For Beginners Free Course
Constant Vs Readonly Vs Static: An Overview
In C#, const, read-only, and static keywords play an important role in designing robust and maintainable code. const and read-only keywords are used tomake a field constant whose value cannot be modified. While the static keyword is used to make members static and can be shared by all the class objects. The purpose of this article is to clarify the differences between const, read-only, and static in C# and how they work in different scenarios.
Hence in this C# tutorial, we will learn when and how to use Constant, Read-only, and Static keywords. Stretching the reach of all c#.net concepts, take into account enrolling in the best Csharp-Programming Course. It will help you in your journey of being a C# and .Net programmer.
Const keyword:
- As long as the value assigned can be fully evaluated at compilation time, we can define a const in C# of any type.
- Remember it is impossible to modify a constant member during runtime, they are defined at compile time only.
- The const keyword is used to declare constants as fields, and they also need to be initialized, as seen below:
Const keyword Example:
public class TestClass
{
public const double TotalDaysInWeek = 7;
public const string FullNameFormat = "Mr./Ms./Mrs" + "{0} {1}";
public const string FullNameFormatEmpty = string.Empty; //Invalid because of string.Empty evaluated on runtime
}
- Constants are static in nature. So, they are static by default and are accessible similarly to static fields, and we can’t use static keywords with const.
- The constant cannot be changed in the application anywhere else in the code as it will give a compilation error.
- Constants have to be Value types (byte, short, ushort, int, uint, long, ulong, char, float, double, decimal, or bool) or enumerations are required for constants.
- The two sorts of reference values that can be found at compile time are strings and references to null. As a result, any other reference type can be declared const as long as null is assigned to it, which is worthless.
- Your constant's scope is restricted to a single assembly, class, or smaller unit. A constant must be completely qualified with the class name in order to be used outside of the class in which it is declared.
- if the const is used outside of the assembly where it is defined! The caller assembly will still see the original value if the assembly that defines the const modifies its value without recompiling because the const's value is swapped at build time.
- So const should be used mainly where values that are not subject to change, or free if the scope of the const is limited to the same assembly.
- Use const when: The constant has compile-time value, and the constant's domain does not change or is limited in scope to a single assembly, class, or method.
Read More - C# Programming Interview Questions
Read-only keyword:
- The read-only keyword can be initialized either at the time of declaration or within the constructor of the same class.
- Therefore, the read-only keyword can be used for run-time constants. A read-only member is like a constant in that it always represents an unchanging value.
- The difference is that a read-only member can be initialized at runtime, in a constructor as well as able to be initialized as they are declared as shown below.
Read-only keyword Example:
Let's see in C# Online Compiler
public class TestCase
{
public readonly double TotalDaysInWeek = 7;
public readonly int TotalMonths;
public TestCase()
{
TotalMonths = 12;
}
}
- Since read-only fields are not limited to values that can be determined at compilation time, it means that they are not changed where they are used at compile time but are the same as other variable lookups.
- This means that you won't have a situation with a constant where a class library can change a const and another assembly using it won't get the updated value.
- It must have a set value by the time the constructor exits.
- A read-only is more usable than a const for constants that are subject to change over time.
- So, things that remain constant (Such as days per week, etc.) can be used with const because we know they never, ever change. But things that may be constant now but after a certain period could conceivably change in the future due to changes in requirements or any policy we can go with read-only instead, especially if they are visible from other assemblies.
- If your read-only field is a value type (primitive or struct) or the type that can't be changed once assigned like string, that field will behave like a constant.
- Use read-only when the constant is a struct, a non-string, and a non-null class, and cannot be determined at compile-time.
- The read-only keyword is evaluated when the instance is created, A read-only member can hold a complex object by using the new keyword at initialization.
- One last thing that makes read-only more efficient is that it can be applied to instance members as well as class (static) members. This means we can provide a constant that applies to all instances of the class.
Real World Example of Read-Only:
using System;
class College {
public readonly string str1;
public readonly string str2;
public readonly string str3 = "student";
public College(string a, string b)
{
str1 = a;
str2 = b;
Console.WriteLine("Display value of string 1 {0}, "
+ "and string 2 {1}", str1, str2);
}
static public void Main()
{
College ob = new College("VJNT College", "Oxford College");
}
}
Output
Display value of string 1 VJNT College, and string 2 Oxford College
Explanation
We have taken read-only variables str1,str2, and 3rd variable called str3 initialized declaration time only. The values of the rest of the read-only variables are assigned Using a constructor In the main method we created a College class object and gave values to variables.
Static-read-only keyword
Here static-read-only is almost similar to the read-only keyword, Perhaps it is used to declare a static field that can only be initialized once, either at the time of declaration or in a static constructor.
Static-read-only keyword Example
Let's see in C# Editor
public class TestCase
{
public static readonly Test testObj = new Test();
}
- static-read-only is specially used if the type of the field is not allowed in a const declaration, or when the value is not known at compile time.
- The difference is that the value of a static-read-only field is set at run time, and can be modified by the containing class, whereas the value of a const field is set to a compile-time constant only.
- static-read-only evaluated when code execution hits class reference (i.e. new instance is created or static method is executed)
- In the static-read-only case, the containing class is allowed to modify it only in the variable declaration (through a variable initializer) or in the static constructor (instance constructors, if it's not static).
Unlock the next level of C#
- Introduction to C#: A Beginner's Guide
- Objects and Classes in C#: Examples and Differences
- Data Types in C# with Examples: Value and Reference Data Type
- Conditional Statements in C#: if, if..else, Nested if, if-else-if
- Scope of Variables in C#: Class Level, Method Level, and Block Level Scope
Summary:
Understanding the differences between const, read-only, and static in C# is essential for writing clean, maintainable, and efficient code. Each keyword serves a specific purpose, and you can choose the right one depending on the requirements of the program. By learning these differences, you can leverage the strengths of each keyword to design robust and flexible software solutions. I hope you will enjoy the tips while programming with C#. I would like to have feedback from my blog readers. Your valuable feedback, questions, or comments about this article are always welcome. Also don't forget to enroll in our .Net Training Course for a better understanding of .net concepts. Enjoy coding..!
FAQs
Q1. What is the difference between static string and const string in C#?
Q2. What is the difference between read-only and const in C#?
Q3. What is the difference between read-only and static read-only?
Take our free csharp skill challenge to evaluate your skill

In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.