25
AprJump Statement in C#: Break, Continue, Goto, Return and Throw
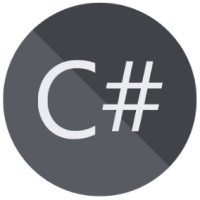
C# Programming For Beginners Free Course
Jump Statement in C#: An Overview
Jump Statements in C# are essential programming tools that allow developers to control the flow of execution within a program. Understanding how "break," "continue," "return," “throw” and "goto" statements work is crucial for building efficient and logically structured C# code. Let's explore these statements and their applications in detail. In this C# Tutorial, we will explore more about jump Statements in C# which will include Understanding how "break," "continue," "return," “throw” and "goto" statements work.
What are Jump Statements in C#?
Jump statements in C# alter the flow of program execution. 'break' exits loops, 'continue' skips the rest of the loop body, and 'return' exits a method, providing flexibility and control in program logic, enabling efficient handling of various conditions and iterations.
There are five keywords in the Jump Statements:
- break
- continue
- goto
- return
- throw
1. Break Statement in C#
The break statement is a powerful tool in the C# programming language that allows developers to control the flow of their code. It is primarily used to exit a loop or switch statement prematurely, based on a certain condition. Understanding how to effectively use the break statement in c# can greatly enhance your programming skills and make your code more efficient.
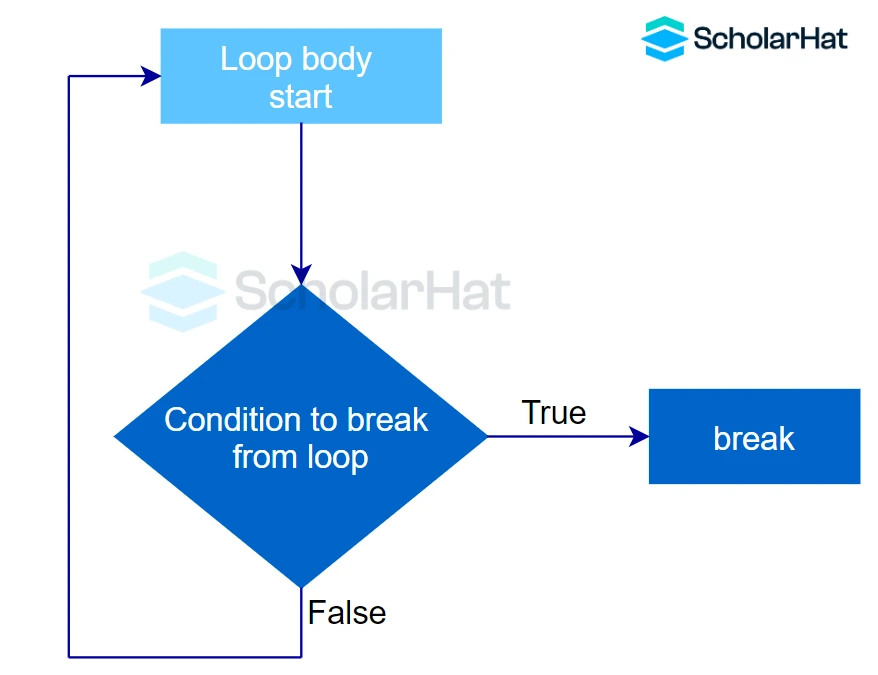
Example
using System;
class Program
{
static void Main()
{
int[] numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
foreach (int num in numbers)
{
if (num % 5 == 0)
{
Console.WriteLine("Found the number: " + num);
break;
}
}
}
}
Explanation
This C# code iterates through the 'numbers' collection. If it finds a number divisible by 5, it prints a message and exits the loop using the 'break' statement, ensuring it only processes the first occurrence of such a number.
Output
The output of this code will be the message "Found the number: " followed by the value of the first number in the "numbers" collection that is divisible by 5. If there is no such number, no output will be generated.
Read More - C Sharp Interview Questions
Examples of using the break statement in loops
The break statement can be used with various types of loops in C#, including for, foreach, and while loops. Here are some examples of how the break statement can be used in different loop scenarios:
1. Using break in a for loop
using System;
class Program
{
static void Main()
{
for (int i = 0; i < 10; i++)
{
if (i == 5)
{
break;
}
Console.WriteLine(i);
}
}
}
Explanation
This code uses a for loop to iterate over the values from 0 to 9. Inside the loop, there is an if statement that checks if the current value of i is equal to 5. If it is, the break statement is executed, which immediately terminates the loop.
Therefore, the loop will iterate and print the values 0, 1, 2, 3, and 4. Once i becomes 5, the break statement is triggered, and the loop ends without printing any further values.
Output
0
1
2
3
4
2. Using break in a while loop
using System;
class Program
{
static void Main()
{
int i = 0;
while (i < 10)
{
if (i == 7)
{
break;
}
Console.WriteLine(i);
i++;
}
}
}
Explanation
This code uses a while loop to iterate as long as the variable i is less than 10. Inside the loop, there is an if statement that checks if the current value of i is equal to 7. If it is, the break statement is executed, causing the loop to terminate immediately. The loop starts with i initialized as 0. It then prints the value of i, increments i by 1, and repeats the process as long as i remains less than 10 and not equal to 7. Therefore, the loop will iterate and print the values 0, 1, 2, 3, 4, 5, and 6. Once i becomes 7, the break statement is triggered, and the loop ends without printing 7 or any further values
Output
0
1
2
3
4
5
6
2. Continue Statement in C#
In addition to the break statement, C# also provides the continue statement, which allows developers to skip the remaining code within a loop iteration and move on to the next iteration. The continue statement in C# is particularly useful when you want to skip certain iterations based on a specific condition.
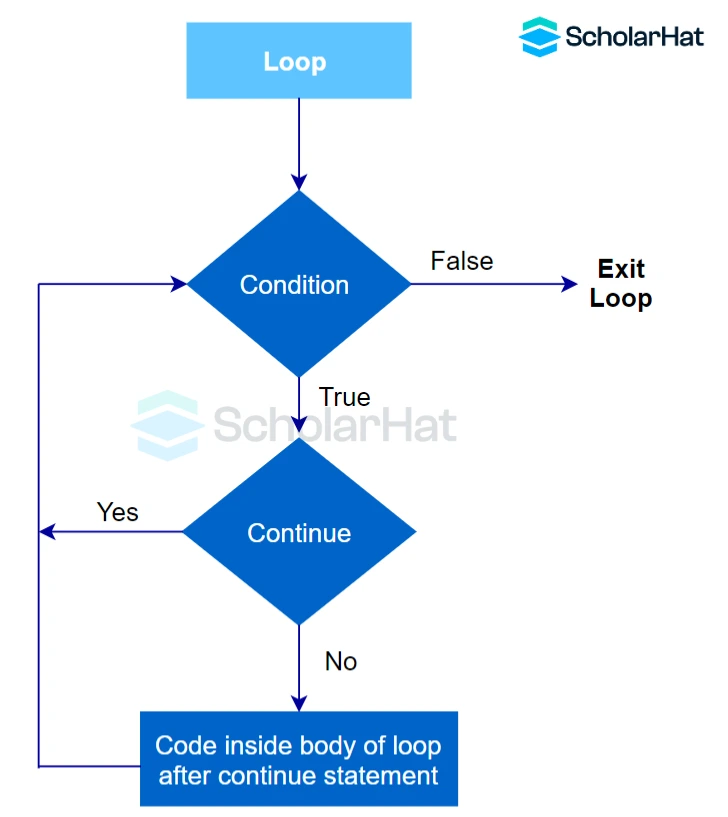
Examples of using the continue statement in loops
Here are a few examples of how the continue statement can be used in different loop scenarios:
1. Using continue in a for loop
using System;
class Program
{
static void Main()
{
for (int i = 0; i < 10; i++)
{
if (i % 2 == 0)
{
continue;
}
Console.WriteLine(i);
}
}
}
Explanation
This code in the C# Editor prints the odd numbers from 0 to 9 (inclusive) by skipping the even numbers using the continue statement in a loop.
Output
1
3
5
7
9
2. Using continue in a while loop
using System;
class Program
{
static void Main()
{
int i = 0;
while (i < 10)
{
i++;
if (i % 3 == 0)
{
continue;
}
Console.WriteLine(i);
}
}
}
Explanation
This code initializes i to 0 and then enters a while loop. In each iteration, i is incremented by 1. If i is divisible by 3, the loop skips to the next iteration using continue. Otherwise, it prints the value of i. The loop continues until it reaches 10.
Output
1
2
4
5
7
8
10
3. Goto Statement in C#
The goto statement in C# allows developers to transfer the control of the program to a labeled statement within the same method, or to a labeled statement within a different method in the same class. While the goto statement can be a powerful tool, it should be used with caution as it can make the code harder to understand and maintain.
Examples of using the goto statement in C#
Here are a few examples of how the goto statement can be used in different scenarios:
1. Breaking out of nested loops
using System;
class Program
{
static void Main()
{
for (int i = 0; i < 10; i++)
{
for (int j = 0; j < 10; j++)
{
if (i == 5 && j == 5)
{
goto end;
}
Console.WriteLine(i + ", " + j);
}
}
end:
Console.WriteLine("Loop exited");
}
}
Explanation
This code consists of nested for loops. It iterates through the values of i and j from 0 to 9. When i and j both equal 5, the code executes a goto statement, jumping to the end label and exiting both loops. If the condition is not met, it prints the values of i and j using Console.WriteLine(i + ", " + j);. After the loops or upon goto execution, "Loop exited" is printed to the console.
Output
0, 0
0, 1
0, 2
0, 3
0, 4
0, 5
0, 6
0, 7
0, 8
0, 9
1, 0
1, 1
1, 2
1, 3
1, 4
1, 5
1, 6
1, 7
1, 8
1, 9
2, 0
2, 1
2, 2
2, 3
2, 4
2, 5
2, 6
2, 7
2, 8
2, 9
3, 0
3, 1
3, 2
3, 3
3, 4
3, 5
3, 6
3, 7
3, 8
3, 9
4, 0
4, 1
4, 2
4, 3
4, 4
4, 5
4, 6
4, 7
4, 8
4, 9
5, 0
5, 1
5, 2
5, 3
5, 4
Loop exited
2. Error handling
using System;
class Program
{
static void Main()
{
try
{
// Some code that might throw an exception
}
catch (Exception ex)
{
Console.WriteLine("An error occurred: " + ex.Message);
goto error;
}
Console.WriteLine("Code executed successfully");
error:
Console.WriteLine("Error handling code executed");
}
}
Explanation
This code in the C# Online Compiler demonstrates exception handling using a try-catch block. The try block contains the code that might throw an exception. If an exception occurs, the catch block is executed. It prints an error message with the exception message. Then, using the goto statement, it jumps to the error label. Regardless of whether an exception occurred or not, the code after the catch block is executed, printing "Code executed successfully". Finally, the code reaches the error label and executes the error handling code, printing "Error handling code executed".
Output
Case 1: Exception is thrown
An error occurred:
Error handling code executed
Case 2: No exception is thrown
Code executed successfully
Error handling code executed
3. Simplifying complex control flow
using System;
class Program
{
static void Main()
{
int i = 0;
start:
if (i < 10)
{
Console.WriteLine(i);
i++;
goto start;
}
}
}
Explanation
This code demonstrates a loop using the goto statement. It initializes i to 0 and labels it as start. It then enters a conditional block that checks if i is less than 10. If true, it prints the value of i, increments it by 1, and jumps back to the start label using goto. This process repeats until i is no longer less than 10. Essentially, it prints the numbers from 0 to 9 and utilizes the goto statement for looping behavior.
Output
0
1
2
3
4
5
6
7
8
9
4. Return statement in C#
In C#, the "return" statement is used within a method to explicitly specify the value that the method should return to the caller. It can return a value of a specified data type, or it can be used without a value in methods with a return type of "void." Once a "return" statement is executed, it immediately exits the method, and control is returned to the caller with the specified value, if any.
Examples of using the return statement in C#
Returning an Integer
using System;
public class Program
{
public int Add(int a, int b)
{
int result = a + b;
return result;
}
public static void Main()
{
// Creating an instance of Program
Program programInstance = new Program();
// Calling the method using the instance
int sum = programInstance.Add(5, 3);
Console.WriteLine("Sum is: " + sum);
}
}
Explanation
This C# code in the C# Editor defines a method named Add that takes two integer parameters, a and b, calculates their sum, and returns the result. When called with values 5 and 3, it assigns the sum (8) to the variable sum and prints "Sum is: 8" to the console.
Output
Sum is: 8
Returning a String
using System;
public class Program
{
public string Greet(string name)
{
string greeting = "Hello, " + name + "!";
return greeting;
}
public static void Main()
{
// Calling the method
string message = Greet("Scholars");
Console.WriteLine(message);
}
}
Explanation
This C# code defines a method called Greet that takes a string parameter name, constructs a greeting message by appending the name to "Hello," and returns the resulting string. When the method is called with the argument "Scholars," it prints "Hello, Scholars!" to the console using Console.WriteLine.
Output
Hello, Scholars!
5. Throw statement in C#
By using the new keyword manually, this is utilized to construct an object of any legitimate exception class. Every legitimate exception must derive from the Exception class.
Example
using System;
public class Program
{
public static string Greet(string name)
{
string greeting = "Hello, " + name + "!";
return greeting;
}
public static void Main()
{
// Calling the method
string message = Greet("Scholars");
Console.WriteLine(message);
}
}
Explanation
This C# program in the C# Playground demonstrates the use of the throw keyword. It defines a class Scholars with a method displaysubject that throws a NullReferenceException if the input string is null. In the Main method, it calls displaysubject with a null argument and catches the exception, printing the exception message if an exception occurs.
Output
Exception Message
Conclusion
In conclusion, jump statements in C# offer powerful control flow mechanisms, enhancing program flexibility. Whether it's breaking out of loops, skipping iterations, or transferring control within methods, understanding and using break, continue, goto and return statements judiciously can significantly improve code readability, efficiency, and functionality. Also, Consider our C# Programming Course for a better understanding of all C# concepts.
FAQs
Q1. What are the 4 jump statements in C?
Q2. What is a jump statement in C#?
Q3. What is continue vs break vs return in C#?
Take our free csharp skill challenge to evaluate your skill

In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.