30
MayjQuery Attribute Manipulation
jQuery provides the methods which are used to manipulate the attribute in HTML element. In this article, I’m going to share the frequently used jQuery methods used to manipulate the attribute of an HTML element.
jQuery methods to manipulate the HTML element attribute
Read More - jQuery Interview Questions and Answers
addClass()
This method basically used to add the CSS class for the HTML element .
$(selector).addClass([class_name])HTML
<h2> jQuery Attribute Manipulation</h2> <p id="paraText"> Hello! Welcome to jQuery World</p>CSS
.my-color{ color:orange; } .my-font{ font-style:italic; }JavaScript
$(document).ready(function () { $('#paraText').addClass('my-color'); });Browser
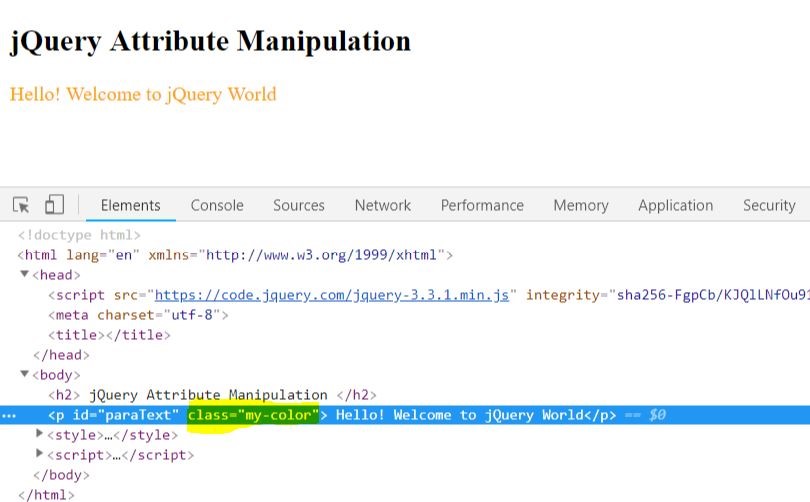
From the inspect element it is obvious the CSS class is added to the
element using the addClass().We can also concatenate the CSS Class using addClass().
JavaScript$(document).ready(function () { $('#paraText').addClass('my-color my-font'); });Browser
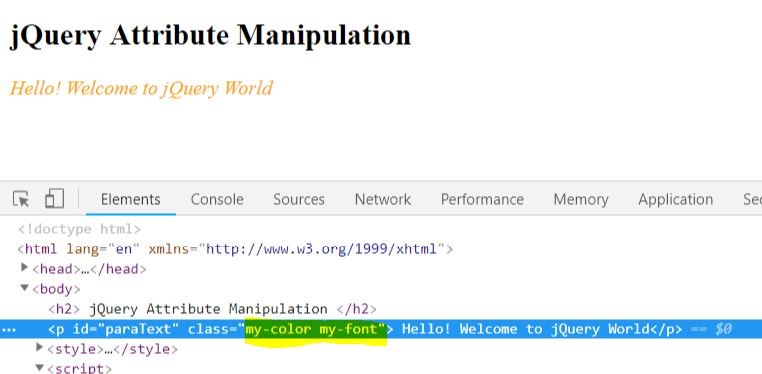
It will return undefined if the doesn’t matches the element
HTML<h2> jQuery Attribute Manipulation </h2> <p id="paraText">Hello! Welcome to jQuery World</p>JavaScript
$(document).ready(function () { $('#paraText').addClass('my-color1'); });
attr ()
It is used to get the attribute value from the matched element, if there is a set of matched elements it will return the attribute value of first element or else it will return undefined.
JavaScriptvar attrValue = $('#paraText').attr('id');Browser
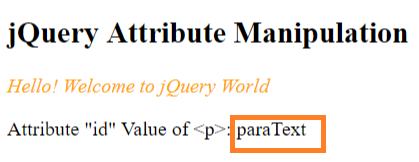
We can also set an attribute to the matched first element
JavaScript$('#paraText').attr('align', 'justify');
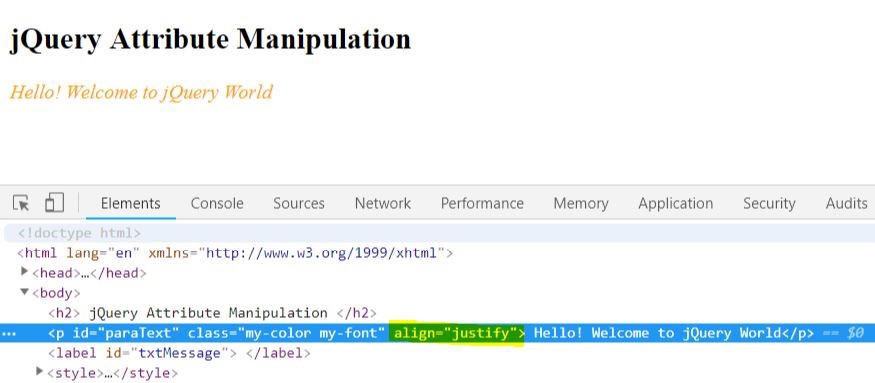
From the above image it is obvious the ‘align’ attribute is added to the <p> Note: If there is set of matched elements the attribute will be add to the first element, this rule is applied for all attribute manipulation methods check the demo below
HTML<h2> jQuery Attribute Manipulation </h2> <p id="paraText"> Hello! Welcome to jQuery World </p> <p id="paraText"> Hello! Welcome to jQuery World </p> <p id="paraText"> Hello! Welcome to jQuery World </p> <label id="txtMessage"> </label>JavaScript
$('#paraText').attr('align', 'justify');Browser
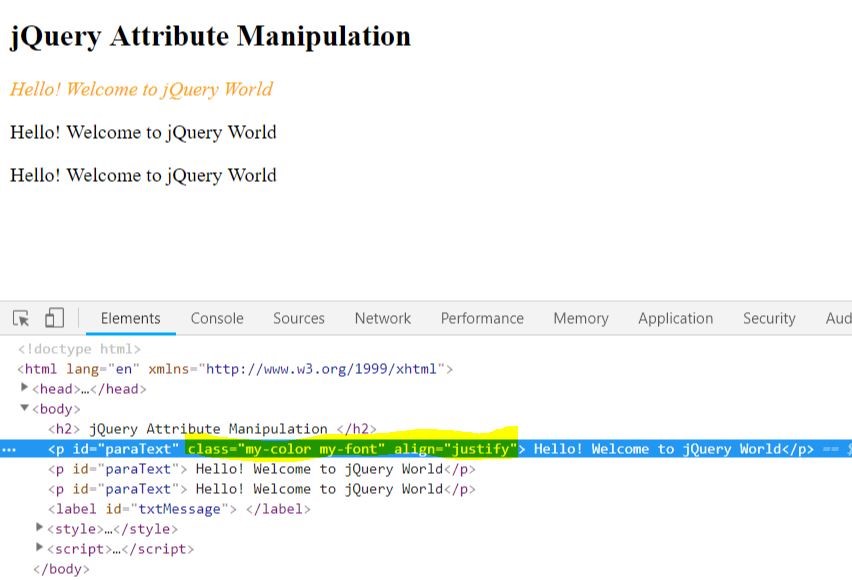
The attribute is added only to the first element even though the selector gets a set of elements. It will return undefined if the attribute is not found in the matched element
HTML<h2> jQuery Attribute Manipulation </h2> <p id="paraText"> Hello! Welcome to jQuery World</p>JavaScript
var attrValue = $('#paraText').attr('class');Output
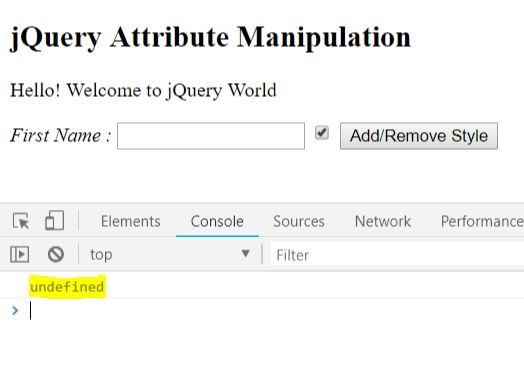
Since the class attribute is not available in matched element, it returns as undefined.
hasClass()
It is used to check whether the matched element holds the CSS class name which is overloaded with it. If the matched element contains the class it returns as true or else false
HTML<h2> jQuery Attribute Manipulation </h2> <p id="paraText"> Hello! Welcome to jQuery World</p> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" /> <label id="txtMessage"> </label>JavaScript
if ($("#lblFirstName").hasClass('my-font')) { $('#txtInput').addClass('my-bg-color'); }
If the label contains a class ‘my-font’, the CSS class is added to the input text box.
Browser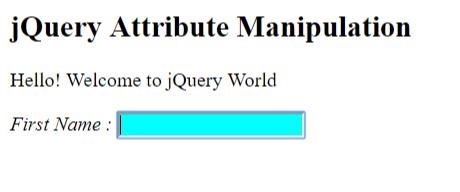
html ()
It is used to fetch the HTML content of the first matched element.
JavaScriptconsole.log("HTML ofBrowser- " + $('#paraText').html());
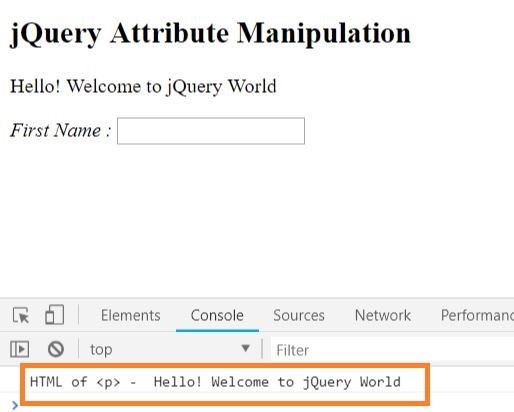
From above image you can observe the HTML content which is rendered in browser is returned from the html().
prop ()
It is used to set and get the property for first HTML element from the set of matched element, there is a certain difference between the prop() and attr().For example, the selectedIndex, nodeName, tagName and many more properties which are associated with HTML Element should be retrieved and set using prop method. These are not belonging to attribute it’s only a property.
HTML<input id="chk" type="checkbox" checked="checked">JavaScript
console.log("check box property value of checked attribute - " + $('#chk').prop('checked'));
This will give you the checked property value
Browser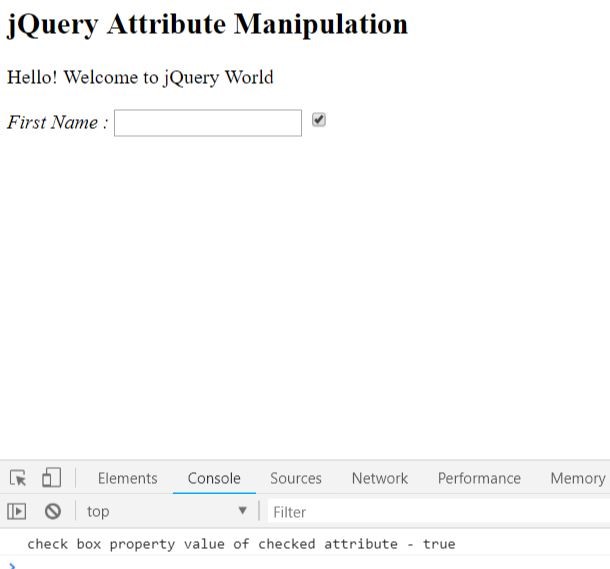
The checked property returns the value as “true”, In case of attr() it will return as “checked” Set the property value using prop()
HTML<input id="chk" type="checkbox" checked="checked">JavaScript
$('#chk').prop('checked',false)Browser
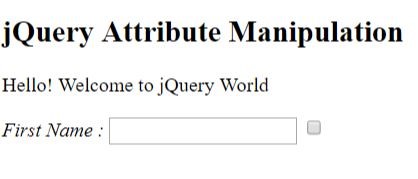
From the above figure you can notice the check box is unchecked now, because we set the checked property as false for checkbox.
removeAttr()
It is used to remove the attribute of the first element from the set of matched HTML element.
HTML<label id="lblFirstName" class="my-font">First Name: </label><input type="text" id="txtInput" class="my-font" />JavaScript
$('#lblFirstName').removeAttr('class');Browser
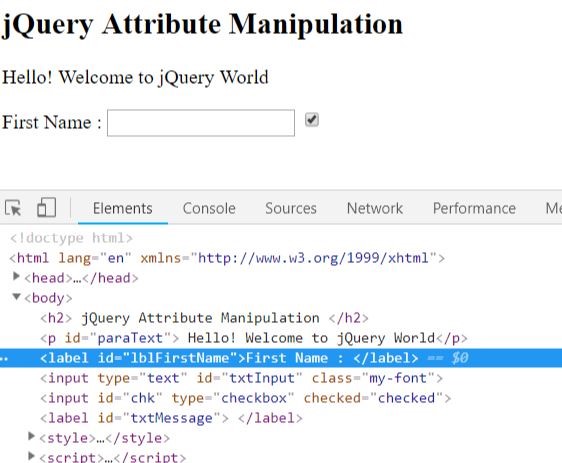
From the above figure you can notice the class attribute Is removed from <label>
removeProp()
It is used to remove the property set by prop() for the matched element Note: Don’t use removeProp() to remove checked, disabled or selected, because once it is removed it can’t be added again, better you can use the prop() to set the value as false
JavaScript$('#chk').prop("dummyProperty", "I'm dummy property"); console.log($('#chk').prop("dummyProperty")); $('#chk').removeProp("dummyProperty"); console.log("After removing the prop the value of dummyProperty - ", $('#chk').prop("dummyProperty"));Browser
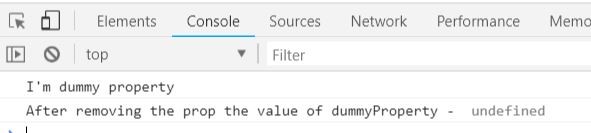
Once the property is removed, if you try to access it, the prop() will return “undefined”.
removeClass()
It is used to remove the CSS class of the first element from the matched set of elements.
HTML<input type="text" id="txtInput" class="my-font" />JavaScript
$('#txtInput').removeClass('my-font')Browser
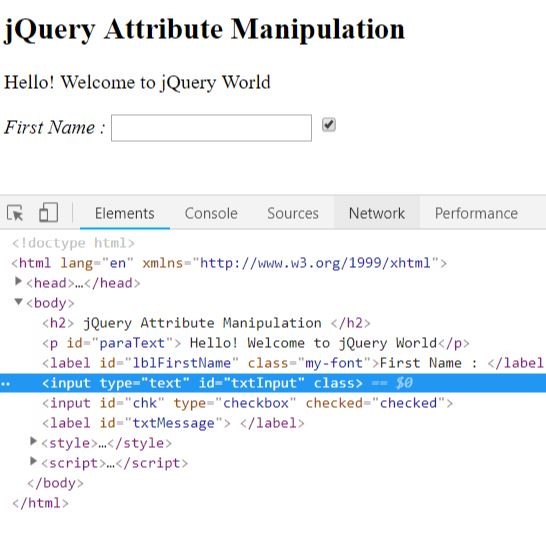
From the image you can observe the class attribute value is removed from the input element.
toggleClass ()
Basically, it is used to add or remove the classes for the matched element.
HTML<h2> jQuery Attribute Manipulation </h2> <p id="paraText"> Hello! Welcome to jQuery World</p> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" /> <input id="chk" type="checkbox" checked="checked"> <label id="txtMessage"> </label> <button id="btn"> Add/Remove Style </button>JavaScript
$("#btn").on("click", function () { $("#lblFirstName").toggleClass('my-font'); })Browser
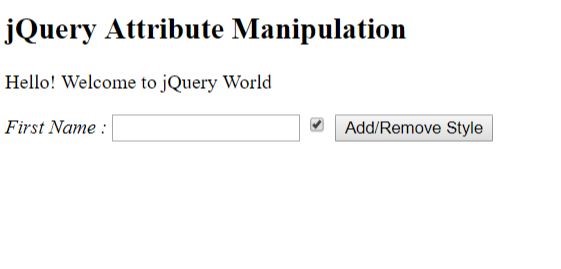
Before Button Click
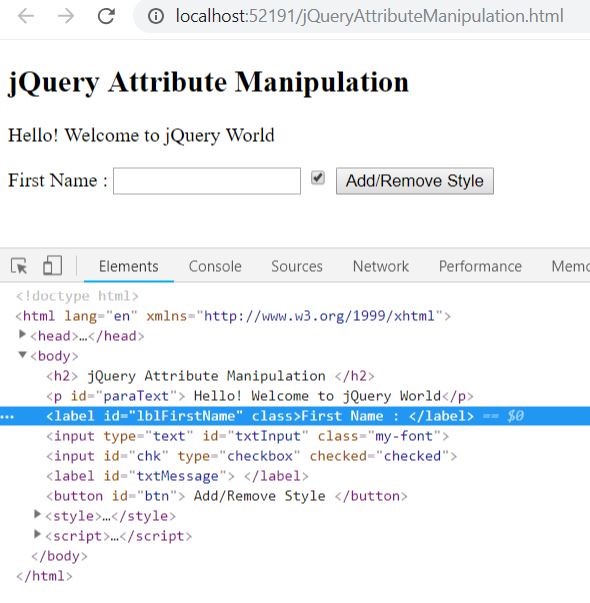
After button Click
After button click you can notice the CSS class attribute value is removed for <label>.
val()
It is used to get the value of the matched form element like textbox, radio, checkbox, textarea, drop down list and many more.
HTML<h2> jQuery Attribute Manipulation </h2> <p id="paraText"> Hello! Welcome to jQuery World</p> <label id="lblFirstName" class="my-font">First Name : </label><input type="text" id="txtInput" class="my-font" /> <input id="chk" type="checkbox" checked="checked"> <label id="txtMessage"> </label> <button id="btnTxtVal">Get Value</button>JavaScript
$('#btnTxtVal').on('click', function () { alert($('#txtInput').val()); })Browser
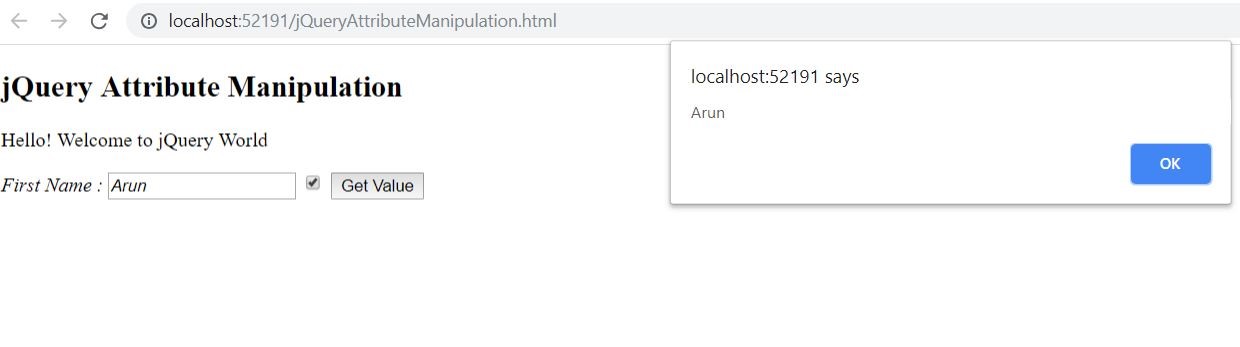
When the button is clicked the val() returns the entered value in the textbox and displayed in alert box Even you can set the value to the text box using the val().
JavaScript$('#txtInput').val("Arun Kumar");Browser
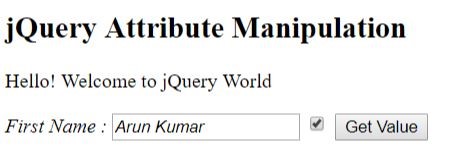
From the above figure you can observe the value “Arun Kumar” overloaded to the val() which is set to the text box when the document in loaded.
Summary
We have seen how to manipulate the HTML element attributes using the jQuery manipulation methods, and the note is if there is a set of matched elements, the jQuery manipulation function will manipulate only the first element and finally don’t use the removeProp() to remove checked, disabled or selected property from the element.