25
AprEverything About React Functional Components
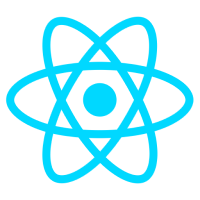
ReactJS Course
React Functional Components: An Overview
React Functional Components are the common components widely used in React. They are JavaScript/ES6 functions. In this section of React Tutorial, we will try to understand What are React Functional Components? and What are the different types of Functional Components in React. To have deeper knowledge of React and its concepts, consider enrolling in our React JS Certification Course.
What are React Functional Components
- React Function Components is another term used for React Functional Components.
- You can easily create a functional component to React after writing a JavaScript function. Every time, these functions may not receive data in the form of parameters. The return value in the functional components is the JSX code useful to render to the DOM tree.
- You can use the React functional components as the status quo of writing contemporary React applications. There were several React Component Types prevalent in the past. However, with the inception of React Hooks, it is now possible to write your entire application through just functions as React components.
- A functional component in React always begins with a capital letter. Moreover, it takes props as a parameter if needed.
An example of a valid functional component in React is:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
Now let’s go through some of the commonly used Functional Components in React:
Props -React Functional Component:
- Props are read-only and are received from a parent component. In React, props are widely utilized to pass information from one component to another. Moreover, they are passed down the component tree:
- It is important to know what the components in React render. Whenever rendering a component, you can pass props to the component, in form of HTML attributes. Subsequently, in the Function Component, the props object is presented as an argument within the function signature.
- Props always come as objects, and frequently, you have to extract the info from the props anyway. Therefore, the JavaScript object destructuring proves to be useful. Furthermore, you can directly incorporate it within the function signature for the props object. It is vital to be familiar with Props when you plan to Learn Reactjs Step By Step.
import React from 'react';
const Greeting = (props) => (
<div>
<h1>Hello, {props.name}!</h1>
<p>{props.message}</p>
</div>
);
export default Greeting;
Read More - React Interview Questions And Answers
React Arrow Function Component:
- The inception of JavaScript ES6 give rise inclusion of new coding concepts in JavaScript and React. For example, a JavaScript function can be represented as lambda (arrow function). Therefore, a Function Component is occasionally regarded as Arrow Function Components (or Lambda Function Component).
- Whenever using arrow functions for the React components, there are no changes for the props. They can still be accessed as arguments just like before. Alternatively, Arrow is a React Function Component in which the ES6 Functions are represented as arrows instead of ES5 functions. Generally, the ES5 functions are the default manner of representing functions in JS.
- Arrow function is quite handy when you want to create the function without being used as a constructive function because the arrow function does not have its own "this" context, unlike normal function.
import React from 'react';
const Greeting = ({ name, message }) => (
<div>
<h1>Hello, {name}!</h1>
<p>{message}</p>
</div>
);
export default Greeting;
Stateless React Function Component:
- The components discussed above can be called Stateless functional components. They simply receive input in form of props and return output in form of JSX: (props) => JSX.
- If the input is only available as props, it defines the rendered output. Such types of components don’t deal with state and don’t show any side effects. You will not find a side effect like retrieving the browser's local storage.
- The reason why they are called the Functional Stateless Components is they are stateless and denoted by a function. But, with the React Hooks, the state is now available in the Function Components. The same is discussed below.
import React from 'react';
const Greeting = (props) => {
return (
<div>
<h1>Hello, {props.name}!</h1>
<p>{props.message}</p>
</div>
);
};
export default Greeting;
Read More - React JS Roadmap For Beginners
React Function Component: State
- It is possible to use state and the side-effects in Function Components with the React Hooks. To understand it, can assume that there is the transfer of all logic to our other Function Component and there is no passing of props to it.
- Without a state, it is impossible to interact with the application. Also, without a state, you cannot change the greeting variable. With the use of React Hooks, the State React Functional Component makes React interactive and fascinating.
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<h1>Counter: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={() => setCount(count - 1)}>Decrement</button>
</div>
);
};
export default Counter;
Event Handler -React Function Component:
- One of the most common event handlers in React isonChange. It is used in the input field to keep you notified whenever the internal value of the input field changes.
- Some other prevalent event handlers are onMouseDown, onClick, and onBlur.
- To define the function within the component, you can use an arrow function or class method. It is allowed to either create or add multiple Functional Components as you like to either work as explicit event handlers or summarize other business logic.
- The app builds React Virtual DOM which in turn builds the DOM. As a response, the DOM delivers events to React Virtual DOM which in turn delivers events to the app. So, the event handling is smooth and perfect.
import React from 'react';
const Button = () => {
const handleClick = () => {
console.log('Button clicked!');
};
return (
<button onClick={handleClick}>
Click me
</button>
);
};
export default Button;
Callback Function -React Function Component:
- There are no props passed to Child Function Component. The question that arises is whether it is allowed to pass a function to a component as a prop too or not. The answer is it can be managed to call a component function from the outside. This can be done by passing a function to a Child Component and then handling everything that takes place in the Parent Component.
- Also, you can execute certain functions between the Child Component for the onChangeHeadline function. This helps you to add extra functionality within the Child Component. In this way, you can call a Child Component's function directly from a parent component.
- One thing to be considered while passing props to children and their sub-child is that there are some possibilities to generate a "props drilling" situation and to avoid this, we can make use of state management systems such as Redux, Context API and use the single state object into multiple components without worrying props drilling.
import React from 'react';
const ParentComponent = () => {
const callbackFunction = (message) => console.log(message);
return <ChildComponent callback={callbackFunction} />;
};
const ChildComponent = ({ callback }) => (
<button onClick={() => callback('Callback from ChildComponent')}>
Call Callback
</button>
);
export default ParentComponent;
Lifecycle -React Function Component:
- For those who earlier used the React Class Components, they may be familiar with the lifecycle methods namely componentWillUnmount, componentDidMount, and shouldComponentUpdate. These methods are not accessible in the Function Components, so it is now interesting to see how they can be implemented.
- Keep in mind that there is no constructor in a Function Component. Typically, the constructor is used within a React Class Component for assigning the initial state. It is not required in a Function Component since you can assign the initial state with the useState hook as well as set up functions inside the Function Component for business logic.
- With the recent version of React, React Hooks was introduced in version 16.8 and by using it, we can create a stateful component without declaring a class or a class-based syntax. The aim was to introduce all the benefits of using class components in function components such as life cycle hooks, state so that we can take advantage of using function components as well.
import React, { useState, useEffect } from 'react';
const LifecycleComponent = () => {
const [count, setCount] = useState(0);
useEffect(() => {
console.log('Component mounted');
return () => console.log('Component unmounted');
}, []);
useEffect(() => {
console.log('Count updated:', count);
}, [count]);
return (
<div>
<h1>Lifecycle Component</h1>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment Count</button>
</div>
);
};
export default LifecycleComponent;
Export and Import -React Function Component:
- Typically, you will observe Export and Import functions separately in their files. Because React Components are classes or functions, you can utilize the standard import & export statements presented by JavaScript. It is possible to define and export a component in one file and then import it into another file.
- If the component name is not significant to you, you can let it stay as Anonymous Function Component while using a default export within the React Function Component.
- The same applied to third-party libraries where we can import multiple components in a single line coming from the library itself, so syntax and lines of codes are being optimized.
Pure React Function Component:
- React Class Components provide you with the ability to determine whether a component needs to be rerendered or not. The same was made possible with the PureComponent or shouldComponentUpdate by avoiding rerenders. As a result, they prevent performance congestion.
- With the Pure React Function Component, whenever you type anything in the input field, the App component would update its state, rerenders, and also re-renders the Count component.
TypeScript -React Function Component:
- When you are searching for a type system for your React application, you can try out TypeScript for React Components.
- TypeScript offers plenty of benefits to a developer. For example, it provides IDE support and can make the code base robust.
PropTypes -React Function Component:
- After you have defined your component, just assign it PropTypes to confirm the incoming props of a particular component. You can use the PropTypes for Function Components and React Class Components identically.
- The proptypes need to be defined before using it validates each proper against its type so it is recommended to assign the respective types of props to avoid values assigned to it.
Summary
Through this article, you learned about different types of Functional Components in React and how they can be used to create React Applications in a more efficient manner. To have a deeper understanding of React and React Development, you should definitely try our React JS Certification Training.
FAQs
Q1. What is a functional component in React?
Q2. Why use functional components React?
Q3. What is the difference between props and state?
Take our free react skill challenge to evaluate your skill

In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.