11
JulAccess Modifiers in TypeScript Explained
13 Dec 2024
Intermediate
73.6K Views
13 min read
TypeScript Access Modifiers
TypeScript access modifiers are keywords that affect the visibility of class members such as properties and methods. They decide who has access to these members and where they can get it. Consider access modifiers in typescript as a means to safeguard certain data or functionality, such as closing some doors while leaving others open.In this Typescript tutorial, We will explore public, private, and protected access modifiers in Typescript, as well as a specific read-only modifier that assures things remain as they were once you set them. It's all about controlling how your code interacts with itself and with other sections of your app, so everything is more structured and secure. So let's go.
Table of Content |
Types of Access Modifiers |
|
Access Modifiers in Action: Code Examples |
|
Best Practices for Using Access Modifiers |
Conclusion |
Types of Access Modifiers in Typescript
There are 3 Types of Access Modifiers in Typescript:
- Public
- Private
- Protected
Access Modifiers in Action: Code Examples
In the "Access Modifiers in Action" section, you'll see how public, private, protected, and readonly modifiers work through real-world TypeScript code examples. These examples demonstrate how access modifiers control the visibility and accessibility of class properties and methods, enabling better encapsulation and structure within your applications.
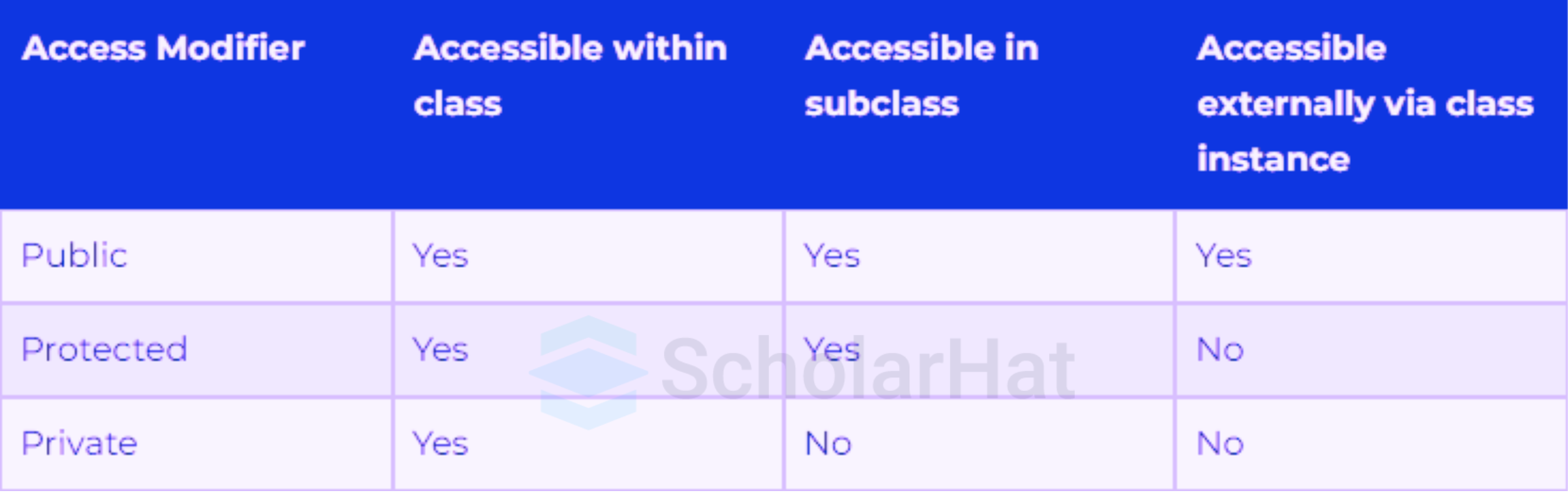
1. Public Access Modifier in Typescript
The public access modifier in typescript has two big benefits.
Example of Public Access Modifier in Typescript
class Person {
public name: string;
constructor(name: string) {
this.name = name;
}
public greet(): void {
console.log(`Hello,Welcome to Scholarhat ${this.name}.`);
}
}
const person = new Person("Buddy");
console.log(person.name); // Accessible
person.greet(); // Accessible
Output
Buddy
Hello,Welcome to Scholarhat Buddy.
Explanation
In the Person class, the name is declared public, making it available outside of the class. Using dot notation, we may get the name of a Person object (person).
2. Private Access Modifier in Typescript
The private access modifier in typescript limits a class member's visibility to the class itself. It promotes encapsulation and data protection by preventing direct access from outside the class. In short, Private members are inaccessible outside the classroom, maintaining encapsulation.Example of Private Access Modifier in Typescript
class BankAccount {
private balance: number;
constructor(initialBalance: number) {
this.balance = initialBalance;
}
public deposit(amount: number): void {
this.balance += amount;
console.log(`Deposited ${amount}. New balance: ${this.balance}.`);
}
private logTransaction(): void {
// Logging logic
}
}
const account = new BankAccount(1000);
account.deposit(500); // Accessible
// account.balance; // Error: Property 'balance' is private and only accessible within class 'BankAccount'.
// account.logTransaction(); // Error: Property 'logTransaction' is private and only accessible within class 'BankAccount'.
Output
Deposited 500. New balance: 1500.
Explanation
In this TypeScript example, the class BankAccount has a private balance property, meaning it can only be accessed within the class itself, not from outside. The deposit method is public, allowing it to be called on instances of the class (like account. deposit(500)), which modifies the balance and displays the new value. The logTransaction method is marked as private, preventing it from being accessed outside the class. This example demonstrates the use of access modifiers (private and public) to control access to class members, ensuring proper encapsulation of sensitive data (like balance) and internal logic.
3. Protected Access Modifier in Typescript
Members can be accessed within the class and by subclasses, but not directly from outside the class hierarchy, thanks to the protected access modifier. It encourages inheritance as well as regulated access to shared functions.Example of Protected Access Modifier in Typescript
class Employee {
protected employees: string;
constructor(employees: string) {
this.employees = employees;
}
protected employeeName(): void {
console.log("Employees: Pradnya, Aman, Shailesh.");
}
}
class Company extends Employee {
public name: string;
constructor(name: string) {
super("Company");
this.name = name;
}
public companyName(): void {
console.log(`${this.name} says: Welcome to Scholarhat!`);
this.employeeName(); // Accessible within subclass
}
}
// Create an instance of the Company class
const companyInstance = new Company("Buddy");
companyInstance.companyName(); // Accessible
// Uncommenting the following will raise errors:
// console.log(companyInstance.employees); // Error: Property 'employees' is protected and only accessible within class 'Employee' and its subclasses.
// companyInstance.employeeName(); // Error: Property 'employeeName' is protected and only accessible within class 'Employee' and its subclasses.
Output
Buddy says: Welcome to Scholarhat!
Employees: Pradnya, Aman, Shailesh.
Explanation
In this corrected TypeScript code, the Employee class has a protected employeeName() method and a protected employees property, both of which are accessible only within the class and its subclass, Company. The Company class can call employeeName() because it's within the inheritance chain, but external code cannot access it. The public companyName() method demonstrates how the subclass interacts with the protected members while keeping them inaccessible from outside.
Read More - Typescript Interview Questions And Answers |
Read-only Access Modifier in Typescript
After initialization, the read-only access modifier marks a property as immutable, enabling it to be read but not directly updated. This improves data security and makes state management easier.Example of Read-only Access Modifier in Typescript
class Car {
public readonly model: string;
private readonly vin: string;
constructor(model: string, vin: string) {
this.model = model;
this.vin = vin;
}
public getVin(): string {
return this.vin;
}
// Attempting to modify a readonly property will cause a compile-time error
// public updateModel(newModel: string): void {
// this.model = newModel; // Error
// }
}
const car = new Car("Tesla Model S", "5YJSA1E26HF000337");
console.log(car.model); // Accessible
console.log(car.getVin()); // Accessible
// car.model = "Tesla Model 3"; // Error: Cannot assign to 'model' because it is a read-only property.
// car.vin = "NEWVIN1234567890"; // Error: Cannot assign to 'vin' because it is a read-only property.
Output
Tesla Model S
5YJSA1E26HF000337
Best Practices for Using Access Modifiers
- 1. Encapsulate Data: Use private or protected modifiers to encapsulate internal class data and expose only what is required via public methods.
- 2. Minimize Public Surface Area: Limit the number of public members to lower the complexity of the class's interface.
- 3. Use Readonly for Constants: Add the readonly modification to attributes that should remain constant after initialization to improve immutability and predictability.
- 4. When building classes designed for inheritance, use protected rather than public to expose members that subclasses require without revealing them to the wider world.
- 5. Consistent Modifier Usage: To increase readability and maintainability, apply access modifiers consistently throughout your program.
- 6. Use TypeScript's compile-time checks to implement access controls and detect potential issues early.
Summary
Access modifiers in TypeScript are essential for establishing encapsulation, a core idea in object-oriented programming. They assist regulate class members' visibility and accessibility, resulting in more secure, maintainable, and robust programs. Developers may improve code quality, collaborate more easily, and scale huge projects by understanding and effectively implementing public, private, protected, and readonly modifiers.FAQs
There are four sorts of access modifiers in Typescript: public, private, protected, and read-only.
Private, protected, and public are the three access modifiers for class properties and methods in TypeScript.
Members who have been designated as public can be accessed from anywhere within or outside of the class. Members marked as private cannot be accessible from outside the class.
TypeScript provides three types of access modifiers: public, private, and protected. Members (properties and methods) of the TypeScript class are public by default, therefore you don't need to precede members with the public keyword.
The term protected denotes that the method or property is only accessible within the class or any class that extends it, but not externally. Finally, if the value of a readonly property is altered after its first assignment in the class constructor, the TypeScript compiler will generate an error.
Take our Typescript skill challenge to evaluate yourself!

In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.