23
MayDifference between c sharp generics and Collections with example
Generics and Collections in C#: An Overview
Generics and Generic collections are the most crucial concepts in C#.NET. So many developers feel that both are very complex, but after reading this article you will feel easy and comfortable using this Generics and Generic collection. In this C# Tutorial, we will explore more about Generics and Collections which will include c# generic list, c sharp generics, the difference between generic collections and non-generic collections, collections vs generics, the advantage of generics over collections with an example, generic and non-generic collections.
To gain a deeper understanding of Generics and Collections and other essential C# concepts, you can enroll in a C Sharp Online Course Free. This course will provide you with hands-on experience and practical knowledge to master these topics and become proficient in C#.
What are C# Generics?
- Generics are a powerful tool that can be used to code re-usability.
- Generics provides the type-safe code with re-usability like an algorithm.
- In algorithms such as sorting, searching, comparing, etc. you don’t specify what data type(s) the algorithm operates on.
- The algorithm can be operated with any type of data.
- In the same way, Generics operate, you can provide different data types to Generics. For example, a sorting algorithm can operate on integer type, decimal type, string type, DateTime type, etc.
Syntax Of Generics:
Generics are denoted using the <> symbol. The following code in the C# Compiler declares a generic method called Student.
public static void Student<T>(T value)
{
Console.WriteLine(value);}
Generics Example:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Generics
{
class Program
{
static void Main(string[] args)
{
Check < int > obj1 = new Check < int > ();
bool intResult = obj1.Compare(22, 33);
Check < string > obj2 = new Check < string > ();
bool strResult = obj2.Compare("Pragya", "Pragya");
Console.WriteLine("The Datatypes is Integer : {0}\nThe Datatypes is String : {1}", intResult, strResult);
Console.Read();
}
class Check < SecretDataType >
{
public bool Compare(SecretDataType var1, SecretDataType var2)
{
if (var1.Equals(var2))
{
return true;
}
else
{
return false;
}
}
}
}
}
Output
The Datatypes is Integer : False
The Datatypes is String : True
C# generic List(List<T>)
- The list is a generic type of collection.
- It allows to storage of only strongly typed objects, i.e., elements of the same data type.
- The size of the list changes dynamically based on application requirements, like adding or removing elements from the list.
- The list is the same as an ArrayList, but the only difference is ArrayList is a non-generic type of collection, so it will allow storing elements of different data types.
Generics List Example:
using System;
using System.Collections.Generic;
namespace Scholarhat
{
class Program
{
static void Main(string[] args)
{
// Creating and initializing list
List lst = new List();
lst.Add(11);
lst.Add(33);
lst.Add(55);
List lst2 = new List();
lst2.Add("Hi");
lst2.Add("Welcome");
lst2.Add("to");
lst2.Add("Scholarhat.com");
Console.WriteLine("List1 Count: " + lst.Count);
Console.WriteLine("List1 Capacity: " + lst.Capacity);
Console.WriteLine("List1 Elements");
// Accessing list elements
foreach (var item in lst)
{
Console.WriteLine(item);
}
Console.WriteLine("List2 Count: " + lst2.Count);
Console.WriteLine("List2 Capacity: " + lst2.Capacity);
Console.WriteLine("List2 Elements");
foreach (var item in lst2)
{
Console.WriteLine(item);
}
Console.ReadLine();
}
}
}
Output:
List1 Count: 3
List1 Capacity: 4
List1 Elements
11
33
55
List2 Count: 4
List2 Capacity: 4
List2 Elements
Hi
Welcome
to
Scholarhat.com
What are C# Collections?
- Collections are used to more effectively organize, store, and modify comparable data.
- By Adding, deleting, discovering, and inserting data into the collection we can achieve data manipulation.
- Collections support stacks, queues, lists, and hash tables.
- Most of the collection classes implement the same interfaces.
There are two types of collections in C#:
1. Generic Collections
2. Non-Generic Collections
Collections Example in C#:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace GenericCollectionSample
{
class Program
{
static void Main(string[] args)
{
List < int > listObj = new List < int > ();
listObj.Add(123);
listObj.Add(235);
listObj.Add(444);
Console.WriteLine("List Third Value: {0}", listObj[2]);
// Key based generic Collection (Dictionary)
Dictionary < int, string > objDic = new Dictionary < int, string > ();
objDic.Add(444, "Pragati Chauhan");
// Displaying Dictionary value using Key
Console.WriteLine("Dictionary Value: {0}", objDic[444]);
// Priority based Generic Collection (Stack)
Stack < int > objStack = new Stack < int > ();
objStack.Push(1);
objStack.Push(2);
objStack.Push(3);
// Display first value from Stack
Console.WriteLine("First Get Value from Stack: {0}", objStack.Pop());
// Priority based Generic Collection (Queues)
Queue < int > objQueue = new Queue < int > ();
objQueue.Enqueue(1);
objQueue.Enqueue(2);
objQueue.Enqueue(3);
// Display first value from Stack
Console.WriteLine("First Get Value from Queue: {0}", objQueue.Dequeue());
Console.WriteLine();
// Creating Employee records
Employee empObj1 = new Employee();
empObj1.ID = 1001;
empObj1.Name = "Surbhi";
empObj1.Address = "Pune";
Employee empObj2 = new Employee();
empObj2.ID = 1002;
empObj2.Name = "Sourav";
empObj2.Address = "Delhi";
// Creating generic List with Employee records
List < Employee > empListObj = new List < Employee > ();
empListObj.Add(empObj1);
empListObj.Add(empObj2);
// Displaying employee records from list collection
foreach(Employee emp in empListObj)
{
Console.WriteLine(emp.ID);
Console.WriteLine(emp.Name);
Console.WriteLine(emp.Address);
Console.WriteLine();
}
Console.Read();
}
public class Employee
{
public int ID;
public string Name;
public string Address;
}
}
}
Output
List Third Value: 444
Dictionary Value: Pragati Chauhan
First Get Value from Stack: 3
First Get Value from Queue: 1
1001
Surbhi
Pune
1002
Sourav
Delhi
Difference between generic and non-generic collections in C#
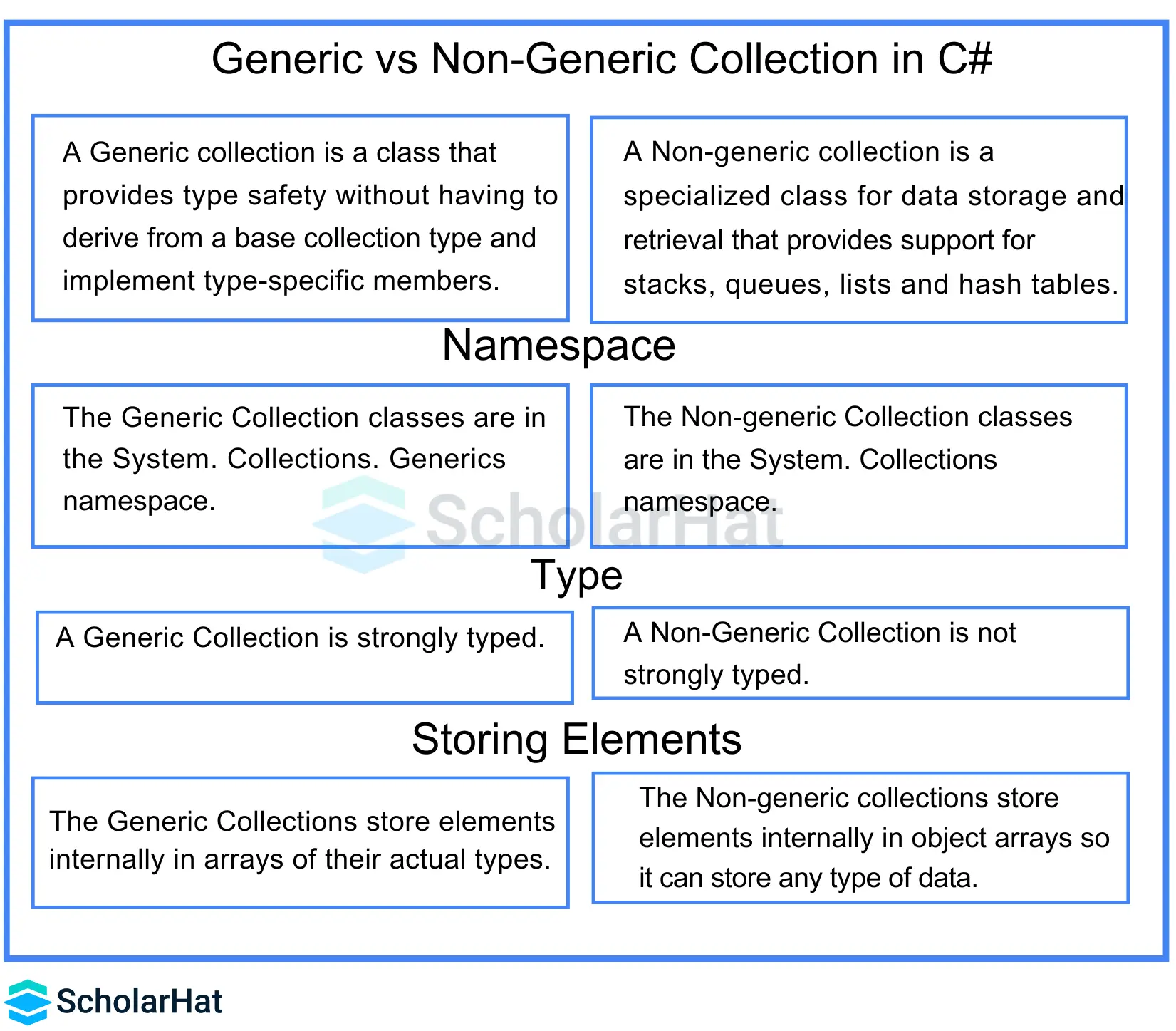
Advantage of generics over collections
1. Code Re-usability with Generics
Suppose, you are required to sort the integer and floating type numbers, then let's see how to do it in collections and generics.
How to do it using Collections
//Overloaded sort methods
private int[] Sort(int[] inputArray)
{
//Sort array
//and return sorted array
return inputArray;
}
private float[] Sort(float[] inputArray)
{
//Sort array
//and return sorted array
return inputArray;
}
How to do it using Generics
private T[] Sort(T[] inputArray)
{
//Sort array
//and return sorted array
return inputArray;
}
Here, T is short for Type and can be replaced with the Type defined in the C# language at runtime. So once we have this method, we can call it with different data types as follows and can see the beauty of Generics. In this way, Generics provide code re-usability.
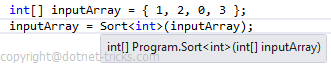

Now if you thinking you can make fool to the compiler by passing an integer array while it is asking for a float, you are wrong. The compiler will show the error at compile time like as:

Read More - C# Interview Questions And Answers
2. Type Safety with Generics
Suppose, you want to make a list of students, then let's see how to do it in collections and generics.
How to do it using Collections
In collections we can use ArrayList to store a list of Student objects like as:
class Student
{
public int RollNo{get; set;}
public string Name{get; set;}
}
//List of students
ArrayList studentList = new ArrayList();
Student objStudent = new Student();
objStudent.Name = "Rajat";
objStudent.RollNo = 1;
studentList.Add(objStudent);
objStudent = new Student();
objStudent.Name = "Sam";
objStudent.RollNo = 2;
studentList.Add(objStudent);
foreach (Object s in studentList)
{
//Type-casting. If s is anything other than a student
Student currentStudent = (Student)s;
Console.WriteLine("Roll # " + currentStudent.RollNo + " " + currentStudent.Name);
}
Problem with Collections
Suppose by mistake you have added a string value to ArrayList like as
studentList.Add("Generics"); //Fooling the compiler
Since ArrayList is a loosely typed collection it never ensures compile-time type checking. Hence the above statement will compile without error but it will throw an InvalidCastException at run time when you try to cast it to Student Type.
How to do it using Generics
In generics, we can use a generic List to store a list of Student objects like as:
List<Student> lstStudents = new List<Student>();
Student objStudent = new Student();
objStudent.Name = "Rajat";
objStudent.RollNo = 1;
lstStudents.Add(objStudent);
objStudent = new Student();
objStudent.Name = "Sam";
objStudent.RollNo = 2;
lstStudents.Add(objStudent);
//Looping through the list of students
foreach (Student currentSt in lstStudents)
{
//no need to type cast since compiler already knows that everything inside
//this list is a Student
Console.WriteLine("Roll # " + currentSt.RollNo + " " + currentSt.Name);
}
Using Generics
In the case of collections, you can make a fool to the compiler but in the case of generics, you can't make fool to the compiler as shown below. Hence Generics provide Type Safety.
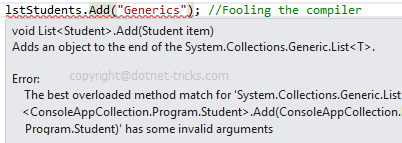
3. Cleaner Code with Generics
Since the compiler enforces type safety with Generics. Hence fetching data from Generics doesn't require typecasting which means your code is clean and easier to write and maintain.
4. Better Performance with Generics
As you have seen in the above example, at the time of fetching the data from the ArrayList collection we need to do type casting which causes performance degrades. But at the time of fetching the data from the generic List, we aren't required to do typecasting. In this way, Generics provide better performance than collections.
Read More Articles Related to csharpConclusion:
I hope you will enjoy the tips while programming with C#. I would like to have feedback from my blog readers. Your valuable feedback, questions, or comments about this article are always welcome. Also, Consider our C# Programming Course for a better understanding of all C# concepts
FAQs
Take our Csharp skill challenge to evaluate yourself!

In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.