Difference Between Finalize and Dispose Method
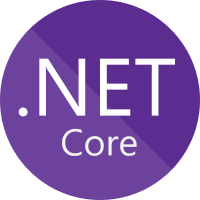
.NET Course
Finalize Vs. Dispose Method: An Overview
.NET Framework provides two methods Finalize and Dispose for releasing unmanaged resources like files, database connections, COM, etc. This article will help you to understand the difference between Finalize and Dispose methods. Check out our .NET Certification Training to get an edge over the competition.
Difference between Dispose & Finalize Method
Example of implementing the disposal method
public class MyClass : IDisposable
{
private bool disposed = false;
//Implement IDisposable.
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
if (!disposed)
{
if (disposing)
{
// TO DO: clean up managed objects
}
// TO DO: clean up unmanaged objects
disposed = true;
}
}
}
Example of implementing Finalize method
If you want to implement the Finalize method, it is recommended to use the Finalize and Dispose method together as shown below:
// Using Dispose and Finalize method together
public class MyClass : IDisposable
{
private bool disposed = false;
//Implement IDisposable.
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
if (!disposed)
{
if (disposing)
{
// TO DO: clean up managed objects
}
// TO DO: clean up unmanaged objects
disposed = true;
}
}
//At runtime C# destructor is automatically Converted to Finalize method
~MyClass()
{
Dispose(false);
}
}
Note
It is always recommended to use the Dispose method to clean unmanaged resources. You should not implement the Finalize method until it is extremely necessary.
At runtime C#, C++ destructors are automatically Converted to Finalize method. But in VB.NET you need to override the Finalize method since it does not support the destructor.
You should not implement a Finalize method for managed objects, because the garbage collector cleans up managed resources automatically.
A Dispose method should call the GC.SuppressFinalize() method for the object of a class that has a destructor because it has already done the work to clean up the object, then it is not necessary for the garbage collector to call the object's Finalize method.
Summary:
I hope you will enjoy the tips while programming with the .NET Framework. I would like to have feedback from my blog readers. Your valuable feedback, questions, or comments about this article are always welcome. We'll try to provide you Best .NET Training Course in our articles.