10
meiTop .NET Core Features
.NET Core Features: An Overview
.NET Core is the demand of today's digital era where robust and scalable web applications are increasing rapidly. ASP.NET Core is the open-source version of ASP.NET, that runs on macOS, Linux, and Windows. It includes the core features required to run a basic .NET Core app. Other features are provided as NuGet packages, which you can add to your application as needed.
In this .NET Tutorial, we will explore the Top Features of .NET Core in detail. To learn more about different core concepts of .NET, consider enrolling in our .NET Core Certification Training.
Read More: Top 50 .NET Core Interview Questions and Answers 2024
Top 15 .NET Core Features
A. Core Advantages for Development Efficiency:
1. Framework with Open Source Code
.NET Core is an open-source framework which makes it a cost-effective choice for web development. Due to its open-source nature, a diverse community of developers can work together and contribute to its growth and improvement.
2. Razor Pages
Razor Pages are used for combining the view and controller into a single file which helps in simplification of web development on a great level making the process of development easier.
Example of Razor pages in .NET Core:
using Microsoft.AspNetCore.Mvc.RazorPages;
using System;
public class IndexModel : PageModel
{
public string CurrentTime { get; set; }
public void OnGet()
{
CurrentTime = DateTime.Now.ToString("HH:mm:ss");
}
}
This code will print the current time onto the web page using the razor page.
3. Integrated MVC & Web API Tools
.NET Core also works by combining Model-View-Controller, also known as MVC for building user interfaces and Web API for creating RESTful services. It helps in improving the productivity of the developer as it maintains consistency in the framework for both UI and backend development.
B. Performance and Scalability:
4. Cross-Platform
.NET Core is cross-platform in nature. The developers can easily deploy applications on various platforms such as Windows, Linux, and MacOS and that too without affecting their performance. This means that the developers can choose the operating system as they wish.
5. High Performance
.NET Core also provides high performance with the help of Just-In-Time (JIT) compilation that helps in optimizing the execution of the code. It improves the performance and the application becomes more responsive.
6. Asynchronous Programming
Then, .NET Core also works on the Async/Await pattern which gives an advantage in handling long-running tasks. It reduces the chances of any blocking of the user interface which results in an enhanced overall user experience.
Example of Asynchronous Programming in .NET Core:
using System;
using System.Net.Http;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
await FetchDataAsync();
}
static async Task FetchDataAsync()
{
using (HttpClient httpClient = new HttpClient())
{
string url = "https://swapi.dev/api/people/1";
HttpResponseMessage response = await httpClient.GetAsync(url);
if (response.IsSuccessStatusCode)
{
var data = await response.Content.ReadAsAsync<Character>();
Console.WriteLine($"Name: {data.Name}");
Console.WriteLine($"Birth Year: {data.BirthYear}");
Console.WriteLine($"Height: {data.Height} cm");
}
else
{
Console.WriteLine($"Failed to fetch data. Status code: {response.StatusCode}");
}
}
}
public class Character
{
public string Name { get; set; }
public string BirthYear { get; set; }
public string Height { get; set; }
}
}
Here, we can see how to perform asynchronous programming using the 'async' and 'await' keywords.
Read More: .Net Developer Salary 2024
ASP.NET Core Development Features
A. Security Features:
7. Support for Multiple Environments and Development Modes
There are so many powerful environments that ASP.NET Core supports which proves to be a great aspect for developers wanting to implement secure development practices. This helps in separating the development, staging, and production environments.
8. Incorporated Dependency Injection
One of the major aspects you might have also heard of is the built-in feature of Dependency Injection in .NET Core, which is used for managing the object dependencies. It also helps in improving the code's testability and maintainability.
Example of Dependency Injection in .NET Core:
using System;
using Microsoft.Extensions.DependencyInjection;
public interface IGreetingService
{
string Greet(string name);
}
public class GreetingService : IGreetingService
{
public string Greet(string name) => $"Hello, {name}!";
}
public class Program
{
public static void Main()
{
var serviceProvider = new ServiceCollection()
.AddSingleton<IGreetingService, GreetingService>()
.BuildServiceProvider();
var greeter = serviceProvider.GetService<IGreetingService>();
Console.WriteLine(greeter.Greet("John"));
}
}
Here, we have defined an interface 'IGreetingService' with a 'Greet' method. 'Greeter' class will also depend on 'IGreetingService' which comes with constructor injection.
9. Cross-Site Request Forgery (CSRF) Protection
ASP.NET Core also provides a built-in protection feature that prevents commonly occurring web attacks like CSRF, also known as Cross-Site Request Forgery. It makes sure that the overall web applications are secure by default.
B. Enhanced User Experience Features:
10. Action Filters
There are action filters in ASP.NET Core. With the help of action filters, the developers can execute pre and post-processing logic for controller actions. This allows in reusing the code and organizing the common actions across controllers.
11. Output Caching Options
Developers can use Output Caching in .NET Core for caching the data or views that are frequently accessed making the application more scalable.
12. Globalization and Localization Features
ASP.NET Core provides great support for features that enhance globalization and localization. Such tools help in building applications that are for the international audience as well.
Advanced Features for Modern Development
13. Swagger OpenAPI
.NET Core helps in simplifying API documentation with the help of Swagger. It automatically generates interactive API documentation which makes it easier for the developers to understand and consume APIs.
14. Model Binding
Another great feature is the model binding in .NET Core. This feature of model binding helps in the automatic mapping of the data of HTTP requests to action method parameters. This helps in reducing boilerplate code.
15. Updates in SignalR
There have been improvements in the latest features of .NET Core as a Java client for SignalR has been added that allows connection to the server of SignalR from Java code. SignalR is a library for real-time web functionality.
Summary
Through this article, you discovered several top features of .NET Core. All of these features and services that .NET Core offers, together makes it up to be the best choice when choosing frameworks for web development. If you are a budding web developer and need guidance on frameworks like .NET, do consider enrolling in ASP.NET Core Certification Course to start your development journey the right way!.
FAQs
Q1. What are the features of .NET Core?
Q2. What is advantage of .NET Core?
Q3. What is special in .NET Core?
Q4. What is .NET and its features?
Take our free skill tests to evaluate your skill!
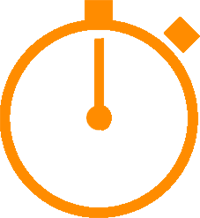
In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.