25
AprReact Core Concepts : State, Props, Virtual DOM, Events and Refs
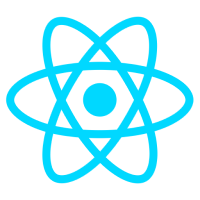
ReactJS Course
React Core Concepts: An Overview
The React library does have a certain core concept on which it is based, and by using those components, we can achieve the development activities with ease, out of them, some of the features are pretty important such as state, props and virtual DOM which makes React powerful amongst the other frameworks and libraries available in the market.
Now that we're done dealing with JSX and what role does it serves in React, it’s now the time to deal with the core concepts of React. So, in this section of React Tutorial, we’re going to discuss the following core concepts
State
Props
Virtual DOM
DOM vs. Virtual DOM
Events
Refs
At the end of the article, you should be able to grasp the knowledge of basic concepts that allow you to understand how React works using the above-given concepts combined.
For more understanding of React Concepts, consider enrolling in our React JS Certification Training
Read More - React JS Interview Questions And Answers
State
State in React is an object that determines how a component should be rendered or displayed. It is what makes your component dynamic as it renders/re-renders when you make changes to the state object during the manipulation from the component. React does the re-rendering under the hood when you’ve changed the state using setState(). The state can be said to be very similar to props, but it’s constrained within a component. Each component has their own state that determines what it should look like and put our logic into that state, each state values created for a different purpose.
Take note that a component “should” be a class rather than a functional component as we have two types of components we can use to render react components. Take a note the functional component now is no longer being used just for the rendering purpose, with the Release of React 16.8, it has react hooks function which allows us to use state and various life-cycle methods behavior inside the functional component, so in terms of feature, now both stateful and functional components are similar.
We will tackle what’s the difference between the two later on, but for now, let’s focus on the state. Let’s take a look at the use-case on how we use the state.
Code snippet
class App extends React.Component {
constructor(props) {
super(props);
this.state = {myVal: "Hello world! Click me..."};
}
render() {
return (
<div
className="App"
onClick={e =>
this.setState({
myVal:"You changed me"
})
}
>
<h1>{this.state.myVal}</h1>
</div>
);
}
}
Initial state

After setState

You can see that we used the state to hold and change the value of an object, and finally render it in our component. All of this is possible because of React’s algorithm under the hood where the component gets re-rendered once the existing state values have been manipulated inside the existing component.
Read More - React JS Roadmap 2024
Props
Props in React are properties that are present within a component. Just like how a class in OOP has properties representing its data structure, the same goes with React’s component. And the main point about the props is that it won't allow us to alter the existing props object from the component, rather, it can be used as a value for the rendering.
Just imagine a car’s property within your component. How would you make use of properties in React’s component? We do it like so:
<Car
brand={car.brand}
fuelType={car.fuelType}
gasLevels={car.gasLevels}
/>
We set the properties when we’re about to use a component to render in the page.
export default class Car extends React.Component {
constructor(props) {
super(props);
}
render() {
const {brand, fuelType, gasLevels} = this.props;
return (
<div>
<h1>{brand}</h1>
<div>Brand name: {brand}</div>
<div>Fuel: {fuelType}</div>
<div>Gas Levels: {gasLevels}</div>
)
}
}
Then, we are to access those properties inside a component by calling this.props and the multiple props can be de-structured so that all the required props can be consumed into the child component as required.
Virtual DOM
Now, let’s talk about Virtual DOM and how it shapes the react ecosystem so that it’s better, and well-optimized when rendering it on the webpage. Everything you see on the webpage is rendered in HTML format. And within that HTML, we have the so-called DOM or Document Object Model that allows us to display the buttons, align forms where they should be positioned, divide layouts, and display what a user needs to see. Virtual DOM is simply a stripped-down version of the real DOM to make the rendering more performant. Additionally, it’s using what we called the “diffing algorithm” to make the performance more impactful.
The virtual DOM is the core feature of React which makes it unique and powerful amongst the other libraries and frameworks as it has the capabilities to cater for the fastest performance and better user interactivity.
DOM vs. Virtual DOM
Virtual DOM has some shared similarities with the DOM. Except that Virtual DOM is lighter and faster to render than the real one. Virtual DOM is best paired with React because of diffing algorithm and it isn’t drawn onscreen compared to the real DOM.
The Virtual dom is more powerful than real DOM because it compares the existing and old DOM and compares against the actual changes, and if required to render it again, then the component will be re-rendered otherwise it won't do that unnecessarily.
Events
React does a good job of handling events that handles user interactions inside the web app. It’s one of my favorites and one of the reasons why I’m using react in my recent projects. Fortunately, how to react handles events are pretty similar to how you would do it in HTML DOM, so pretty much it will all be too familiar for you when you delve into it.
React supports all the synthetic events such as a mouse, keyboard, touch, and all other events supported by JavaScript but with some tweaks such as naming conventions.
Code snippet
<h1
onClick={(e) =>
this.setState({ myVal: "you clicked me" })
}
onBlur={(e) => console.log("hovered")}
>
{this.state.myval}
</h1>
You’ll notice that this is pretty similar to how you add an event handler to HTML DOM. I like how it has retained it and it equally feels like I’m using the plain old javascript and HTML DOM.
Refs
If you need more power and flexibility in accessing the particular DOM (such as getting the DOM’s position or getting its coordinates), then React provides an escape hatch for you to use and this is through the use of “Refs”. Refs are the best use when you wanted to get all the properties of the specific DOM within the component you’re trying to access. One use case for this is when you wanted to apply animations and you want to use all the properties the DOM has to fully implement the animation you’re trying to achieve. Here’s the sample code snippet for you to play around with if you wanted to use it (according to official docs)
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.myRef = React.createRef();
}
render() {
return <div ref={this.myRef} />;
}
}
Summary
React does have all the features such as state, props, synthetic events, refs which are capable of implementing full-fledged single-page applications and it also works with any server-side rendering libraries to cater to the best user experience and the faster software development.
So far we have discussed the core concepts of React and how we are going to use them, particularly in the context of React JS training, in order for us to grasp the React ecosystem. I hope you guys learned a lot from our article for today.
Take our free react skill challenge to evaluate your skill

In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.