Strings in JavaScript
Strings in JavaScript: An Overview
Strings in JavaScript are a sequence of characters. In this JavaScript tutorial, we'll discuss all the aspects of strings starting from declaration to various string methods. Consider enrolling in our JavaScript Programming Course to get a thorough practical understanding.
What is a String in JavaScript?
Strings represent text. A JavaScript string is a primitive data type used for storing texts. They are written inside quotes.
JavaScript String Example
const name = 'Welcome to ScholarHat';
Important Terminologies of a JavaScript String
- String: It is a sequence of characters enclosed in single (‘ ‘) or double (” “) quotes.
- Length: The number of characters in a string, obtained using the length property.
- Index: It indicates the position of a character within a string, starting from 0.
- Concatenation: The combination of two or more strings to create a new one.
- Substring: It is a portion of a string, obtained by extracting characters between specified indices.
Read More: JavaScript Interview Questions and Answers
Creation of JavaScript Strings/Declaration of a String in JavaScript
There are various methods to create or declare a string in JavaScript
1. Using Single Quotes
In this, you can create a string by enclosing it within single quotes.
Syntax
let str = 'String with single quote';
Example
let str = 'Welcome to ScholarHat';
console.log(str);
Output
Welcome to ScholarHat
2. Using Double Quotes
In this, enclose your text inside double quotes to declare a string.
Syntax
let str = 'String with double quotes';
Example
let str = "Welcome to ScholarHat";
console.log(str);
Output
Welcome to ScholarHat
Remember: Single quotes and double quotes are practically the same and you can use either of them.
3. String Constructor
Here, a new keyword is used to create an instance of string.
Syntax
let str=new String("string literal");
Example
let str = new String('Welcome to ScholarHat');
console.log(str);
Output
Welcome to ScholarHat
4. Using Template Literals (String Interpolation)
Templates are strings enclosed in backticks (`ScholarHat`). With template literals, you can embed expressions within backticks (`) for dynamic string creation. You can create multiline strings using this method.
Syntax
let str = 'Template Litral String';
let newStr = `String created using ${str}`;
or
let str = `
Multiline
String`;
Example
let str = 'Welcome to ScholarHat';
let newStr = `String created using ${str}`;
=
console.log(newStr);
Output
Welcome to ScholarHat
Example
let str = `
Welcome to
this JavaScript tutorial
of ScholarHat`;
console.log(str);
Output
Welcome to
this JavaScript tutorial
of ScholarHat
Fundamental Concepts of JavaScript Strings
- JavaScript Strings are immutable
The characters of a string cannot be changed in JavaScript once it is declared.
Example
let str = 'ScholarHat'; str[0] = 's'; console.log(str);
Output
ScholarHat
You can't modify the string once created but you can assign a new string to the same variable.
Example
let str = 'ScholarHat'; str = 'scholarhat'; console.log(str);
Output
scholarhat
- JavaScript is Case-Sensitive
In JavaScript, the lowercase and uppercase letters are treated as different values.
Example
const str = 's'; const str1 = 'S' console.log(str === str1);
Output
false
- String Objects and normal strings are different in JavaScript
In JavaScript, the string created by the new keyword is an object and is not the same as normal strings.
Example
const str1 = new String("Welcome to ScholarHat"); const str2 = "Welcome to ScholarHat"; console.log(typeof str1); console.log(typeof str2); console.log(str1 == str2); console.log(str1 === str2);
In the above code, as str1 is an object and str2 is a normal string, the "===" operator returns false.
Output
object string true false
Escape Characters
You can use the backslash escape character \ to include special characters in a string.
The following table describes the special characters you can use with \.
Code | Output |
\" | include double quote |
\\ | include backslash |
\n | new line |
\r | carriage return |
\v | vertical tab |
\t | horizontal tab |
\b | backspace |
\f | form feed |
String Manipulation
1. Access String Characters
This can be done in two ways:
- Using indexes
A string is an array of characters. Hence, we can access its characters using specific indexes.
Example
let str = 'ScholarHat'; console.log(str[2]);
Output
h
- Using charAt() method
Example
let str = 'ScholarHat'; console.log(str.charAt(2));
Output
h
2. String Concatenation
This can be done using the + Operator.
Example
let str1 = 'Welcome to';
let str2 = 'ScholarHat';
let result = str1 + str2;
console.log("Concatenated String: " + result);
Output
Concatenated String: Welcome to ScholarHat
3. String Comparison in JavaScript
This can be done in two ways:
- Using equality operator
It returns the result in boolean values.
Example
let str1 = "ScholarHat"; let str2 = new String("ScholarHat"); console.log(str1 == str2);
Output
true
- Using localeCompare() method
It returns the result in ASCII values.
Example
let str1 = "ScholarHat"; let str2 = new String("ScholarHat"); console.log(str1.localeCompare(str2));
Output
0
4. JavaScript String Length
This is done using the built-in length property.
Example
let str = 'ScholarHat';
let len = str.length;
console.log("String Length: " + len);
Output
String Length: 10
JavaScript String Methods
The table below describes the commonly used JavaScript String methods
Method | Description |
charAt(index) | returns the character at the specified index |
concat() | joins two or more strings |
replace() | replaces a string with another string |
split() | converts the string to an array of strings |
substr(start, length) | returns a part of a string |
substring(start,end) | returns a part of a string |
slice(start, end) | returns a part of a string |
toLowerCase() | returns the passed string in lower case |
toUpperCase() | returns the passed string in the upper case |
trim() | removes whitespace from the strings |
includes() | searches for a string and returns a boolean value |
search() | searches for a string and returns a position of a match |
trimStart() | removes whitespace from the beginning of a string |
trimEnd() | removes white space from the end of a string |
padStart() | pad a string with another string until it reaches the given length |
padEnd() | pad a string with another string until it reaches the given length |
valueOf() | Return the value of the given string |
match() | Search a string for a match against any regular expression |
indexOf() | Finds the index of the first occurrence of the argument string in the given string |
Example to Demonstrate the Working of JavaScript String Methods
let text1 = 'Welcome to';
let text2 = 'ScholarHat';
let text3 = ' JavaScript ';
// concatenating two strings
const result1 = text1.concat(' ', text2);
console.log(result1);
// converting the text to uppercase
const result2 = text1.toUpperCase();
console.log(result2);
// converting the text to uppercase
const lwres = text1.toLowerCase();
console.log(lwres);
// gives the substring of a string
let part = text1.substring(6, 10);
console.log(part);
// removing whitespace from the string
const result3 = text3.trim();
console.log(result3);
//replaces a part of the given string
let rep = text2.replace("ScholarHat", "DotNetTricks");
console.log(rep)
// converting the string to an array
const result4 = text1.split();
console.log(result4);
// slicing the string
const result5= text1.slice(1, 3);
console.log(result5);
Output
Welcome to ScholarHat
WELCOME TO
welcome to
e to
JavaScript
DotNetTricks
[ 'Welcome to' ]
el
JavaScript String() Function
The String() function in JavaScript converts various data types to strings.
Example of String() Function in JavaScript
const a = 125; // number
const b = true; // boolean
//converting to string
const result1 = String(a);
const result2 = String(b);
console.log(result1);
console.log(result2);
In the above program, the String() function converts the number and boolean data type variables into strings.
Output
125
true
Summary
In the above blog, we saw with illustrations a complete picture of Strings in JavaScript. You won't face any difficulty while going through the article. For a practical understanding, consider our JavaScript Programming Course.
Take our free skill tests to evaluate your skill!
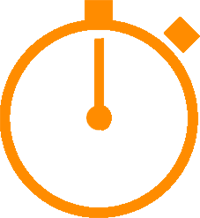
In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.