21
meiData Types in Python - 8 Data Types in Python With Examples
Data Types in Python: An Overview
A data type in Python is a classification of specific types of data by a certain value or certain types of mathematical or logical operations. In this comprehensive Python Tutorial article, we’ll explore the Data types in Python with examples explaining what each one is used for and how to know where which one is suitable. Whether you're new to coding or an experienced programmer looking for Python training, this article has something for everyone!
What is a Data Type in Python?
The way that data items are categorized or classified is known as their data type. It stands for the type of value that indicates the types of operations that can be carried out on a specific set of data. In this programming, python variables are instances (objects) of these classes, and data types are truly classes because everything is an object.
Read More - 50 Python Interview Questions
Types of Data Types in Python
Python has various built-in data types which will be discussed in this article:
- Numeric - int, float, complex
- String - str
- Sequence - list, tuple, range
- Binary - bytes, bytearray, memoryview
- Mapping - dict
- Boolean - bool
- Set - set, frozenset
- None - NoneType
1. Numeric data types in Python
- In Python, data with a numeric value is represented by the numeric data type.
- Python programming language supports four different numerical types −
- int (signed integers)
- long (long integers, they can also be represented by octal and hexadecimal)
- float (floating point real values)
- complex (complex numbers)
int | long | float | complex |
10 | 51924361L | 0.0 | 3.14j |
100 | -0x19323L | 15.20 | 45.j |
-786 | 0122L | -21.9 | 9.322e-36j |
080 | 0xDEFABCECBDAECBFBAEl | 32.3+e18 | .876j |
-0490 | 535633629843L | -90. | -.6545+0J |
-0x260 | -052318172735L | -32.54e100 | 3e+26J |
0x69 | -4721885298529L | 70.2-E12 | 4.53e-7j |
Example of Numeric Data Type in Python Compiler
# integer variable.
a=150
print("The type of variable having value", a, " is ", type(a))
# float variable.
b=20.846
print("The type of variable having value", b, " is ", type(b))
# complex variable.
c=18+3j
print("The type of variable having value", c, " is ", type(c))
In this code, three variables (a, b, and c) are defined with three different data types (integer, float, and complex), and each variable's type and value are printed.
Output
The type of variable having value 150 is <class 'int'>
The type of variable having value 20.846 is <class 'float'>
The type of variable having value (18+3j) is <class 'complex'>
Read More - Python Developer Salary in India
2. Python String Data Type
- A group of one or more characters enclosed in a single, double, or triple quote is called a string in python.
- A character in Python is just a string with a length of one; there is no character data type.
- The str class is used to represent it.
Example of String Data Type in Python
str = 'Hello World!'
print (str) # Prints complete string
print (str[0]) # Prints first character of the string
print (str[2:5]) # Prints characters starting from 3rd to 5th
print (str[2:]) # Prints string stating from 3rd character
print (str * 2) # Prints string two times
print (str + "TEST") # Prints concatenated string
With the help of this code, you can see how to do many string operations in Python, such as printing the complete string, accessing particular characters, slicing the string to get a substring, repeating the text, and concatenating it with another string.
Output
Hello World!
H
llo
llo World!
Hello World!Hello World!
Hello World!TEST
3. Python List Data Type
- Python list is an arranged grouping of the same or dissimilar elements that are enclosed by brackets [] and separated by commas.
- Use the index number to retrieve the list entries.
- To access a particular item in a list, use the index operator [].
Example of List Data Type in Python
list = [ 'abcd', 786 , 2.23, 'Scholar-Hat', 70.2 ]
tinylist = [123, 'Scholar-Hat']
print (list) # Prints complete list
print (list[0]) # Prints first element of the list
print (list[1:3]) # Prints elements starting from 2nd till 3rd
print (list[2:]) # Prints elements starting from 3rd element
print (tinylist * 2) # Prints list two times
print (list + tinylist) # Prints concatenated lists
Working with Python lists is shown by this code in the Python Editor. It introduces two lists, "list" and "tiny list," and it illustrates several list actions, such as printing the complete list, accessing specific components, slicing to extract a sublist, repeating a list, and concatenating two lists.
Output
['abcd', 786, 2.23, 'Scholar-Hat', 70.2]
abcd
[786, 2.23]
[2.23, ‘Scholar-Hat', 70.2]
[123, 'Scholar-Hat', 123, 'john']
['abcd', 786, 2.23, 'Scholar-Hat', 70.2, 123, 'Scholar-Hat']
4. Python Tuple Data Type
- A tuple in Python is an ordered list of elements, just like a list.
- Tuples are not changeable, which is the only difference.
- Once created, tuples cannot be changed.
- In Python, items of a tuple are stored using parentheses ().
- In Python, we utilize the index number to retrieve tuple items, just like with lists.
Example of Tuple Data Type in Python
tuple = ( 'abcd', 786 , 2.23, 'Scholar-Hat', 70.2 )
tinytuple = (123, 'Scholar-Hat')
print (tuple) # Prints the complete tuple
print (tuple[0]) # Prints first element of the tuple
print (tuple[1:3]) # Prints elements of the tuple starting from 2nd till 3rd
print (tuple[2:]) # Prints elements of the tuple starting from 3rd element
print (tinytuple * 2) # Prints the contents of the tuple twice
print (tuple + tinytuple) # Prints concatenated tuples
Python tuples are used in this code to show how they work. It introduces two tuples, "tuple" and "tinytuple," defines them, and illustrates a number of tuple operations, such as publishing the complete tuple, accessing each member, slicing to extract a sub-tuple, repeating a tuple, and concatenating two tuples.
Output
('abcd', 786, 2.23, 'Scholar-Hat', 70.2)
abcd
(786, 2.23)
(2.23, 'Scholar-Hat', 70.2)
(123, ‘Scholar-Hat', 123, 'Scholar-Hat')
('abcd', 786, 2.23, 'Scholar-Hat', 70.2, 123, 'Scholar-Hat')
5. Python Range Data Type
- Range() in Python is a built-in function that returns a series of numbers that begin at 0 and increase by 1 until they reach a predetermined number.
- Utilizing a for and while loop in python, we use the range() method to produce a series of numbers.
Example of Range Data type in Python
for i in range(1, 5):
print(i)
This program iterates over numbers from 1 to 4 (inclusive) and writes each one on a separate line using a for loop in python.
Output
1
2
3
4
6. Python Dictionary Data Type
- Python's dictionary is an unordered collection of data values that is used to store data values in a map-like fashion.
- Unlike other data types, which only include a single value per element, dictionaries contain a key-value pair.
- The dictionary contains key-value pairs to make it more effective.
- In a dictionary, a colon (:) separates each key-value pair, while a comma separates each key.
- A dictionary in Python can be made by enclosing a list of elements in curly {} braces and separating them with commas.
Example of Dictionary Data Type in Python
dict = {}
dict['one'] = "This is one"
dict[2] = "This is two"
tinydict = {'name': 'Scholar-Hat','code':6734, 'dept': 'sales'}
print (dict['one']) # Prints value for 'one' key
print (dict[2]) # Prints value for 2 key
print (tinydict) # Prints complete dictionary
print (tinydict.keys()) # Prints all the keys
print (tinydict.values()) # Prints all the values
The use of dictionaries in Python is shown by the following code. It performs several activities, such as accessing values by keys, printing the complete dictionary, and showing keys and values individually for 'tinydict', and defines three dictionaries: 'dict', and 'tinydict'.
Output
This is one
This is two
{'name': 'Scholar-Hat', 'code': 6734, 'dept': 'sales'}
dict_keys(['name', 'code', 'dept'])
dict_values(['Scholar-Hat', 6734, 'sales'])
7. Python Boolean Data Type
- One of the built-in data types in Python is the boolean type, which can represent either True or False.
- Any expression can be evaluated for value using the Python bool() method, which returns True or False depending on the expression.
Example of Boolean Data Type in Python
a = True
# display the value of a
print(a)
# display the data type of a
print(type(a))
The variable 'a' is given the Boolean value "True" by the following code, which then prints both the value ("True") and the data type ("bool") of the variable.
Output
true
<class 'bool'>
8. Python Set Data Type
- A set is an unsorted collection of distinct components. This means that set elements cannot be repeated, and the order in which they are kept is irrelevant.
- Sets are changeable, which means that after they are constructed, their elements can be added or withdrawn. A set's elements, on the other hand, cannot be modified in place.
- Curly brackets {}are used to define sets.
Example of Set Data Type in Python
my_set = {1, 2, 3, 4, 5}
print(my_set)
This code generates the set my_set and assigns the numbers 1, 2, 3, 4, and 5 to it. The contents of the set are then printed to the console. Output
{1, 2, 3, 4, 5}
What is the data type conversion function?
- In Python, the process of converting an object's data type from one type to another is referred to as data type conversion.
- In Python, there are two primary methods for converting data types:
- Implicit Type Conversion
- Explicit Type Conversion
Example of Data Type Conversion Function in Python Online Compiler
a = str(1) # a will be "1"
b = str(2.2) # b will be "2.2"
c = str("3.3") # c will be "3.3"
print (a)
print (b)
print (c)
In this code, the numbers 1 and 2.2 are converted to string representations and assigned to variables 'a' and 'b', respectively. The string "3.3" is already present in variable "c". Following that, it prints the values of "a," "b," and "c," producing the output.
Output
1
2.2
3.3
How to check Data Type in Python?
These steps can be used to determine the data type of a variable or value in Python:
- Give a variable a value or choose the value whose data type you want to examine.
- To ascertain the variable's or value's data type, use the type() function.
- To see the data type, print the outcome.
FAQs
1. How can I perform mathematical operations with numeric data types?
For mathematical operations in Python with numeric data types, use operators like +, -, *, and /.
2. When should I use complex numbers in Python?
When calculating with both real and fictional components, use complex numbers.
3. How can I manipulate and format strings?
Use tools like str.format() or f-strings to manipulate and format strings.
4. What are some common string operations?
Slicing, concatenating, and searching with tools like find() and split() are common string operations.
5. How can I add or remove elements from a Python list?
Lists can be modified by adding with append() or extend(), removing with remove() or pop(), or using list comprehensions.
6. How can I check if an element exists in a Python List?
Use the "in" keyword or the index() method on lists to verify an element's presence.
7. What are sequence types in Python?
Lists, tuples, and strings are examples of sequence types.
8. How do lists and tuples differ?
Python Tuples are immutable (unchangeable), whereas lists are mutable (may be changed).
9. When should I use the range data type?
If you want to loop through a list of numbers, use the range data type.
10. How can I convert a Python Range into a list or other iterable?
Use list(range()) to turn a Python Range into a list or a for loop to iterate directly.
11. How many data types are there in Python?
There are numerous data types available in Python, including int, float, str, bool, list, tuple, dict, set, and others.
12. What is the difference between mutable and immutable data types in Python, and why does it matter?
Lists are an example of a mutable data type, while tuples are an example of an immutable type.
13. What are the common pitfalls or issues related to data types in Python, and how can I avoid them?
Random type conversions, changeable default parameters, and data loss during type conversions are common hazards. To prevent these problems, use explicit type handling.
14. What is a data type in Python?
The kind of data an object can contain, such as numbers, texts, or lists, is defined by its data type in Python.
Summary
Python has five standard data types − integers, floating point numbers, strings, lists, and dictionaries. In addition, Python also provides some special data types such as files, frozen sets, and a few more. This was all about the basics of data types in Python, if you want to go deeper into data types then consider enrolling in a Python Certification Course.
Take our free skill tests to evaluate your skill!
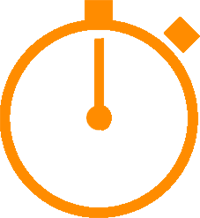
In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.