25
apr.Understanding jQuery Mobile Events
jQuery mobile is made on the top of jQuery Library and jQuery offers various events for handling user interaction. In addition, jQuery Mobile also offers various native events that are especially made for mobile devices. The jQuery mobile events can be categorized into four categories as given below:
Touch Events
These events triggers when you touch the screen. These events also work with your PC when you do tap and swipe with your mouse. Touch events example are: tap, taphold, swipe, swipeleft and swiperight.
<!DOCTYPE html> <html> <head> <title>jQuery Mobile</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="http://code.jquery.com/mobile/1.3.2/jquery.mobile-1.3.2.min.css" /> <script src="http://code.jquery.com/jquery-1.10.2.min.js"></script> <script src="http://code.jquery.com/mobile/1.3.2/jquery.mobile-1.3.2.min.js"></script> <script> $(document).ready(function () { $("p").on("tap", function () { alert("Tap event detected!"); }); $("p").on("taphold", function () { alert("Tap hold event detected!"); }); $("p").on("swipe", function () { alert("Swipe event detected!"); }); $("p").on("swipeleft", function () { alert("Swipe left event detected!"); }); $("p").on("swiperight", function () { alert("Swipe right event detected!"); }); }); </script> </head> <body> <div data-role="page"> <div data-role="header"> <div align="center">jQuery Mobile Events</div> </div> <div data-role="content"> <p>Introduction to jQuery Mobile Events!</p> </div> </div> </body> </html>
Scroll Events
These events triggers when you scroll up and down the screen. Scroll events examples are: scrollstart and scrollstop.
<!DOCTYPE html> <html> <head> <title>jQuery Mobile</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="http://code.jquery.com/mobile/1.3.2/jquery.mobile-1.3.2.min.css" /> <script src="http://code.jquery.com/jquery-1.10.2.min.js"></script> <script src="http://code.jquery.com/mobile/1.3.2/jquery.mobile-1.3.2.min.js"></script> <script> $(document).ready(function () { $("p").on("scrollstart", function () { alert("Scrolling start event detected!"); }); $("p").on("scrollstop", function () { alert("Scrolling stop event detected!"); }); }); </script> </head> <body> <div data-role="page"> <div data-role="header"> <div align="center">jQuery Mobile Events</div> </div> <div data-role="content"> <p> Introduction to jQuery Mobile Events! <br /><br />Introduction to jQuery Mobile Events! <br /><br />Introduction to jQuery Mobile Events! <br /><br />Introduction to jQuery Mobile Events! <br /><br />Introduction to jQuery Mobile Events! <br /><br />Introduction to jQuery Mobile Events! <br /><br />Introduction to jQuery Mobile Events! <br /><br />Introduction to jQuery Mobile Events! <br /><br />Introduction to jQuery Mobile Events! <br /><br />Introduction to jQuery Mobile Events! <br /><br />Introduction to jQuery Mobile Events! <br /><br />Introduction to jQuery Mobile Events! </p> </div> </div> </body> </html>
Page Events
These events triggers when a page is loaded or unloaded, shown or hidden and created. Page events examples are: pagecreate, pageinit, pageload, pageshow, pagehide.
<!DOCTYPE html> <html> <head> <title>jQuery Mobile</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="http://code.jquery.com/mobile/1.3.2/jquery.mobile-1.3.2.min.css" /> <script src="http://code.jquery.com/jquery-1.10.2.min.js"></script> <script src="http://code.jquery.com/mobile/1.3.2/jquery.mobile-1.3.2.min.js"></script> <script> $(document).on("pagecreate", function (event) { alert("Page create event detected!"); }); $(document).on("pageinit", function (event) { alert("Page init event detected!") }); $(document).on("pageshow", "#page2", function () { //When entering nextpage alert("page2 is now shown!"); }); $(document).on("pagehide", "#page2", function () {// When leaving nextpage alert("page2 is now hidden!"); }); </script> </head> <body> <div data-role="page" id="page1"> <div data-role="header"> <div align="center">jQuery Mobile Events</div> </div> <div data-role="content"> <h1>Page1</h1> <p> Introduction to jQuery Mobile Events! </p> <a href="#page2">Go to Next Page</a> </div> </div> <div data-role="page" id="page2"> <div data-role="header"> <div align="center">jQuery Mobile Events</div> </div> <div data-role="content"> <h1>Page2</h1> <p> Introduction to jQuery Mobile Events! </p> <a href="#page1">Go to Previous Page</a> </div> </div> </body> </html>
Orientation Events
These events triggers when you rotates mobile device vertically or horizontally i.e. change your mobile device orientation. Orientation events examples are: orientationchange.
<!DOCTYPE html> <html> <head> <title>jQuery Mobile</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="http://code.jquery.com/mobile/1.3.2/jquery.mobile-1.3.2.min.css" /> <script src="http://code.jquery.com/jquery-1.10.2.min.js"></script> <script src="http://code.jquery.com/mobile/1.3.2/jquery.mobile-1.3.2.min.js"></script> <script> $(document).ready(function () { $(window).on("orientationchange", function (event) { alert("Orientation is: " + event.orientation); }); }); </script> </head> <body> <div data-role="page"> <div data-role="header"> <div align="center">jQuery Mobile Events</div> </div> <div data-role="content"> <span></span> <p> Introduction to jQuery Mobile Events! </p> </div> </div> </body> </html>
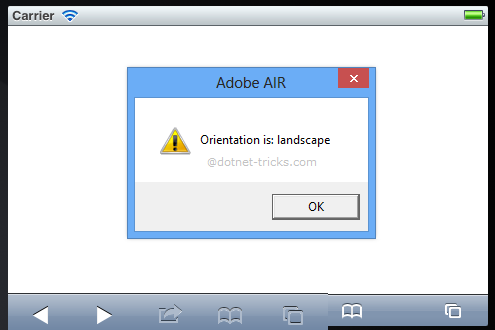
What do you think?
I hope you have enjoyed the article and would able to have a clear picture about jQuery Mobile events. I would like to have feedback from my blog readers. Your valuable feedback, question, or comments about this article are always welcome.
Take our free skill tests to evaluate your skill!
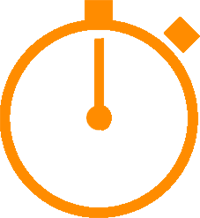
In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.