26
apr.Understanding AngularJS Templates
In Angular, templates are the views with the HTML enriched by Angular elements like directive and attributes. Templates are used to display the information from the model and controller that a user sees in his browser. An angular templates can have Directive, HTML markup, CSS, Filters, Expressions and Form controls. For a comprehensive understanding of Angular's templates and their integration with controllers and models, consider exploring an Angular certification Training program.
A simple Angular Template
<html ng-app> <body ng-controller="MyController"> <input ng-model="name" value="dotnet-tricks.com"> <br/> Input Value is : {{name}} <button ng-click="changeValue()">Click me!</button> <script src="angular.js"> </body> </html>
Types of Templates
There are two types of templates :
Static Templates
A static template is defined by using script tag. It must has an
id
attribute with a unique value and atype
attribute with valuetext/ng-template
. Also, a static template must be inside the scope of the ng-app directive otherwise it will be ignored by Angular.<script type="text/ng-template" id="person.html"> {{person.name}} : {{person.address}} </script>
A static template can be rendered by using ng-include directive.
<div ng-include="'person.html'"></div>
Full Example of Static Template
<!DOCTYPE html> <html> <head> <title>AngularJS Static Templates</title> <script src="lib/angular.js"></script> <script> //defining module var app = angular.module('app', []); app.controller("myController", function ($scope) { $scope.person = { name: "Deepak Chauhan", address: "Delhi" }; }); app.controller("homeController", function ($scope) { $scope.persons = [{ name: "Deepak Chauhan", address: "Delhi" }, { name: "Shailendra Chauhan", address: "Noida" }, { name: "Kuldeep Chauhan", address: "Gurgaon" }] }); </script> </head> <body ng-app="app"> <h1>AngularJS : Static Templates</h1> <!--It should be the part of ng-app directive--> <script type="text/ng-template" id="person.html"> {{person.name}} : {{person.address}} </script> <div ng-controller="myController"> <h1>myController</h1> <!--Please not the single quotes around person.html. The value of the ng-include is an expression and person.html is a string value, so put single quotes around it.--> <div ng-include="'person.html'"></div> </div> <div ng-controller="homeController"> <h1>homeController</h1> <div ng-repeat="person in persons" ng-include="'person.html'"></div> </div> </body> </html>
Dynamic Templates
A dynamic template is an html page which is compiled and rendered by Angular on demand. The above static template can be created as a HTML page within templates folder of your app like as:
person.html
{{person.name}} : {{person.address}}
A dynamic template can be rendered by using ng-include directive.
<div ng-include="'templates/person.html'"></div>
Full Example of Dynamic Template
<!DOCTYPE html> <html> <head> <title>AngularJS Dynamic Templates</title> <script src="lib/angular.js"></script> <script> //defining module var app = angular.module('app', []); app.controller("myController", function ($scope) { $scope.person = { name: "Deepak Chauhan", address: "Delhi" }; }); app.controller("homeController", function ($scope) { $scope.persons = [{ name: "Deepak Chauhan", address: "Delhi" }, { name: "Shailendra Chauhan", address: "Noida" }, { name: "Kuldeep Chauhan", address: "Gurgaon" }] }); </script> </head> <body ng-app="app"> <h1>AngularJS : Dynamic Templates</h1> <div ng-controller="myController"> <h1>myController</h1> <!--Please not the single quotes around person.html. The value of the ng-include is an expression and person.html is a string value, so put single quotes around it.--> <div ng-include="'templates/person.html'"></div> </div> <div ng-controller="homeController"> <h1>homeController</h1> <div ng-repeat="person in persons" ng-include="'templates/person.html'"></div> </div> </body> </html>
person.html
{{person.name}} : {{person.address}}
Note
Always put the single quotes around the name of template with ng-include directive, since it accept an expression. Hence to indicate to Angular that template (person.html) is a string value you have to put single quotes around it's name.
What do you think?
I hope you will enjoy the AngularJS Templates while developing your app with AngularJS. I would like to have feedback from my blog readers. Your valuable feedback, question, or comments about this article are always welcome. For those looking to gain a deeper understanding of AngularJS templates and their application in building robust applications, consider exploring an Angular Certification program. Your journey towards mastering Angular begins here!
Take our free skill tests to evaluate your skill!
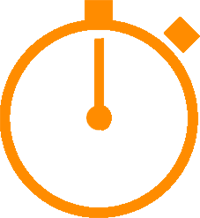
In less than 5 minutes, with our skill test, you can identify your knowledge gaps and strengths.